
A port of the arduino voltmeter example for the mbed using the 4.3' PCT 4d systems touch screen display. Uses the mbed_genie library ported from the arduino visie-genie library by Christian B
main.cpp
00001 /* A port test of the arduino voltmeter example using the 00002 4.3' PCT 4d systems touch screen display. 00003 00004 uses the mbed_genie library ported from the arduino 00005 visie-genie library by Christian B 00006 00007 The display serial TX and RX pins are connected to pin 9 00008 and pin 10 of the mbed 00009 00010 The reset pin is not connected as reset function is not implemented 00011 00012 Pin 15 of the mbed has a potentiometer wiper connected to it 00013 The other connections of the potentiometer are connected to +3.3V 00014 and 0V 00015 00016 For setting up the display in Visie-Genie 00017 00018 The display has an angular meter objecr, a LED digits object, two buttons 00019 and three static text objects. 00020 00021 The program sends digital voltage readings to the LED digits and 00022 angular meter of the display module. 00023 00024 The On and Off button has been set to report on change 00025 00026 The baud rate of the display is set to 115200 baud 00027 00028 */ 00029 00030 #include "mbed.h" 00031 #include "mbed_genie.h" 00032 00033 DigitalOut myled(LED1); //LED 1 for indication 00034 00035 AnalogIn voltReading(p15); //Potentiometer wiper connected to pin 15 00036 00037 int flag = 0; //holds the "power status" of the voltmeter. flag = 0 means voltmeter is "off", flag = 1 means the voltmeter is "on". 00038 float voltMeter; //holds the digital voltage value to be sent to the angular meter 00039 float voltLED; //holds the digital voltage value to be sent to the LED digits 00040 00041 //Event handler for the 4d Systems display 00042 void myGenieEventHandler(void) 00043 { 00044 genieFrame Event; 00045 genieDequeueEvent(&Event); 00046 //event report from an object 00047 if(Event.reportObject.cmd == GENIE_REPORT_EVENT) 00048 { 00049 /* 00050 for example here we check if we received a message from 4dbuttons objects 00051 the index is the button number, refer to the 4dgenie project to know the index 00052 */ 00053 if (Event.reportObject.object == GENIE_OBJ_4DBUTTON) // If the Reported Message was from a button 00054 { 00055 if (Event.reportObject.index == 0) 00056 { 00057 //printf("Off Button pressed!\n\r"); 00058 wait(0.2); 00059 flag=1; 00060 } 00061 if (Event.reportObject.index == 1) 00062 { 00063 //printf("On Button pressed!\n\r"); 00064 wait(0.2); 00065 flag=0; 00066 } 00067 } 00068 } 00069 00070 //Cmd from a reported object (happens when an object read is requested) 00071 // if(Event.reportObject.cmd == GENIE_REPORT_OBJ) 00072 // { 00073 00074 // } 00075 00076 } 00077 00078 int main() 00079 00080 { 00081 SetupGenie(); 00082 genieAttachEventHandler(&myGenieEventHandler); 00083 //genieResetDisplay(); 00084 00085 printf("Langsters's mbed Visi-Genie Voltmeter demo \n\r"); 00086 00087 genieWriteContrast(15); //set screen contrast to full brightness 00088 00089 while(1) 00090 { 00091 //voltLED = 0; 00092 00093 if (flag == 1) 00094 { 00095 //printf("Flag status: %d \r\n", flag); 00096 wait (0.1); 00097 voltLED = voltReading; 00098 wait (0.1); 00099 //printf("Volt bit Reading: %f \n\r",voltLED); 00100 voltLED = voltLED * 3.3; //convert float reading to voltage 00101 //printf("voltLED: %f\r\n",voltLED); 00102 00103 voltLED = voltLED * 1000; 00104 genieWriteObject(GENIE_OBJ_LED_DIGITS, 0x00, voltLED); //write to Leddigits0 the value of voltLED 00105 wait (0.1); 00106 00107 voltMeter = voltLED/100; 00108 genieWriteObject(GENIE_OBJ_ANGULAR_METER, 0x00, voltMeter); //write to Angularmeter0 the value of voltMeter 00109 } 00110 00111 else if(flag == 0) 00112 00113 { 00114 //printf("Flag status: %d \r\n", flag); 00115 wait (0.1); 00116 voltLED = 0; 00117 genieWriteObject(GENIE_OBJ_LED_DIGITS, 0x00, 0); //write to Leddigits0 the value of voltLED 00118 wait (0.1); 00119 00120 //voltMeter = voltLED/100; 00121 genieWriteObject(GENIE_OBJ_ANGULAR_METER, 0x00, 0); //write to Angularmeter0 the value of voltMeter 00122 } 00123 00124 } 00125 00126 }
Generated on Sun Jul 24 2022 04:23:44 by
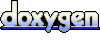