
Basic example showing how to use the ePaper Display (ePD) present on the STM32L053 Discovery (STM32L0538-DISCO) board.
Dependencies: EPD_GDE021A1 lib_dht22 mbed
Fork of DISCO-L053C8_ePD_demo by
main.cpp
00001 #include "mbed.h" 00002 #include "EPD_GDE021A1.h" 00003 #include "dht22.h" 00004 00005 #define EPD_CS PA_15 00006 #define EPD_DC PB_11 00007 #define EPD_RESET PB_2 00008 #define EPD_BUSY PA_8 00009 #define EPD_POWER PB_10 00010 #define EPD_SPI_MOSI PB_5 00011 #define EPD_SPI_MISO PB_4 00012 #define EPD_SPI_SCK PB_3 00013 #define PTB18 PC_15 00014 00015 EPD_GDE021A1 epd(EPD_CS, EPD_DC, EPD_RESET, EPD_BUSY, EPD_POWER, EPD_SPI_MOSI, EPD_SPI_MISO, EPD_SPI_SCK); 00016 00017 DigitalOut led1(LED1); 00018 DigitalOut led2(LED2); 00019 00020 Serial host(USBTX, USBRX); 00021 DHT22 dht22(PTB18); 00022 00023 //width 48 00024 //height 26 00025 static uint8_t Battery_img[] = { 00026 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00027 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00028 0xfe, 0xff, 0xff, 0xff, 0xff, 0x0f, 00029 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x0c, 00030 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00031 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00032 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00033 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00034 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x18, 00035 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00036 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00037 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00038 0xfe, 0xff, 0xff, 0x3f, 0xff, 0x39, 00039 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00040 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00041 0xfe, 0xff, 0xff, 0x3f, 0x10, 0x38, 00042 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x38, 00043 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x18, 00044 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00045 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00046 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x08, 00047 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x0c, 00048 0xfe, 0xff, 0xff, 0x3f, 0x00, 0x0c, 00049 0xfe, 0xff, 0xff, 0xff, 0xff, 0x0f, 00050 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00051 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 00052 }; 00053 00054 int main() 00055 { 00056 led1 = 1; 00057 DHT22_data_t dht22_data; 00058 00059 epd.Clear(EPD_COLOR_WHITE); 00060 epd.DisplayStringAtLine(5, (uint8_t*)"MBED", CENTER_MODE); 00061 epd.DisplayStringAtLine(3, (uint8_t*)"Epaper display", LEFT_MODE); 00062 epd.DisplayStringAtLine(2, (uint8_t*)"demo", LEFT_MODE); 00063 epd.DrawImage(130, 0, 48, 26, Battery_img); 00064 epd.DrawRect(50, 4, 60, 4); 00065 epd.RefreshDisplay(); 00066 wait(1); 00067 00068 while(1) { 00069 led1 = 1; 00070 wait_ms(500); 00071 00072 dht22.read(&dht22_data); 00073 00074 float temperature = dht22_data.temp / 10.0f; 00075 float humidity = dht22_data.humidity / 10.0f; 00076 00077 host.printf("Temperature: %2.2f Humidity: %2.2f%%\r\n", temperature, humidity); 00078 wait_ms(500); 00079 00080 char str1[5], str2[5]; 00081 sprintf(str1, "%.1f", temperature); 00082 sprintf(str2, "%.1f", humidity); 00083 00084 led1 = 0; 00085 led2 = 1; 00086 00087 epd.Clear(EPD_COLOR_WHITE); 00088 epd.DisplayStringAtLine(5, (uint8_t*)"Adrien", CENTER_MODE); 00089 epd.DisplayStringAtLine(3, (uint8_t*)"Temperature: ", LEFT_MODE); 00090 epd.DisplayStringAtLine(3, (uint8_t*)str1, RIGHT_MODE); 00091 epd.DisplayStringAtLine(2, (uint8_t*)"Humidity: ", LEFT_MODE); 00092 epd.DisplayStringAtLine(2, (uint8_t*)str2, RIGHT_MODE); 00093 epd.RefreshDisplay(); 00094 00095 led2 = 0; 00096 wait_ms(500); 00097 } 00098 }
Generated on Thu Jul 14 2022 20:22:47 by
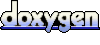