a library to use GPRS like ethernet or wifi, which makes it possible to connect to the internet with your GPRS module
Dependents: Seeed_GPRS_Library_HelloWorld Seeed_GPRS_Xively_HelloWorld Seeed_ARCH_GPRS_V2_Xively_HelloWorld Seeed_ARCH_GPRS_V2_ThingSpeak_HelloWorld ... more
GPRS.cpp
00001 /* 00002 GPRS.cpp 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-2-24 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #include "mbed.h" 00024 #include "GPRS.h" 00025 00026 GPRS* GPRS::inst; 00027 00028 GPRS::GPRS(PinName tx, PinName rx, int baudRate, const char* apn, const char* userName, const char* passWord) : Modem(tx,rx,baudRate) 00029 { 00030 inst = this; 00031 _apn = apn; 00032 _userName = userName; 00033 _passWord = passWord; 00034 socketID = -1; 00035 } 00036 00037 bool GPRS::preInit() 00038 { 00039 for(int i = 0; i < 2; i++) { 00040 sendCmd("AT\r\n"); 00041 wait(1); 00042 } 00043 return checkSIMStatus(); 00044 } 00045 00046 bool GPRS::checkSIMStatus(void) 00047 { 00048 char gprsBuffer[32]; 00049 int count = 0; 00050 cleanBuffer(gprsBuffer,32); 00051 while(count < 3) { 00052 sendCmd("AT+CPIN?\r\n"); 00053 readBuffer(gprsBuffer,32,DEFAULT_TIMEOUT); 00054 if((NULL != strstr(gprsBuffer,"+CPIN: READY"))) { 00055 break; 00056 } 00057 count++; 00058 wait(1); 00059 } 00060 if(count == 3) { 00061 return false; 00062 } 00063 return true; 00064 } 00065 00066 bool GPRS::join() 00067 { 00068 char cmd[64]; 00069 char ipAddr[32]; 00070 //Select multiple connection 00071 sendCmdAndWaitForResp("AT+CIPMUX=1\r\n","OK",DEFAULT_TIMEOUT,CMD); 00072 00073 //set APN 00074 snprintf(cmd,sizeof(cmd),"AT+CSTT=\"%s\",\"%s\",\"%s\"\r\n",_apn,_userName,_passWord); 00075 sendCmdAndWaitForResp(cmd, "OK", DEFAULT_TIMEOUT,CMD); 00076 00077 //Brings up wireless connection 00078 sendCmdAndWaitForResp("AT+CIICR\r\n","OK",DEFAULT_TIMEOUT,CMD); 00079 00080 //Get local IP address 00081 sendCmd("AT+CIFSR\r\n"); 00082 readBuffer(ipAddr,32,2); 00083 00084 if(NULL != strstr(ipAddr,"AT+CIFSR")) { 00085 _ip = str_to_ip(ipAddr+12); 00086 if(_ip != 0) { 00087 return true; 00088 } 00089 } 00090 return false; 00091 } 00092 00093 bool GPRS::setProtocol(int socket, Protocol p) 00094 { 00095 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00096 return false; 00097 } 00098 //ToDo: setProtocol 00099 return true; 00100 } 00101 00102 bool GPRS::connect(int socket, Protocol ptl,const char * host, int port, int timeout) 00103 { 00104 char cmd[64]; 00105 char resp[96]; 00106 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00107 return false; 00108 } 00109 if(ptl == TCP) { 00110 sprintf(cmd, "AT+CIPSTART=%d,\"TCP\",\"%s\",%d\r\n",socket, host, port); 00111 } else if(ptl == UDP) { 00112 sprintf(cmd, "AT+CIPSTART=%d,\"UDP\",\"%s\",%d\r\n",socket, host, port); 00113 } else { 00114 return false; 00115 } 00116 sendCmd(cmd); 00117 readBuffer(resp,96,2*DEFAULT_TIMEOUT); 00118 if(NULL != strstr(resp,"CONNECT")) { //ALREADY CONNECT or CONNECT OK 00119 return true; 00120 } 00121 return false;//ERROR 00122 } 00123 00124 bool GPRS::gethostbyname(const char* host, uint32_t* ip) 00125 { 00126 uint32_t addr = str_to_ip(host); 00127 char buf[17]; 00128 snprintf(buf, sizeof(buf), "%d.%d.%d.