now this shit works
Fork of ESP8266NodeMCUInterface by
Embed:
(wiki syntax)
Show/hide line numbers
TCPSocketConnection.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #include "TCPSocketConnection.h" 00019 #include <cstring> 00020 #include <algorithm> 00021 00022 using std::memset; 00023 using std::memcpy; 00024 00025 //Debug is disabled by default 00026 #ifdef DEBUG 00027 #define DBG(x, ...) printf("[TCPConnection : DBG]"x"\r\n", ##__VA_ARGS__); 00028 #define WARN(x, ...) printf("[TCPConnection: WARN]"x"\r\n", ##__VA_ARGS__); 00029 #define ERR(x, ...) printf("[TCPConnection : ERR]"x"\r\n", ##__VA_ARGS__); 00030 #else 00031 #define DBG(x, ...) 00032 #define WARN(x, ...) 00033 #define ERR(x, ...) 00034 #endif 00035 00036 TCPSocketConnection::TCPSocketConnection() : 00037 _is_connected(false) 00038 { 00039 } 00040 00041 int TCPSocketConnection::connect(const char* host, const int port) 00042 { 00043 // if (init_socket(SOCK_STREAM) < 0) 00044 // return -1; 00045 // 00046 if (set_address(host, port) != 0) 00047 return -1; 00048 // 00049 // if (lwip_connect(_sock_fd, (const struct sockaddr *) &_remoteHost, sizeof(_remoteHost)) < 0) { 00050 // close(); 00051 // return -1; 00052 // } 00053 // _is_connected = true; 00054 _is_connected = ESP8266->open(ESP_TCP_TYPE,_ipAddress,_port); 00055 if(_is_connected) { //success 00056 return 0; 00057 } else { // fail 00058 return -1; 00059 } 00060 } 00061 00062 bool TCPSocketConnection::is_connected(void) 00063 { 00064 return _is_connected; 00065 } 00066 00067 int TCPSocketConnection::send(char* data, int length) 00068 { 00069 if (!_is_connected) { 00070 ERR("TCPSocketConnection::receive() - _is_connected is false : you cant receive data untill you connect to a socket!"); 00071 return -1; 00072 } 00073 Timer tmr; 00074 tmr.start(); 00075 while ((tmr.read_ms() < _timeout) || _blocking) { 00076 00077 if (wifi->send(data, length)) 00078 return length; 00079 } 00080 00081 return -1; 00082 00083 //return wifi->send(data,length); 00084 // 00085 // if (!_blocking) { 00086 // TimeInterval timeout(_timeout); 00087 // if (wait_writable(timeout) != 0) 00088 // return -1; 00089 // } 00090 // 00091 // int n = lwip_send(_sock_fd, data, length, 0); 00092 // _is_connected = (n != 0); 00093 // 00094 // return n; 00095 00096 } 00097 00098 // -1 if unsuccessful, else number of bytes written 00099 int TCPSocketConnection::send_all(char* data, int length) 00100 { 00101 // if ((_sock_fd < 0) || !_is_connected) 00102 // return -1; 00103 // 00104 // int writtenLen = 0; 00105 // TimeInterval timeout(_timeout); 00106 // while (writtenLen < length) { 00107 // if (!_blocking) { 00108 // // Wait for socket to be writeable 00109 // if (wait_writable(timeout) != 0) 00110 // return writtenLen; 00111 // } 00112 // 00113 // int ret = lwip_send(_sock_fd, data + writtenLen, length - writtenLen, 0); 00114 // if (ret > 0) { 00115 // writtenLen += ret; 00116 // continue; 00117 // } else if (ret == 0) { 00118 // _is_connected = false; 00119 // return writtenLen; 00120 // } else { 00121 // return -1; //Connnection error 00122 // } 00123 // } 00124 // return writtenLen; 00125 return send(data,length); // just remap to send 00126 } 00127 00128 int TCPSocketConnection::receive(char* buffer, int length) 00129 { 00130 if (!