OB1203 basic mbed driver
Embed:
(wiki syntax)
Show/hide line numbers
OB1203.h
00001 #ifndef __OB1203_H__ 00002 #define __OB1203_H__ 00003 00004 #include "mbed.h" 00005 //#include "SoftI2C.h" 00006 00007 //#define DEBUG 00008 00009 #define OB1203_ADDR 0xA6 00010 00011 //Define registers 00012 #define REG_STATUS_0 0x00 00013 #define REG_STATUS_1 0x01 00014 #define REG_PS_DATA 0x02 00015 #define REG_LS_W_DATA 0x04 00016 #define REG_LS_G_DATA 0x07 00017 #define REG_LS_B_DATA 0x0A 00018 #define REG_LS_R_DATA 0x0D 00019 #define REG_LS_C_DATA 0x10 00020 #define REG_TEMP_DATA 0x13 00021 #define REG_MAIN_CTRL_0 0x15 00022 #define REG_MAIN_CTRL_1 0x16 00023 #define REG_PS_LED_CURR 0x17 00024 #define REG_PS_CAN_PULSES 0x19 00025 #define REG_PS_PWIDTH_RATE 0x1A 00026 #define REG_PS_CAN_DIG 0x1B 00027 #define REG_PS_MOV_AVG_HYS 0x1D 00028 #define REG_PS_THRES_HI 0x1E 00029 #define REG_PS_THRES_LO 0x20 00030 #define REG_LS_RES_RATE 0x22 00031 #define REG_LS_GAIN 0x23 00032 #define REG_LS_THRES_HI 0x24 00033 #define REG_LS_THRES_LO 0x27 00034 #define REG_LS_THRES_VAR 0x2A 00035 #define REG_INT_CFG_0 0x2B 00036 #define REG_PS_INT_CFG_1 0x2C 00037 #define REG_INT_PST 0x2D 00038 #define REG_PPG_PS_GAIN 0x2E 00039 #define REG_PPG_PS_CFG 0x2F 00040 #define REG_PPG_IRLED_CURR 0x30 00041 #define REG_PPG_RLED_CURR 0x32 00042 #define REG_PPG_CAN_ANA 0x34 00043 #define REG_PPG_AVG 0x35 00044 #define REG_PPG_PWIDTH_RATE 0x36 00045 #define REG_FIFO_CFG 0x37 00046 #define REG_FIFO_WR_PTR 0x38 00047 #define REG_FIFO_RD_PTR 0x39 00048 #define REG_FIFO_OVF_CNT 0x3A 00049 #define REG_FIFO_DATA 0x3B 00050 #define REG_PART_ID 0x3D 00051 #define REG_DEVICE_CFG 0x4D 00052 #define REG_OSC_TRIM 0x3E 00053 #define REG_LED_TRIM 0x3F 00054 #define REG_BIO_TRIM 0x40 00055 #define REG_DIG_LED1_TRIM 0x42 00056 #define REG_DIG_LED2_TRIM 0x43 00057 00058 //Define settings 00059 //STATUS_0 00060 #define POR_STATUS 0x80 00061 #define LS_INT_STATUS 0x02 00062 #define LS_NEW_DATA 0x01 00063 //STATUS_1 00064 #define LED_DRIVER_STATUS 0x40 00065 #define FIFO_AFULL_STATUS 0x20 00066 #define PPG_DATA_STATUS 0x10 00067 #define PS_LOGIC_STATUS 0x04 00068 #define PS_INT_STATUS 0x02 00069 #define PS_NEW_DATA 0x01 00070 //MAIN_CTRL_0 00071 #define SW_RESET 0x01<<7 00072 #define LS_SAI_ON 0x01<<3 00073 #define LS_SAI_OFF 0x00 00074 #define ALS_MODE 0x00 00075 #define RGB_MODE 0x01<<1 00076 #define LS_OFF 0x00 00077 #define LS_ON 0x01 00078 //MAIN_CTRL_1 00079 #define PS_SAI_ON 0x01<<3 00080 #define PS_SAI_OFF 0x00 00081 #define PS_MODE 0x00 00082 #define HR_MODE 0x01<<1 00083 #define SPO2_MODE 0x02<<1 00084 #define PPG_PS_ON 0x01 00085 #define PPG_PS_OFF 0x00 00086 #define TEMP_ON 0x01<<7 00087 #define TEMP_OFF 0x00 00088 //PS_CAN_PULSES 00089 #define PS_CAN_ANA_0 0x00 //off 00090 #define PS_CAN_ANA_1 0x01<<6 //50% of FS 00091 #define PS_CAN_ANA_2 0x02<<6 //100% of FS 00092 #define PS_CAN_ANA_3 0x03<<6 //150% of FS 00093 #define PS_PULSES(x) (( (x) & (0x07) )<<3) //where x = 0..5 and num pulses = 2^x 00094 //PS_PWIDTH_RATE 00095 #define