
HuyNQ
Dependencies: C12832 MQTT_MbedOS
Fork of mbed-os-mqtt by
main.cpp
00001 #include "mbed.h" 00002 #include "MQTTEthernet.h" 00003 #include "MQTTClient.h" 00004 00005 00006 // MQTT Variables 00007 char* MqttHostname = "192.168.1.105"; 00008 int MqttPort = 1883; 00009 char* MqttTopic = "mbed-sample-dssdsdhhuynq"; 00010 char* MqttClientId = "changeme-huynq-zcaxcaz"; 00011 00012 int main() 00013 { 00014 00015 printf("Example MQTT client\r\n"); 00016 00017 // Brings up the network interface 00018 MQTTEthernet eth = MQTTEthernet(); 00019 const char *ip = eth.get_ip_address(); 00020 00021 00022 printf("IP address is: %s\r\n", ip ? ip : "No IP"); 00023 00024 // Create Mbed Client Interface 00025 MQTT::Client<MQTTEthernet, Countdown> client = MQTT::Client<MQTTEthernet, Countdown>(eth); 00026 00027 // Create TCP connection 00028 eth.open(eth.getEth()); 00029 int rc = eth.connect(MqttHostname, MqttPort); 00030 printf("TCP Status: %s\r\n", (rc == 0) ? "Success" : "Failure"); 00031 00032 // Wait for a short length of time to allow user to see output messages. 00033 Thread::wait(2000); 00034 00035 if(rc == 0){ 00036 00037 00038 printf("Starting MQTT Client"); 00039 00040 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00041 data.MQTTVersion = 3; 00042 data.clientID.cstring = MqttClientId; 00043 rc = client.connect(data); 00044 00045 00046 printf("MQTT Client: %s\r\n", (rc == 0) ? "Connected" : "Failed"); 00047 00048 while(rc == 0){ 00049 MQTT::Message message; 00050 00051 // QoS 0 00052 char buf[100]; 00053 sprintf(buf, "Hello World!"); 00054 message.qos = MQTT::QOS0; 00055 message.retained = false; 00056 message.dup = false; 00057 message.payload = (void*)buf; 00058 message.payloadlen = strlen(buf)+1; 00059 rc = client.publish(MqttTopic, message); 00060 00061 Thread::wait(5000); 00062 00063 } 00064 } 00065 00066 // It is good practice to close the socket 00067 eth.disconnect(); 00068 } 00069
Generated on Thu Jul 21 2022 20:28:03 by
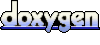