
Initial commit
Dependencies: ConfigFile FXOS8700CQ M2XStreamClient-JMF MODSERIAL SDFileSystem WNCInterface jsonlite mbed-rtos mbed
Fork of StarterKit_M2X_DevLab by
hts221_driver.cpp
00001 /* =================================================================== 00002 Copyright © 2016, AVNET Inc. 00003 00004 Licensed under the Apache License, Version 2.0 (the "License"); 00005 you may not use this file except in compliance with the License. 00006 You may obtain a copy of the License at 00007 00008 http://www.apache.org/licenses/LICENSE-2.0 00009 00010 Unless required by applicable law or agreed to in writing, 00011 software distributed under the License is distributed on an 00012 "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, 00013 either express or implied. See the License for the specific 00014 language governing permissions and limitations under the License. 00015 00016 ======================================================================== */ 00017 00018 #include "HTS221.h" 00019 00020 00021 // ------------------------------------------------------------------------------ 00022 //jmf -- define I2C pins and functions to read & write to I2C device 00023 00024 #include <string> 00025 #include "mbed.h" 00026 extern I2C i2c; 00027 00028 // Read a single unsigned char from addressToRead and return it as a unsigned char 00029 unsigned char HTS221::readRegister(unsigned char slaveAddress, unsigned char ToRead) 00030 { 00031 char data = ToRead; 00032 00033 //i2c.write(slaveAddress, &data, 1, 0); 00034 i2c.write(slaveAddress, &data, 1, 1); //by Stefan 00035 i2c.read(slaveAddress, &data, 1, 0); 00036 return data; 00037 } 00038 00039 // Writes a single unsigned char (dataToWrite) into regToWrite 00040 int HTS221::writeRegister(unsigned char slaveAddress, unsigned char regToWrite, unsigned char dataToWrite) 00041 { 00042 const char data[] = {regToWrite, dataToWrite}; 00043 00044 return i2c.write(slaveAddress,data,2,0); 00045 } 00046 00047 00048 //jmf end 00049 // ------------------------------------------------------------------------------ 00050 00051 //static inline int humidityReady(uint8_t data) { 00052 // return (data & 0x02); 00053 //} 00054 //static inline int temperatureReady(uint8_t data) { 00055 // return (data & 0x01); 00056 //} 00057 00058 00059 HTS221::HTS221(void) : _address(HTS221_ADDRESS) 00060 { 00061 _temperature = 0; 00062 _humidity = 0; 00063 } 00064 00065 00066 int HTS221::begin(void) 00067 { 00068 uint8_t data; 00069 00070 data = readRegister(_address, WHO_AM_I); 00071 if (data == WHO_AM_I_RETURN){ 00072 if (activate()){ 00073 storeCalibration(); 00074 return data; 00075 } 00076 } 00077 00078 return 0; 00079 } 00080 00081 int 00082 HTS221::storeCalibration(void) 00083 { 00084 uint8_t data; 00085 uint16_t tmp; 00086 00087 for (int reg=CALIB_START; reg<=CALIB_END; reg++) { 00088 if ((reg!=CALIB_START+8) && (reg!=CALIB_START+9) && (reg!=CALIB_START+4)) { 00089 00090 data = readRegister(HTS221_ADDRESS, reg); 00091 00092 switch (reg) { 00093 case CALIB_START: 00094 _h0_rH = data; 00095 break; 00096 case CALIB_START+1: 00097 _h1_rH = data; 00098 break; 00099 case CALIB_START+2: 00100 _T0_degC = data; 00101 break; 00102 case CALIB_START+3: 00103 _T1_degC = data; 00104 break; 00105 00106 case CALIB_START+5: 00107 tmp = _T0_degC; 00108 _T0_degC = (data&0x3)<<8; 00109 _T0_degC |= tmp; 00110 00111 tmp = _T1_degC; 00112 _T1_degC = ((data&0xC)>>2)<<8; 00113 _T1_degC |= tmp; 00114 break; 00115 case CALIB_START+6: 00116 _H0_T0 = data; 00117 break; 00118 case CALIB_START+7: 00119 _H0_T0 |= data<<8; 