increased chunk size
Fork of MTS-Socket by
Embed:
(wiki syntax)
Show/hide line numbers
UDPSocket.cpp
00001 #include "UDPSocket.h" 00002 #include <string> 00003 #include <algorithm> 00004 00005 UDPSocket::UDPSocket() 00006 { 00007 } 00008 00009 int UDPSocket::init(void) 00010 { 00011 return 0; 00012 } 00013 00014 // Server initialization 00015 int UDPSocket::bind(int port) 00016 { 00017 if (ip->bind(port)) { 00018 return 0; 00019 } else { 00020 return -1; 00021 } 00022 } 00023 00024 // -1 if unsuccessful, else number of bytes written 00025 int UDPSocket::sendTo(Endpoint &remote, char *packet, int length) 00026 { 00027 if (!ip->open(remote.get_address(), remote.get_port(), IPStack::UDP)) { 00028 return -1; 00029 } 00030 00031 if (_blocking) { 00032 return ip->write(packet, length, -1); 00033 } else { 00034 return ip->write(packet, length, _timeout); 00035 } 00036 } 00037 00038 // -1 if unsuccessful, else number of bytes received 00039 int UDPSocket::receiveFrom(Endpoint &remote, char *buffer, int length) 00040 { 00041 if (!ip->open(remote.get_address(), remote.get_port(), IPStack::UDP)) { 00042 return -1; 00043 } 00044 00045 if (_blocking) { 00046 return ip->read(buffer, length, -1); 00047 } else { 00048 return ip->read(buffer, length, _timeout); 00049 } 00050 }
Generated on Thu Jul 14 2022 20:47:11 by
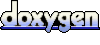