
Car Bon car module
Dependencies: C027_Support HTTPClient-basicAuth M2XStreamClient jsonlite mbed
main.cpp
00001 #include "mbed.h" 00002 #include "MDM.h" 00003 #include "HTTPClient.h" 00004 #include "M2XStreamClient.h" 00005 00006 // M2X stuff 00007 char feedId[] = "c6eabf437b8c69efbb4e4a8d5c60c04d"; // Feed you want to post to 00008 char m2xKey[] = "10bc8a4dc4a37c5dc549b41ffaa6d6c1"; // Your M2X access key 00009 char streamName[] = "danger_bit"; // Stream you want to post to 00010 00011 // Connected Car stuff 00012 char ccUser[] = "provider"; 00013 char ccPass[] = "1234"; 00014 char ccOnUrl[] = "http://toy.hack.att.io:3000/remoteservices/v1/vehicle/engineOn/1234567890abcd"; 00015 char ccOffUrl[] = "http://toy.hack.att.io:3000/remoteservices/v1/vehicle/engineOff/1234567890abcd"; 00016 00017 Ticker sensortick; 00018 00019 DigitalOut heat(P2_13); 00020 AnalogIn sensor(P0_23); 00021 00022 int volatile reading; 00023 bool volatile newReading = false; 00024 00025 void sensor_isr() 00026 { 00027 reading = (int)(sensor.read() * 1000); 00028 newReading = true; 00029 } 00030 00031 int main() 00032 { 00033 MDMSerial mdm; 00034 00035 Client client; 00036 M2XStreamClient m2xClient(&client, m2xKey); 00037 00038 DigitalOut led(LED); 00039 00040 led = 1; 00041 sensortick.attach(sensor_isr, 2); 00042 heat = 1; 00043 00044 printf("\nConnecting...\n"); 00045 00046 //mdm.setDebug(4); // enable this for debugging issues 00047 if (!mdm.connect()) 00048 { 00049 printf("Connect failed!\n"); 00050 return -1; 00051 } 00052 00053 led = 0; 00054 printf("Connected!\n"); 00055 set_time(0); 00056 00057 HTTPClient http; 00058 HTTPMap map; 00059 char str[512]; 00060 HTTPText inText(str, 512); 00061 int ret; 00062 00063 http.basicAuth(ccUser, ccPass); 00064 http.customHeaders(NULL, 0); 00065 00066 printf("\nTurning on engine...\n"); 00067 do 00068 { 00069 ret = http.post(ccOnUrl, map, &inText, 15000); 00070 if (!ret) 00071 { 00072 printf("Executed POST successfully - read %d characters\n", strlen(str)); 00073 printf("Result: %s\n", str); 00074 } 00075 else 00076 { 00077 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00078 } 00079 } while (ret); 00080 00081 time_t seconds; 00082 00083 int currentReading, highReading = 1000, prevReading = 1000; 00084 bool alarm = true; 00085 unsigned int wait = 15; 00086 00087 while (1) 00088 { 00089 if (newReading) 00090 { 00091 led = 1; 00092 currentReading = reading; // make a copy because the isr might update it before we're done here 00093 seconds = time(NULL); 00094 printf("%d:%02d:%02d:%02d ", seconds / 86400, (seconds / 3600) % 24, (seconds / 60) % 60, seconds % 60); 00095 printf("Alarm: %s Reading: %d (prev: %d; high: %d)\n", (alarm ? "yes" : "no"), currentReading, prevReading, highReading); 00096 00097 if (!alarm && ((currentReading - prevReading) > 50)) 00098 { 00099 // rise since previous reading 00100 printf("*** Danger!\n"); 00101 00102 printf("Turning off engine...\n"); 00103 do 00104 { 00105 ret = http.post(ccOffUrl, map, &inText, 15000); 00106 if (!ret) 00107 { 00108 printf("Executed POST successfully - read %d characters\n", strlen(str)); 00109 printf("Result: %s\n", str); 00110 } 00111 else 00112 { 00113 printf("Error - ret = %d - HTTP return code = %d\n", ret, http.getHTTPResponseCode()); 00114 } 00115 } while (ret); 00116 00117 do 00118 { 00119 printf("Reporting danger...\n"); 00120 ret = m2xClient.post(feedId, streamName, 1); 00121 printf("Post response code: %d\n", ret); 00122 } while (ret != 204); 00123 00124 highReading = currentReading; 00125 alarm = true; 00126 wait = 0; 00127 } 00128 else if (alarm && ((highReading - currentReading) > 50)) 00129 { 00130 wait++; 00131 if (wait > 15) // 30 s 00132 { 00133 // fall since last high reading 00134 printf("*** Safe!\n"); 00135 00136 00137 do 00138 { 00139 printf("Reporting safe...\n"); 00140 ret = m2xClient.post(feedId, streamName, 0); 00141 printf("Post response code: %d\n", ret); 00142 } while (ret != 204); 00143 00144 alarm = false; 00145 } 00146 } 00147 prevReading = currentReading; 00148 newReading = false; 00149 led = 0; 00150 } 00151 } 00152 00153 /*mdm.disconnect(); 00154 mdm.powerOff(); 00155 00156 while (1);*/ 00157 }
Generated on Tue Jul 19 2022 03:14:06 by
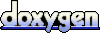