00120 break; 00121 case CALIB_START+0xA: 00122 _H1_T0 = data; 00123 break; 00124 case CALIB_START+0xB: 00125 _H1_T0 |= data <<8; 00126 break; 00127 case CALIB_START+0xC: 00128 _T0_OUT = data; 00129 break; 00130 case CALIB_START+0xD: 00131 _T0_OUT |= data << 8; 00132 break; 00133 case CALIB_START+0xE: 00134 _T1_OUT = data; 00135 break; 00136 case CALIB_START+0xF: 00137 _T1_OUT |= data << 8; 00138 break; 00139 00140 00141 case CALIB_START+8: 00142 case CALIB_START+9: 00143 case CALIB_START+4: 00144 //DO NOTHING 00145 break; 00146 00147 // to cover any possible error 00148 default: 00149 return false; 00150 } /* switch */ 00151 } /* if */ 00152 } /* for */ 00153 return true; 00154 } 00155 00156 00157 int 00158 HTS221::activate(void) 00159 { 00160 uint8_t data; 00161 00162 data = readRegister(_address, CTRL_REG1); 00163 data |= POWER_UP; 00164 data |= ODR0_SET; 00165 writeRegister(_address, CTRL_REG1, data); 00166 00167 return true; 00168 } 00169 00170 00171 int HTS221::deactivate(void) 00172 { 00173 uint8_t data; 00174 00175 data = readRegister(_address, CTRL_REG1); 00176 data &= ~POWER_UP; 00177 writeRegister(_address, CTRL_REG1, data); 00178 return true; 00179 } 00180 00181 00182 int 00183 HTS221::bduActivate(void) 00184 { 00185 uint8_t data; 00186 00187 data = readRegister(_address, CTRL_REG1); 00188 data |= BDU_SET; 00189 writeRegister(_address, CTRL_REG1, data); 00190 00191 return true; 00192 } 00193 00194 00195 int 00196 HTS221::bduDeactivate(void) 00197 { 00198 uint8_t data; 00199 00200 data = readRegister(_address, CTRL_REG1); 00201 data &= ~BDU_SET; 00202 writeRegister(_address, CTRL_REG1, data); 00203 return true; 00204 } 00205 00206 00207 int 00208 HTS221::readHumidity(void) 00209 { 00210 uint8_t data = 0; 00211 uint16_t h_out = 0; 00212 double h_temp = 0.0; 00213 double hum = 0.0; 00214 00215 data = readRegister(_address, STATUS_REG); 00216 00217 if (data & HUMIDITY_READY) { 00218 data = readRegister(_address, HUMIDITY_H_REG); 00219 h_out = data << 8; // MSB 00220 data = readRegister(_address, HUMIDITY_L_REG); 00221 h_out |= data; // LSB 00222 00223 // Decode Humidity 00224 hum = ((int16_t)(_h1_rH) - (int16_t)(_h0_rH))/2.0; // remove x2 multiple 00225 00226 // Calculate humidity in decimal of grade centigrades i.e. 15.0 = 150. 00227 h_temp = (((int16_t)h_out - (int16_t)_H0_T0) * hum) / ((int16_t)_H1_T0 - (int16_t)_H0_T0); 00228 hum = ((int16_t)_h0_rH) / 2.0; // remove x2 multiple 00229 _humidity = (int16_t)((hum + h_temp)); // provide signed % measurement unit 00230 } 00231 return _humidity; 00232 } 00233 00234 00235 00236 double 00237 HTS221::readTemperature(void) 00238 { 00239 uint8_t data = 0; 00240 uint16_t t_out = 0; 00241 double t_temp = 0.0; 00242 double deg = 0.0; 00243 00244 data = readRegister(_address, STATUS_REG); 00245 00246 if (data & TEMPERATURE_READY) { 00247 00248 data= readRegister(_address, TEMP_H_REG); 00249 t_out = data << 8; // MSB 00250 data = readRegister(_address, TEMP_L_REG); 00251 t_out |= data; // LSB 00252 00253 // Decode Temperature 00254 deg = ((int16_t)(_T1_degC) - (int16_t)(_T0_degC))/8.0; // remove x8 multiple 00255 00256 // Calculate Temperature in decimal of grade centigrades i.e. 15.0 = 150. 00257 t_temp = (((int16_t)t_out - (int16_t)_T0_OUT) * deg) / ((int16_t)_T1_OUT - (int16_t)_T0_OUT); 00258 deg = ((int16_t)_T0_degC) / 8.0; // remove x8 multiple 00259 _temperature = deg + t_temp; // provide signed celsius measurement unit 00260 } 00261 00262 return _temperature; 00263 }
Generated on Fri Jul 15 2022 01:40:15 by
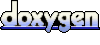