now this shit works
Fork of ESP8266NodeMCUInterface by
Embed:
(wiki syntax)
Show/hide line numbers
ESP8266.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 * @section DESCRIPTION 00019 * 00020 * ESP8266 serial wifi module 00021 * 00022 * Datasheet: 00023 * 00024 * http://www.electrodragon.com/w/Wi07c 00025 */ 00026 00027 #ifndef ESP8266_H 00028 #define ESP8266_H 00029 00030 #include "mbed.h" 00031 #include "CBuffer.h" 00032 #include "BufferedSerial.h" 00033 00034 #define ESP_TCP_TYPE 1 00035 #define ESP_UDP_TYPE 0 00036 #define ESP_MAX_LINE 62 00037 00038 /** 00039 * The ESP8266 class 00040 */ 00041 class ESP8266 00042 { 00043 00044 public: 00045 /** 00046 * Constructor 00047 * 00048 * @param tx mbed pin to use for tx line of Serial interface 00049 * @param rx mbed pin to use for rx line of Serial interface 00050 * @param reset reset pin of the wifi module () 00051 * @param baud the baudrate of the serial connection 00052 * @param timeout the timeout of the serial connection 00053 */ 00054 ESP8266(PinName tx, PinName rx, PinName reset, int baud = 9600, int timeout = 3000); 00055 00056 /** 00057 * Initialize the wifi hardware 00058 * 00059 * @return true if successful 00060 */ 00061 bool init(); 00062 00063 /** 00064 * Connect the wifi module to the specified ssid. 00065 * 00066 * @param ssid ssid of the network 00067 * @param phrase WEP, WPA or WPA2 key 00068 * @return true if successful 00069 */ 00070 bool connect(const char *ssid, const char *phrase); 00071 00072 /** 00073 * Check connection to the access point 00074 * @return true if successful 00075 */ 00076 bool is_connected(); 00077 00078 /** 00079 * Disconnect the ESP8266 module from the access point 00080 * 00081 * @return true if successful 00082 */ 00083 bool disconnect(); 00084 00085 /* 00086 * Start up a UDP or TCP Connection 00087 * 00088 * @param type 0 for UDP, 1 for TCP 00089 * @param ip A string that contains the IP, no quotes 00090 * @param port Numerical port number to connect to 00091 * @param id number between 0-4, if defined it denotes ID to use in multimode (Default to Single connection mode with -1) 00092 * @return true if sucessful, 0 if fail 00093 */ 00094 bool open(bool type, char* ip, int port, int id = -1); 00095 00096 /** 00097 * Close a connection 00098 * 00099 * @return true if successful 00100 */ 00101 bool close(); 00102 00103 /** 00104 * Read a character or block 00105 * 00106 * @return the character read or -1 on error 00107 */ 00108 int getc(); 00109 00110 /** 00111 * Write a character 00112 * 00113 * @param the character which will be written 00114 * @return -1 on error 00115 */ 00116 int putc(char c); 00117 00118 /** 00119 * Write a string 00120 * 00121 * @param buffer the buffer that will be written 00122 * @param len the length of the buffer 00123 * @return true on success 00124 */ 00125 bool send(const char *buffer, int len); 00126 00127 /** 00128 * Read a string without blocking 00129 * 00130 * @param buffer the buffer that will be written 00131 * @param len the length of the buffer, is replaced by read length 00132 * @return true on success 00133 */ 00134 bool recv(char *buffer, int *len); 00135 00136 /** 00137 * Check if wifi is writable 00138 * 00139 * @return 1 if wifi is writable 00140 */ 00141 int writeable(); 00142 00143 /** 00144 * Check if wifi is readable 00145 * 00146 * @return number of characters available 00147 */ 00148 int readable(); 00149 00150 /** 00151 * Return the IP address 00152 * @return IP address as a string 00153 */ 00154 const char *getIPAddress(); 00155 00156 /** 00157 * Return the IP address from host name 00158 * @return true on success, false on failure 00159 */ 00160 bool getHostByName(const char *host, char *ip); 00161 00162 /** 00163 * Reset the wifi module 00164 */ 00165 bool reset(); 00166 00167 /** 00168 * Obtains the current instance of the ESP8266 00169 */ 00170 static ESP8266 *getInstance() { 00171 return _inst; 00172 }; 00173 00174 /** 00175 * Send part of a command to the wifi module. 00176 * 00177 * @param cmd string to be sent 00178 * @param len optional length of cmd 00179 * @param sanitize flag indicating if cmd is actually payload and needs to be escaped 00180 * @return true if successful 00181 */ 00182 bool command(const char *cmd); 00183 00184 private: 00185 /** 00186 * Read a character with timeout 00187 * 00188 * @return the character read or -1 on timeout 00189 */ 00190 int serialgetc(); 00191 00192 /** 00193 * Write a character 00194 * 00195 * @param the character which will be written 00196 * @return -1 on timeout 00197 */ 00198 int serialputc(char c); 00199 00200 /** 00201 * Discards echoed characters 00202 * 00203 * @return true if successful 00204 */ 00205 bool discardEcho(); 00206 00207 /** 00208 * Flushes to next prompt 00209 * 00210 * @return true if successful 00211 */ 00212 bool flush(); 00213 00214 00215 00216 /** 00217 * Execute the command sent by command 00218 * 00219 * @param resp_buf pointer to buffer to store response from the wifi module 00220 * @param resp_len len of buffer to store response from the wifi module, is replaced by read length 00221 * @return true if successful 00222 */ 00223 bool execute(char *resp_buffer = 0, int *resp_len = 0); 00224 bool execute_full_length(char *resp_buffer = 0, int *resp_len = 0); 00225 00226 protected: 00227 BufferedSerial _serial; 00228 DigitalOut _reset_pin; 00229 00230 static ESP8266 * _inst; 00231 00232 // TODO WISHLIST: ipv6? 00233 // this requires nodemcu support 00234 char _ip[16]; 00235 00236 int _baud; 00237 int _timeout; 00238 }; 00239 00240 #endif
Generated on Thu Jul 14 2022 16:33:07 by
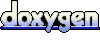