a library to use GPRS like ethernet or wifi, which makes it possible to connect to the internet with your GPRS module
Dependents: Seeed_GPRS_Library_HelloWorld Seeed_GPRS_Xively_HelloWorld Seeed_ARCH_GPRS_V2_Xively_HelloWorld Seeed_ARCH_GPRS_V2_ThingSpeak_HelloWorld ... more
GPRS.h
00001 /* 00002 GPRS.h 00003 2014 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet zou(lawliet.zou@gmail.com) 00006 2014-2-24 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #ifndef __GPRS_H__ 00024 #define __GPRS_H__ 00025 00026 #include "mbed.h" 00027 #include "modem.h" 00028 00029 #define DEFAULT_WAIT_RESP_TIMEOUT 500 00030 #define MAX_SOCK_NUM 7 //(0~6) 00031 00032 enum Protocol { 00033 CLOSED = 0, 00034 TCP = 1, 00035 UDP = 2, 00036 }; 00037 00038 class GPRS: public Modem 00039 { 00040 00041 public: 00042 /** Constructor 00043 * @param tx mbed pin to use for tx line of Serial interface 00044 * @param rx mbed pin to use for rx line of Serial interface 00045 * @param baudRate serial communicate baud rate 00046 * @param apn name of the gateway for GPRS to connect to the network 00047 * @param userName apn's username, usually is NULL 00048 * @param passWord apn's password, usually is NULL 00049 */ 00050 GPRS(PinName tx, PinName rx, int baudRate, const char* apn, const char* userName = NULL, const char *passWord = NULL); 00051 00052 /** get instance of GPRS class 00053 */ 00054 static GPRS* getInstance() { 00055 return inst; 00056 }; 00057 00058 /** Connect the GPRS module to the network. 00059 * @return true if connected, false otherwise 00060 */ 00061 bool join(void); 00062 00063 /** Disconnect the GPRS module from the network 00064 * @returns true if successful 00065 */ 00066 bool disconnect(void); 00067 00068 /** Close a tcp connection 00069 * @returns true if successful 00070 */ 00071 bool close(int socket); 00072 00073 /** Open a tcp/udp connection with the specified host on the specified port 00074 * @param socket an endpoint of an inter-process communication flow of GPRS module,for SIM900 module, it is in [0,6] 00075 * @param ptl protocol for socket, TCP/UDP can be choosen 00076 * @param host host (can be either an ip address or a name. If a name is provided, a dns request will be established) 00077 * @param port port 00078 * @param timeout wait seconds till connected 00079 * @returns true if successful 00080 */ 00081 bool connect(int socket, Protocol ptl, const char * host, int port, int timeout = DEFAULT_TIMEOUT); 00082 00083 /** Set the protocol (UDP or TCP) 00084 * @param socket socket 00085 * @param p protocol 00086 * @returns true if successful 00087 */ 00088 bool setProtocol(int socket, Protocol p); 00089 00090 /** Reset the GPRS module 00091 */ 00092 void reset(); 00093 00094 /** check if GPRS module is readable or not 00095 * @returns true if readable 00096 */ 00097 bool readable(void); 00098 00099 /** wait a few time to check if GPRS module is readable or not 00100 * @param socket socket 00101 * @param wait_time time of waiting 00102 */ 00103 int wait_readable(int socket, int wait_time); 00104 00105 /** wait a few time to check if GPRS module is writeable or not 00106 * @param socket socket 00107 * @param wait_time time of waiting 00108 */ 00109 int wait_writeable(int socket, int req_size); 00110 00111 /** Check if a tcp link is active 00112 * @returns true if successful 00113 */ 00114 bool is_connected(int socket); 00115 00116 /** send data to socket 00117 * @param socket socket 00118 * @param str string to be sent 00119 * @param len string length 00120 * @returns return bytes that actually been send 00121 */ 00122 int send(int socket, const char * str, int len); 00123 00124 /** read data from socket 00125 * @param socket socket 00126 * @param buf buffer that will store the data read from socket 00127 * @param len string length need to read from socket 00128 * @returns bytes that actually read 00129 */ 00130 int recv(int socket, char* buf, int len); 00131 00132 /** convert the host to ip 00133 * @param host host ip string, ex. 10.11.12.13 00134 * @param ip long int ip address, ex. 0x11223344 00135 * @returns true if successful 00136 */ 00137 bool gethostbyname(const char* host, uint32_t* ip); 00138 00139 int new_socket(); 00140 uint16_t new_port(); 00141 uint32_t _ip; 00142 00143 protected: 00144 00145 bool preInit(); 00146 bool checkSIMStatus(void); 00147 uint32_t str_to_ip(const char* str); 00148 static GPRS* inst; 00149 int socketID; 00150 const char* _apn; 00151 const char* _userName; 00152 const char* _passWord; 00153 }; 00154 00155 #endif
Generated on Thu Jul 14 2022 07:16:02 by
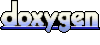