PS_PWIDTH(x) (x & 0x03)<<4 //where x = 0..3 00096 #define PS_RATE(x) (x & 0x07) //where x = 0..7 00097 //PS_MOV_AVG_HYS 00098 #define PS_AVG_ON 1<<7 00099 #define PS_AVG_OFF 0 00100 #define PS_HYS_LEVEL(x) x>>1 //where x=0..256 00101 //LS_RES_RATE 00102 #define LS_RES(x) (x & 0x07)<<4 //where x=0..7 00103 #define LS_RATE(x) (x & 0x07) //where x=0..7 00104 00105 #define LS_RES_20 0x00 00106 #define LS_RES_19 0x01 00107 #define LS_RES_18 0x02 00108 #define LS_RES_17 0x03 00109 #define LS_RES_16 0x04 00110 #define LS_RES_13 0x05 00111 00112 //LS_GAIN 00113 #define LS_GAIN(x) (x & 0x03) 00114 #define LS_GAIN_1 0x00 00115 #define LS_GAIN_3 0x01 00116 #define LS_GAIN_6 0x10 00117 #define LS_GAIN_20 0x11 00118 00119 //LS_THRES_VAR 00120 #define LS_THRES_VAR(x) (x & 0x07) 00121 //INT_CFG_0 00122 #define LS_INT_SEL_W 0 00123 #define LS_INT_SEL_G 1<<4 00124 #define LS_INT_SEL_R 2<<4 00125 #define LS_INT_SEL_B 3<<4 00126 #define LS_THRES_INT_MODE 0 00127 #define LS_VAR_INT_MODE 1<<1 00128 #define LS_INT_ON 1 00129 #define LS_INT_OFF 0 00130 //INT_CFG_1 00131 #define AFULL_INT_ON 1<<5 00132 #define AFULL_INT_OFF 0 00133 #define PPG_INT_ON 1<<4 00134 #define PPG_INT_OFF 0 00135 #define PS_INT_READ_CLEARS 0<<1 00136 #define PS_INT_LOGIC 1 00137 #define PS_INT_ON 1 00138 #define PS_INT_OFF 0 00139 00140 //INT_PST 00141 #define LS_PERSIST(x) (x & 0x0F)<<4 00142 #define PS_PERSIST(x) (x & 0x0F) 00143 //PPG_PS_GAIN 00144 #define PPG_PS_GAIN_1 0 00145 #define PPG_PS_GAIN_1P5 1<<4 00146 #define PPG_PS_GAIN_2 2<<4 00147 #define PPG_PS_GAIN_4 3<<4 00148 #define PPG_LED_SETTLING(x) (x & 0x03)<<2 // 0=0us, 1 = 5us, 2 = 10us (Default), 3=20us 00149 #define PPG_ALC_TRACK(x) (x & 0x03) // 0 = 10us, 1 (20us) DEFAULT ,2 = 30us, 3 = 60us 00150 //PPG_PS_CFG 00151 #define PPG_POW_SAVE_ON 1<<6 00152 #define PPG_POW_SAVE_OFF 0 00153 #define LED_FLIP_ON 1<<3 00154 #define LED_FLIP_OFF 0 00155 #define DIFF_OFF 2 00156 #define ALC_OFF 1 00157 #define DIFF_ON 0 00158 #define ALC_ON 0 00159 #define SIGNAL_OUT 1<<2 00160 #define OFFSET_OUT 0 00161 //PPG_CAN_ANA 00162 #define PPG_CH1_CAN(x) (x & 0x03)<<2 00163 #define PPG_CH2_CAN(x) (x & 0x03) 00164 //PPG_AVG 00165 #define PPG_AVG(x) (x & 0x07)<<4 00166 //PPG_PWIDTH_RATE 00167 #define PPG_PWIDTH(x) (x & 0x07)<<4 00168 #define PPG_FREQ_PREPRODUCTION 0 00169 #define PPG_FREQ_PRODUCTION 1 00170 #define PPG_RATE(x) (x & 0x07) 00171 //FIFO_CFG 00172 #define FIFO_ROLL_ON 1<<4 00173 #define FIFO_ROLL_OFF 0 00174 #define AFULL_ADVANCE_WARNING(x) (x & 0x0F) 00175 00176 00177 00178 00179 00180 #define POR_TIME_MS 5 //a guess 00181 00182 00183 #define IR_TARGET_COUNTS 196608 00184 #define R_TARGET_COUNTS 196608 00185 #define TOL1 6000 00186 #define TOL2 40000 00187 #define STEP 8 00188 #define IN_RANGE_PERSIST 4 00189 #define IR_MAX_CURRENT 0x02AF 00190 #define R_MAX_CURRENT 0x01FF 00191 00192 00193 class OB1203 00194 { 00195 I2C *i2c; 00196 00197 public: 00198 char osc_trim; 00199 char ls_res; 00200 char ls_rate; 00201 char ls_gain; 00202 uint32_t ls_thres_hi; 00203 uint32_t