
smart dustbin using hcsr04
Dependencies: HCSR04 TextLCD mbed-rtos mbed
main.cpp
00001 #include "hcsr04.h" 00002 #include "TextLCD.h" 00003 #include "rtos.h" 00004 #include <string> 00005 00006 DigitalOut myled(LED1); 00007 DigitalOut myled1(LED2); 00008 DigitalOut myled2(LED3); 00009 Serial pc(USBTX, USBRX); 00010 //TextLCD lcd(p21,p22,p23,p24,p25,p26); 00011 HCSR04 usensor(p15,p16); 00012 HCSR04 usensor1(p21,p22); 00013 HCSR04 usensor2(p23,p24); 00014 00015 Serial SIM900(p13, p14); //tx, rx SIM 900 00016 string result; 00017 char x; 00018 Thread thread; 00019 unsigned int dist; 00020 unsigned int dist1; 00021 unsigned int dist2; 00022 00023 void clearString() 00024 { 00025 result.clear(); 00026 } 00027 00028 void callback_rx() { 00029 00030 while (SIM900.readable()) { 00031 x = SIM900.getc(); 00032 result += x; 00033 pc.putc(x); // print the answer from SIM900 00034 00035 } 00036 } 00037 00038 void sendSMS1() 00039 { 00040 00041 clearString(); 00042 SIM900.printf("AT+CMGF=1\r\n"); //at command for send sms 00043 wait_ms(1000); 00044 clearString(); 00045 wait_ms(1000); 00046 SIM900.printf("AT+CMGS="); 00047 SIM900.putc('"'); 00048 SIM900.printf("+919405204727"); 00049 SIM900.putc('"'); 00050 SIM900.printf("\r"); 00051 SIM900.printf("\n"); 00052 wait_ms(1000); 00053 SIM900.printf("Bin 1 is full"); 00054 wait_ms(1000); 00055 SIM900.putc(0x1A); 00056 wait_ms(5000); 00057 } 00058 00059 void sendSMS2() 00060 { 00061 00062 clearString(); 00063 SIM900.printf("AT+CMGF=1\r\n"); //at command for send sms 00064 wait_ms(1000); 00065 clearString(); 00066 wait_ms(1000); 00067 SIM900.printf("AT+CMGS="); 00068 SIM900.putc('"'); 00069 SIM900.printf("+919405204727"); 00070 SIM900.putc('"'); 00071 SIM900.printf("\r"); 00072 SIM900.printf("\n"); 00073 wait_ms(1000); 00074 SIM900.printf("Bin 2 is full"); 00075 wait_ms(1000); 00076 SIM900.putc(0x1A); 00077 wait_ms(5000); 00078 } 00079 00080 void sendSMS3() 00081 { 00082 00083 clearString(); 00084 SIM900.printf("AT+CMGF=1\r\n"); //at command for send sms 00085 wait_ms(1000); 00086 clearString(); 00087 wait_ms(1000); 00088 SIM900.printf("AT+CMGS="); 00089 SIM900.putc('"'); 00090 SIM900.printf("+919405204727"); 00091 SIM900.putc('"'); 00092 SIM900.printf("\r"); 00093 SIM900.printf("\n"); 00094 wait_ms(1000); 00095 SIM900.printf("Bin 3 is full"); 00096 wait_ms(1000); 00097 SIM900.putc(0x1A); 00098 wait_ms(5000); 00099 } 00100 00101 00102 void led1_thread() { 00103 while (true) { 00104 wait_ms(10); 00105 sendSMS1(); // SEND SMS 00106 wait_ms(10); 00107 00108 00109 Thread::wait(1000); 00110 } 00111 } 00112 00113 void led2_thread() { 00114 while (true) { 00115 wait_ms(10); 00116 sendSMS2(); // SEND SMS 00117 wait_ms(100); 00118 00119 00120 Thread::wait(1000); 00121 } 00122 } 00123 void led3_thread() { 00124 while (true) { 00125 wait_ms(10); 00126 sendSMS3(); // SEND SMS 00127 wait_ms(100); 00128 00129 Thread::wait(1000); 00130 } 00131 } 00132 00133 00134 int main() 00135 { 00136 SIM900.attach(&callback_rx); 00137 SIM900.baud(9600); // 00138 wait_ms(100); 00139 00140 while(1) 00141 { 00142 usensor.start(); 00143 usensor1.start(); 00144 usensor2.start(); 00145 wait_ms(500); 00146 dist=usensor.get_dist_cm(); 00147 dist1=usensor1.get_dist_cm(); 00148 dist2=usensor2.get_dist_cm(); 00149 // lcd.locate(0,0); 00150 // lcd.printf("distance"); 00151 if(dist<20) 00152 { 00153 myled=1; 00154 thread.start(led1_thread); 00155 // lcd.locate(0,0); 00156 // lcd.printf("dist=cm:%ld\r\n",dist ); 00157 } 00158 else 00159 { 00160 myled=0; 00161 // lcd.printf("abnormal"); 00162 } 00163 00164 if(dist1<20) 00165 { 00166 myled1=1; 00167 thread.start(led2_thread); 00168 //lcd.locate(0,0); 00169 //lcd.printf("dist=cm:%ld\r\n",dist ); 00170 } 00171 else 00172 { 00173 myled1=0; 00174 00175 } 00176 00177 if(dist2<20) 00178 { 00179 myled2=1; 00180 thread.start(led3_thread); 00181 //lcd.locate(0,0); 00182 //lcd.printf("dist=cm:%ld\r\n",dist ); 00183 } 00184 else 00185 { 00186 myled2=0; 00187 //lcd.printf("abnormal"); 00188 } 00189 } 00190 00191 00192 00193 00194 }
Generated on Sat Jul 16 2022 01:44:04 by
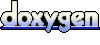