_is_connected) { 00131 ERR("TCPSocketConnection::receive() - _is_connected is false : you cant receive data untill you connect to a socket!"); 00132 return -1; 00133 } 00134 00135 while (wifi->readable() < length) 00136 ; 00137 00138 if (!wifi->recv(buffer, &length)) 00139 return -1; 00140 00141 return length; 00142 00143 00144 /* 00145 Timer tmr; 00146 int idx = 0; 00147 int nb_available = 0; 00148 int time = -1; 00149 00150 //make this the non-blocking case and return if <= 0 00151 // remember to change the config to blocking 00152 // if ( ! _blocking) { 00153 // if ( wifi.readable <= 0 ) { 00154 // return (wifi.readable); 00155 // } 00156 // } 00157 //--- 00158 tmr.start(); 00159 if (_blocking) { 00160 while (1) { 00161 nb_available = wifi->readable(); 00162 if (nb_available != 0) { 00163 break; 00164 } 00165 } 00166 } 00167 //--- 00168 // blocking case 00169 else { 00170 tmr.reset(); 00171 00172 while (time < _timeout) { 00173 nb_available = wifi->readable(); 00174 if (nb_available < 0) return nb_available; 00175 if (nb_available > 0) break ; 00176 time = tmr.read_ms(); 00177 } 00178 00179 if (nb_available == 0) return nb_available; 00180 } 00181 00182 // change this to < 20 mS timeout per byte to detect end of packet gap 00183 // this may not work due to buffering at the UART interface 00184 tmr.reset(); 00185 // while ( tmr.read_ms() < 20 ) { 00186 // if ( wifi.readable() && (idx < length) ) { 00187 // buffer[idx++] = wifi->getc(); 00188 // tmr.reset(); 00189 // } 00190 // if ( idx == length ) { 00191 // break; 00192 // } 00193 // } 00194 //--- 00195 while (time < _timeout) { 00196 00197 nb_available = wifi->readable(); 00198 //for (int i = 0; i < min(nb_available, length); i++) { 00199 for (int i = 0; i < min(nb_available, (length-idx)); i++) { 00200 buffer[idx] = wifi->getc(); 00201 idx++; 00202 } 00203 if (idx == length) { 00204 break; 00205 } 00206 time = tmr.read_ms(); 00207 } 00208 //--- 00209 return (idx == 0) ? -1 : idx; 00210 */ 00211 //************************ original code below 00212 // 00213 // if (!_blocking) { 00214 // TimeInterval timeout(_timeout); 00215 // if (wait_readable(timeout) != 0) 00216 // return -1; 00217 // } 00218 // 00219 // int n = lwip_recv(_sock_fd, data, length, 0); 00220 // _is_connected = (n != 0); 00221 // 00222 // return n; 00223 } 00224 00225 // -1 if unsuccessful, else number of bytes received 00226 int TCPSocketConnection::receive_all(char* data, int length) 00227 { 00228 // if ((_sock_fd < 0) || !_is_connected) 00229 // return -1; 00230 // 00231 // int readLen = 0; 00232 // TimeInterval timeout(_timeout); 00233 // while (readLen < length) { 00234 // if (!_blocking) { 00235 // //Wait for socket to be readable 00236 // if (wait_readable(timeout) != 0) 00237 // return readLen; 00238 // } 00239 // 00240 // int ret = lwip_recv(_sock_fd, data + readLen, length - readLen, 0); 00241 // if (ret > 0) { 00242 // readLen += ret; 00243 // } else if (ret == 0) { 00244 // _is_connected = false; 00245 // return readLen; 00246 // } else { 00247 // return -1; //Connnection error 00248 // } 00249 // } 00250 // return readLen; 00251 if (!wifi->recv(data, &length)) 00252 return -1; 00253 00254 return length; 00255 }
Generated on Thu Jul 14 2022 16:33:07 by
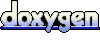