offer some API for GPRS use, such as call / sms / tcp connect
Dependents: ARCH_GPRS_Test ARCH_GPRS_Xively GPRS_Shield_Test Seeed_Arch_GPRS_V2_HelloWorld
gprs.h
00001 /* 00002 gprs.h 00003 2013 Copyright (c) Seeed Technology Inc. All right reserved. 00004 00005 Author:lawliet.zou@gmail.com 00006 2013-11-14 00007 00008 This library is free software; you can redistribute it and/or 00009 modify it under the terms of the GNU Lesser General Public 00010 License as published by the Free Software Foundation; either 00011 version 2.1 of the License, or (at your option) any later version. 00012 00013 This library is distributed in the hope that it will be useful, 00014 but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00016 Lesser General Public License for more details. 00017 00018 You should have received a copy of the GNU Lesser General Public 00019 License along with this library; if not, write to the Free Software 00020 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00021 */ 00022 00023 #ifndef __GPRS_H__ 00024 #define __GPRS_H__ 00025 00026 #include <stdio.h> 00027 #include "mbed.h" 00028 00029 #define DEFAULT_TIMEOUT 5 00030 #define SMS_MAX_LENGTH 16 00031 00032 00033 enum GPRS_MESSAGE { 00034 MESSAGE_RING = 0, 00035 MESSAGE_SMS = 1, 00036 MESSAGE_ERROR 00037 }; 00038 00039 00040 /** GPRS class. 00041 * Used for mobile communication. attention that GPRS module communicate with MCU in serial protocol 00042 */ 00043 class GPRS 00044 { 00045 public: 00046 /** Create GPRS instance 00047 * @param tx uart transmit pin to communicate with GPRS module 00048 * @param rx uart receive pin to communicate with GPRS module 00049 * @param baudRate baud rate of uart communication 00050 * @param number default phone number during mobile communication 00051 */ 00052 GPRS(PinName tx, PinName rx, int baudRate,char *number) : gprsSerial(tx, rx) { 00053 //gprsSerial.baud(baudRate); 00054 phoneNumber = number; 00055 }; 00056 00057 int powerCheck(void); 00058 /** init GPRS module including SIM card check & signal strength & network check 00059 * @returns 00060 * 0 on success 00061 * -1 on error 00062 */ 00063 int init(void); 00064 00065 /** check SIM card' Status 00066 * @returns 00067 * 0 on success 00068 * -1 on error 00069 */ 00070 int checkSIMStatus(void); 00071 00072 /** check signal strength 00073 * @returns 00074 * signal strength in number(ex 3,4,5,6,7,8...) on success 00075 * -1 on error 00076 */ 00077 int checkSignalStrength(void); 00078 00079 /** set SMS format and processing mode 00080 * @returns 00081 * 0 on success 00082 * -1 on error 00083 */ 00084 int settingSMS(void); 00085 00086 /** send text SMS 00087 * @param *number phone number which SMS will be send to 00088 * @param *data message that will be send to 00089 * @returns 00090 * 0 on success 00091 * -1 on error 00092 */ 00093 int sendSMS(char *number, char *data); 00094 00095 /** read SMS by index 00096 * @param *message buffer used to get SMS message 00097 * @param index which SMS message to read 00098 * @returns 00099 * 0 on success 00100 * -1 on error 00101 */ 00102 int readSMS(char *message, int index); 00103 00104 /** delete SMS message on SIM card 00105 * @param *index the index number which SMS message will be delete 00106 * @returns 00107 * 0 on success 00108 * -1 on error 00109 */ 00110 int deleteSMS(int index); 00111 00112 /** read SMS when coming a message,it will be store in messageBuffer. 00113 * @param message buffer used to get SMS message 00114 */ 00115 int getSMS(char* message); 00116 00117 /** call someone 00118 * @param *number the phone number which you want to call 00119 * @returns 00120 * 0 on success 00121 * -1 on error 00122 */ 00123 int callUp(char *number); 00124 00125 /** auto answer if coming a call 00126 * @returns 00127 * 0 on success 00128 * -1 on error 00129 */ 00130 int answer(void); 00131 00132 /** a loop to wait for some event. if a call comes in, it will auto answer it and if a SMS message comes in, it will read the message 00133 * @param *check whether to check phone number when get event 00134 * @returns 00135 * 0 on success 00136 * -1 on error 00137 */ 00138 int loopHandle(void); 00139 00140 /** gprs network init 00141 * @param *apn Access Point Name to connect network 00142 * @param *userName general is empty 00143 * @param *passWord general is empty 00144 */ 00145 int networkInit(char* apn, char* userName = NULL, char* passWord = NULL); 00146 /** build TCP connect 00147 * @param *ip ip address which will connect to 00148 * @param *port TCP server' port number 00149 * @returns 00150 * 0 on success 00151 * -1 on error 00152 */ 00153 int connectTCP(char *ip, char *port); 00154 00155 /** send data to TCP server 00156 * @param *data data that will be send to TCP server 00157 * @returns 00158 * 0 on success 00159 * -1 on error 00160 */ 00161 int sendTCPData(char *data); 00162 00163 /** close TCP connection 00164 * @returns 00165 * 0 on success 00166 * -1 on error 00167 */ 00168 int closeTCP(void); 00169 00170 /** close TCP service 00171 * @returns 00172 * 0 on success 00173 * -1 on error 00174 */ 00175 int shutTCP(void); 00176 00177 Serial gprsSerial; 00178 //USBSerial pc; 00179 private: 00180 /** read from GPRS module and save to buffer array 00181 * @param *buffer buffer array to save what read from GPRS module 00182 * @param *count the maximal bytes number read from GPRS module 00183 * @returns 00184 * 0 on success 00185 * -1 on error 00186 */ 00187 int readBuffer(char *buffer,int count); 00188 00189 /** send AT command to GPRS module 00190 * @param *cmd command array which will be send to GPRS module 00191 */ 00192 void sendCmd(char *cmd); 00193 00194 /** check GPRS module response before timeout 00195 * @param *resp correct response which GPRS module will return 00196 * @param *timeout waiting seconds till timeout 00197 * @returns 00198 * 0 on success 00199 * -1 on error 00200 */ 00201 int waitForResp(char *resp, int timeout); 00202 00203 /** send AT command to GPRS module and wait for correct response 00204 * @param *cmd AT command which will be send to GPRS module 00205 * @param *resp correct response which GPRS module will return 00206 * @param *timeout waiting seconds till timeout 00207 * @returns 00208 * 0 on success 00209 * -1 on error 00210 */ 00211 int sendCmdAndWaitForResp(char *cmd, char *resp, int timeout); 00212 00213 Timer timeCnt; 00214 char *phoneNumber; 00215 char messageBuffer[SMS_MAX_LENGTH]; 00216 }; 00217 00218 #endif 00219
Generated on Wed Jul 13 2022 23:09:51 by
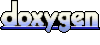