a library to use GPRS like ethernet or wifi, which makes it possible to connect to the internet with your GPRS module
Dependents: Seeed_GPRS_Library_HelloWorld Seeed_GPRS_Xively_HelloWorld Seeed_ARCH_GPRS_V2_Xively_HelloWorld Seeed_ARCH_GPRS_V2_ThingSpeak_HelloWorld ... more
modem.h
00001 /* 00002 modem.h 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-2-24 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #ifndef __MODEM_H__ 00024 #define __MODEM_H__ 00025 00026 #include "mbed.h" 00027 00028 #define DEFAULT_TIMEOUT 5 00029 00030 enum DataType { 00031 CMD = 0, 00032 DATA = 1, 00033 }; 00034 00035 /** Modem class. 00036 * Used for Modem communication. attention that Modem module communicate with MCU in serial protocol 00037 */ 00038 class Modem 00039 { 00040 00041 public: 00042 /** Create Modem Instance 00043 * @param tx uart transmit pin to communicate with Modem 00044 * @param rx uart receive pin to communicate with Modem 00045 * @param baudRate baud rate of uart communication 00046 */ 00047 Modem(PinName tx, PinName rx, int baudRate) : serialModem(tx, rx) { 00048 serialModem.baud(baudRate); 00049 }; 00050 00051 Serial serialModem; 00052 protected: 00053 /** Power on Modem 00054 */ 00055 void preInit(void); 00056 00057 /** check serialModem is readable or not 00058 * @returns 00059 * true on readable 00060 * false on not readable 00061 */ 00062 bool readable(); 00063 00064 /** read one byte from serialModem 00065 * @returns 00066 * one byte read from serialModem 00067 */ 00068 char readByte(void); 00069 00070 /** read from Modem module and save to buffer array 00071 * @param buffer buffer array to save what read from Modem module 00072 * @param count the maximal bytes number read from Modem module 00073 * @param timeOut time to wait for reading from Modem module 00074 * @returns 00075 * 0 on success 00076 * -1 on error 00077 */ 00078 int readBuffer(char* buffer,int count, unsigned int timeOut); 00079 00080 00081 /** clean Buffer 00082 * @param buffer buffer to clean 00083 * @param count number of bytes to clean 00084 */ 00085 void cleanBuffer(char* buffer, int count); 00086 00087 /** send AT command to Modem module 00088 * @param cmd command array which will be send to GPRS module 00089 */ 00090 void sendCmd(const char* cmd); 00091 00092 /**send "AT" to Modem module 00093 */ 00094 void sendATTest(void); 00095 00096 /** compare the response from GPRS module with a string 00097 * @param resp buffer to be compared 00098 * @param len length that will be compared 00099 * @param timeout waiting seconds till timeout 00100 */ 00101 bool respCmp(const char *resp, unsigned int len, unsigned int timeout); 00102 00103 /** check Modem module response before time out 00104 * @param *resp correct response which Modem module will return 00105 * @param *timeout waiting seconds till timeout 00106 * @returns 00107 * 0 on success 00108 * -1 on error 00109 */ 00110 int waitForResp(const char *resp, unsigned int timeout,DataType type); 00111 00112 /** send AT command to GPRS module and wait for correct response 00113 * @param *cmd AT command which will be send to GPRS module 00114 * @param *resp correct response which GPRS module will return 00115 * @param *timeout waiting seconds till timeout 00116 * @returns 00117 * 0 on success 00118 * -1 on error 00119 */ 00120 int sendCmdAndWaitForResp(const char* data, const char *resp, unsigned timeout,DataType type); 00121 00122 Timer timeCnt; 00123 00124 private: 00125 00126 }; 00127 00128 #endif
Generated on Thu Jul 14 2022 07:16:02 by
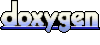