%d", (addr>>24)&0xff, (addr>>16)&0xff, (addr>>8)&0xff, addr&0xff); 00129 if (strcmp(buf, host) == 0) { 00130 *ip = addr; 00131 return true; 00132 } 00133 return false; 00134 } 00135 00136 bool GPRS::disconnect() 00137 { 00138 sendCmd("AT+CIPSHUT\r\n"); 00139 return true; 00140 } 00141 00142 bool GPRS::is_connected(int socket) 00143 { 00144 char cmd[16]; 00145 char resp[96]; 00146 snprintf(cmd,16,"AT+CIPSTATUS=%d\r\n",socket); 00147 sendCmd(cmd); 00148 readBuffer(resp,sizeof(resp),DEFAULT_TIMEOUT); 00149 if(NULL != strstr(resp,"CONNECTED")) { 00150 //+CIPSTATUS: 1,0,"TCP","216.52.233.120","80","CONNECTED" 00151 return true; 00152 } else { 00153 //+CIPSTATUS: 1,0,"TCP","216.52.233.120","80","CLOSED" 00154 //+CIPSTATUS: 0,,"","","","INITIAL" 00155 return false; 00156 } 00157 } 00158 00159 void GPRS::reset() 00160 { 00161 00162 } 00163 00164 bool GPRS::close(int socket) 00165 { 00166 char cmd[16]; 00167 char resp[16]; 00168 00169 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00170 return false; 00171 } 00172 // if not connected, return 00173 if (is_connected(socket) == false) { 00174 return true; 00175 } 00176 snprintf(cmd, sizeof(cmd),"AT+CIPCLOSE=%d\r\n",socket); 00177 snprintf(resp,sizeof(resp),"%d, CLOSE OK",socket); 00178 if(0 != sendCmdAndWaitForResp(cmd, resp, DEFAULT_TIMEOUT,CMD)) { 00179 return false; 00180 } 00181 return true; 00182 } 00183 00184 bool GPRS::readable(void) 00185 { 00186 return readable(); 00187 } 00188 00189 int GPRS::wait_readable(int socket, int wait_time) 00190 { 00191 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00192 return -1; 00193 } 00194 char resp[16]; 00195 snprintf(resp,sizeof(resp),"\r\n\r\n+RECEIVE,%d",socket);//"+RECEIVE:<socketID>,<length>" 00196 int len = strlen(resp); 00197 00198 if(false == respCmp(resp,len,wait_time)) { 00199 return -1; 00200 } 00201 char c = readByte();//',' 00202 char dataLen[4]; 00203 int i = 0; 00204 c = readByte(); 00205 while((c >= '0') && (c <= '9')) { 00206 dataLen[i++] = c; 00207 c = readByte(); 00208 } 00209 c = readByte();//'\n' 00210 len = atoi(dataLen); 00211 return len; 00212 } 00213 00214 int GPRS::wait_writeable(int socket, int req_size) 00215 { 00216 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00217 return -1; 00218 } 00219 return req_size>256?256:req_size+1; 00220 } 00221 00222 int GPRS::send(int socket, const char * str, int len) 00223 { 00224 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00225 return -1; 00226 } 00227 char cmd[32]; 00228 wait(1); 00229 if(len > 0){ 00230 snprintf(cmd,sizeof(cmd),"AT+CIPSEND=%d\r\n",socket); 00231 if(0 != sendCmdAndWaitForResp(cmd,">",2,CMD)) { 00232 return false; 00233 } 00234 sendCmd(str); 00235 serialModem.putc((char)0x1a); 00236 } 00237 return len; 00238 } 00239 00240 int GPRS::recv(int socket, char* buf, int len) 00241 { 00242 if (socket < 0 || socket > MAX_SOCK_NUM-1) { 00243 return -1; 00244 } 00245 cleanBuffer(buf,len); 00246 readBuffer(buf,len,DEFAULT_TIMEOUT/2); 00247 return len; 00248 //return strlen(buf); 00249 } 00250 00251 int GPRS::new_socket() 00252 { 00253 socketID = 0; //we only support one socket. 00254 return socketID; 00255 } 00256 00257 uint16_t GPRS::new_port() 00258 { 00259 uint16_t port = rand(); 00260 port |= 49152; 00261 return port; 00262 } 00263 00264 uint32_t GPRS::str_to_ip(const char* str) 00265 { 00266 uint32_t ip = 0; 00267 char* p = (char*)str; 00268 for(int i = 0; i < 4; i++) { 00269 ip |= atoi(p); 00270 p = strchr(p, '.'); 00271 if (p == NULL) { 00272 break; 00273 } 00274 ip <<= 8; 00275 p++; 00276 } 00277 return ip; 00278 }
Generated on Thu Jul 14 2022 07:16:02 by
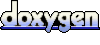