A library for ADT7410 I2C connecting temperature sensor module.
Embed:
(wiki syntax)
Show/hide line numbers
KuADT7410.h
00001 #ifndef KU_ADT7410_H 00002 #define KU_ADT7410_H 00003 00004 #include "mbed.h" 00005 00006 /** 00007 * A library for ADT7410 I2C connecting temperature sensor module. 00008 */ 00009 class KuADT7410 { 00010 private: 00011 I2C &i2c; 00012 int i2c_address; 00013 00014 public: 00015 00016 /** 00017 * Constractor of ADT7410 driver. 00018 * @param i2c I2C object 00019 * @param i2c_address Target's I2C address (LSB is used for R/W flag). 00020 */ 00021 explicit KuADT7410(I2C &i2c, int i2c_address = 0x48 << 1); 00022 00023 /** 00024 * Destractor 00025 */ 00026 ~KuADT7410(); 00027 00028 /** 00029 * Reset target device. 00030 */ 00031 void reset(); 00032 00033 /** 00034 * Get temperature 00035 */ 00036 float get_temp(); 00037 00038 /** 00039 * Get status 00040 */ 00041 unsigned char get_status(); 00042 }; 00043 00044 #endif
Generated on Mon Aug 1 2022 00:49:53 by
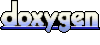