
cansat
Dependencies: BMP085 JPEGCamera SDFileSystem mbed
Fork of SaibiCansat2014 by
FastJpegCamera.h
00001 #ifndef FAST_JPEG_CAMERA_H 00002 #define FAST_JPEG_CAMERA_H 00003 00004 #include "JPEGCamera/JPEGCamera.h" 00005 00006 #define FILENAME "/sd/pic%05d.jpg" 00007 00008 #define min(x, y) ((x) < (y)) ? (x) : (y) 00009 00010 class FastJpegCamera : public JPEGCamera { 00011 public: 00012 DigitalOut myled1; //show successful picture was taken 00013 int baudrate; 00014 Serial pc; 00015 Serial& xbee; 00016 00017 FastJpegCamera(PinName tx, PinName rx, Serial& xbee) : JPEGCamera(tx,rx), baudrate(38400), pc(USBTX, USBRX), myled1(LED1), xbee(xbee){ 00018 this->baud115200(); 00019 }; 00020 00021 void baud115200(){ 00022 char buf[7] = {0x56, 0x00, 0x24, 0x03, 0x01, 0x0d, 0xa6}; 00023 //char buf[5] = {0x56, 0x00, 0x34, 0x0d, 0xa6}; 00024 //int ret = sendReceive(buf, sizeof buf, 5); 00025 for (int i = 0; i < sizeof buf; i++) putc(buf[i]); 00026 wait(0.5); 00027 this->baudrate = 115200; 00028 this->baud(this->baudrate); 00029 wait(4); 00030 //this->reset(); 00031 receive(buf, 5, 500); 00032 pc.printf("%02x %02x %02x %02x %02x", buf[0], buf[1], buf[2], buf[3], buf[4]); 00033 }; 00034 00035 void baud57600(){ 00036 char buf[7] = {0x56, 0x00, 0x24, 0x03, 0x01, 0x1c, 0x4c}; 00037 int ret = sendReceive(buf, sizeof buf, 5); 00038 wait(0.5); 00039 this->reset(); 00040 this->baudrate = 57600; 00041 this->baud(this->baudrate); 00042 }; 00043 00044 00045 void baud38400(){ 00046 char buf[7] = {0x56, 0x00, 0x24, 0x03, 0x01, 0x2a, 0xf2}; 00047 //int ret = sendReceive(buf, sizeof buf, 5); 00048 for (int i = 0; i < sizeof buf; i++) putc(buf[i]); 00049 wait(0.5); 00050 //this->reset(); 00051 this->baudrate = 38400; 00052 this->baud(this->baudrate); 00053 wait(4); 00054 //this->reset(); 00055 receive(buf, 5, 500); 00056 pc.printf("%02x %02x %02x %02x %02x", buf[0], buf[1], buf[2], buf[3], buf[4]); 00057 }; 00058 00059 bool processPicture() { 00060 if (state == PROCESSING) { 00061 if (address < imageSize) { 00062 char data[1024]; 00063 int size = readData(data, min(sizeof(data), imageSize - address), address); 00064 int ret = fwrite(data, size, 1, fp); 00065 if (ret > 0) 00066 address += size; 00067 if (ret == 0 || address >= imageSize) { 00068 stopPictures(); 00069 fclose(fp); 00070 wait(0.1); // ???? 00071 state = ret > 0 ? READY : ERROR; 00072 }//if 00073 //for(int i=0; i<sizeof data; ++i){ 00074 // xbee.putc(data[i]); 00075 // //pc.printf("%02x ", data[i]); 00076 //}//for 00077 }//if 00078 }//if 00079 return state == PROCESSING || state == READY; 00080 }//processPicture 00081 00082 void shoot(int i){ 00083 00084 if (!this->isReady()) { 00085 pc.printf("show camera is not ready\n"); 00086 //myled4 = 1; //show camera is not ready 00087 //wait(2.0); 00088 //myled4 = 0; 00089 return; 00090 }//if 00091 00092 char filename[32]; 00093 sprintf(filename, FILENAME, i); 00094 printf("Picture: %s ", filename); 00095 myled1 = 1; 00096 if (this->takePicture(filename)) { 00097 while (this->isProcessing()) { 00098 this->processPicture(); 00099 }//while 00100 //myled1 = 1; //show successful picture was taken 00101 //wait(2.0); 00102 //myled1 = 0; 00103 } else { 00104 pc.printf("shot %s\n", filename); 00105 //myled3 = 1; //show picture take failed 00106 //wait(2.0); 00107 //myled3 = 0; 00108 }//if 00109 this->stopPictures(); 00110 myled1 = 0; 00111 };//shoot 00112 00113 00114 };//FastJpegCamera 00115 00116 #endif
Generated on Mon Jul 18 2022 12:26:52 by
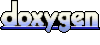