ls_thres_lo; 00204 char ls_sai; 00205 char ls_mode; 00206 char ls_en; 00207 char ls_int_sel; 00208 char ls_var_mode; 00209 char ps_sai_en; 00210 char temp_en; 00211 char ppg_ps_mode; 00212 char ppg_ps_en; 00213 char ps_can_ana; 00214 char afull_int_en; 00215 char ppg_int_en; 00216 char ps_logic_mode; 00217 uint16_t ps_digital_can; 00218 char ps_int_en; 00219 char ls_persist; 00220 char ps_persist; 00221 uint16_t ps_thres_hi; 00222 uint16_t ps_thres_lo; 00223 uint16_t ps_current; 00224 volatile uint16_t ir_current; 00225 volatile uint16_t r_current; 00226 char ppg_ps_gain; 00227 char ppg_pow_save; 00228 char led_flip; 00229 char ch1_can_ana; 00230 char ch2_can_ana; 00231 char ppg_avg; 00232 char ppg_pwidth; 00233 char ppg_freq; 00234 char ppg_rate; 00235 char ppg_LED_settling; 00236 char ppg_ALC_track; 00237 char ps_pulses; 00238 char ps_pwidth; 00239 char ps_rate; 00240 char ps_avg_en; 00241 char ps_hys_level; 00242 char ls_int_en; 00243 char fifo_rollover_en; 00244 char fifo_afull_advance_warning; 00245 char writePointer; 00246 char readPointer; 00247 char fifoOverflow; 00248 char bio_trim; 00249 char led_trim; 00250 char diff; 00251 char alc; 00252 char sig_out; 00253 char led1_trim; 00254 char led2_trim; 00255 00256 OB1203 (I2C *); 00257 00258 // Low-level operations 00259 void reset(); 00260 uint16_t get_status(); 00261 void writeRegister(int, char, char); 00262 void writeBlock(int, char, char *, char); 00263 void readBlock(int, char, char *, int); 00264 uint32_t bytes2uint32(char *, int); 00265 uint32_t twoandhalfBytes2uint32(char *, int); 00266 00267 // High-level operations 00268 void setOscTrim(); 00269 bool dataIsNew(); 00270 bool lsIsNew(); 00271 bool psIsNew(); 00272 bool tempIsNew(); 00273 bool bioIsNew(); 00274 void setMainConfig(); 00275 void setIntConfig(); 00276 void setLSthresh(); 00277 void setPSthresh(); 00278 void setPScurrent(); 00279 void setPPGcurrent(); 00280 void setPPG_PSgain_cfg(); 00281 void setPPGana_can(); 00282 void setDigitalCan(); 00283 void setPPGavg_and_rate(); 00284 void setFifoConfig(); 00285 void setBioTrim(); 00286 void setLEDTrim(); 00287 char get_ps_data(uint32_t *); 00288 char get_ls_data(uint32_t *); 00289 char get_ps_ls_data(uint32_t *); 00290 void resetFIFO(); 00291 void init_ps(); 00292 void init_rgb(); 00293 void init_ps_rgb(); 00294 void init_hr(); 00295 void init_spo2(); 00296 void getFifoInfo(char *fifo_info); 00297 void getNumFifoSamplesAvailable(char *fifo_info, char *sample_info); 00298 void getFifoSamples(uint8_t, char *); 00299 void parseFifoSamples(char, char *, uint32_t *); 00300 char get_part_ID(char *); 00301 void setDigTrim(void); 00302 00303 //agc functions 00304 void do_agc(uint32_t,bool); 00305 00306 //variables 00307 uint16_t rate; 00308 char res; 00309 char gain; 00310 uint32_t data_max; 00311 uint32_t reg_max; 00312 00313 00314 // const uint32_t targetCounts[2]; 00315 00316 bool update; 00317 00318 }; 00319 00320 00321 #endif
Generated on Wed Jul 20 2022 11:26:56 by
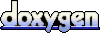