
Updated
Dependencies: C12832 LM75B PWM_Tone_Library mbed
Fork of IoT Project Final by
main.cpp
00001 #include "mbed.h" 00002 #include "C12832.h" 00003 #include "pwm_tone.h" 00004 00005 //DigitalOut myled(LED1); 00006 PwmOut Buzzer(D5); 00007 C12832 lcd(p5, p7, p6, p8, p11); 00008 AnalogIn sensor(p16); 00009 PwmOut spkr(p26); 00010 BusOut RGB (p23, p24, p25); 00011 00012 float C_3 = 1000000/Do3, 00013 Cs_3 = 1000000/Do3s, 00014 D_3 = 1000000/Re3, 00015 Ds_3 = 1000000/Re3s, 00016 E_3 = 1000000/Mi3, 00017 F_3 = 1000000/Fa3, 00018 Fs_3 = 1000000/Fa3s, 00019 G_3 = 1000000/So3, 00020 Gs_3 = 1000000/So3s, 00021 A_3 = 1000000/La3, 00022 As_3 = 1000000/La3s, 00023 B_3 = 1000000/Ti3, 00024 C_4 = 1000000/Do4, 00025 Cs_4 = 1000000/Do4s, 00026 D_4 = 1000000/Re4, 00027 Ds_4 = 1000000/Re4s, 00028 E_4 = 1000000/Mi4, 00029 F_4 = 1000000/Fa4, 00030 Fs_4 = 1000000/Fa4s, 00031 G_4 = 1000000/So4, 00032 Gs_4 = 1000000/So4s, 00033 A_4 = 1000000/La4, 00034 As_4 = 1000000/La4s, 00035 B_4 = 1000000/Ti4, 00036 C_5 = 1000000/Do5, 00037 Cs_5 = 1000000/Do5s, 00038 D_5 = 1000000/Re5, 00039 Ds_5 = 1000000/Re5s, 00040 E_5 = 1000000/Mi5, 00041 F_5 = 1000000/Fa5, 00042 Fs_5 = 1000000/Fa5s, 00043 G_5 = 1000000/So5, 00044 Gs_5 = 1000000/So5s, 00045 A_5 = 1000000/La5, 00046 As_5 = 1000000/La5s, 00047 B_5 = 1000000/Ti5; 00048 00049 int tones[] = {E_4, D_4, C_4, D_4, E_4, E_4, E_4, 0, D_4, D_4, D_4, 0, E_4, G_4, G_4, 0, 00050 E_4, D_4, C_4, D_4, E_4, E_4, E_4, 0, D_4, D_4, E_4, D_4, C_4, 0, 0, 0}; 00051 int tones_num = 32; 00052 00053 00054 float multiplier = 50; // this number got me closest to the reading on my multimeter temp probe 00055 float temp; // calculated temperature 00056 int count; // for computing average reading 00057 float total; 00058 float average; 00059 int MAX = 22; 00060 int MIN = 20; 00061 int Boiler_MAX = MIN + 2; 00062 00063 00064 void buzz() 00065 { 00066 for (float i=2000.0; i<7000.0; i+=1000) { 00067 spkr.period(0.5/i); 00068 spkr=0.1; 00069 wait(0.1); 00070 } 00071 spkr=0.0; 00072 } 00073 00074 int main() { 00075 count = 0; 00076 total = 0.0; 00077 // RGB = 0xFF; 00078 // buzz(); 00079 00080 while (1) { 00081 // formula is analog reading * multiplier 00082 00083 temp = sensor.read() * multiplier; 00084 count++; 00085 total += temp; 00086 average = total / count; 00087 00088 if(temp > MAX) 00089 { 00090 RGB = 0xff; 00091 buzz(); 00092 } 00093 else 00094 { 00095 RGB = 0x19; 00096 } 00097 00098 if(temp < MIN) 00099 { 00100 RGB = 0x06; 00101 } 00102 else 00103 { 00104 RGB = 0x19; 00105 } 00106 00107 lcd.cls(); 00108 lcd.locate(0,3); 00109 lcd.printf("Temperature= %6.2f \n Average= %5.1f \n", temp , average ); 00110 wait(1); 00111 } 00112 } 00113 00114 //int main(void) 00115 //{ 00116 // Tune(Buzzer, C_4, 4); //4 Octave C beat 4/16 00117 // wait_ms(250); 00118 // Tune(Buzzer, D_4, 4); //4 Octave D beat 4/16 00119 // wait_ms(250); 00120 // Tune(Buzzer, E_4, 4); //4 Octave E beat 4/16 00121 // wait_ms(250); 00122 // 00123 // int i; 00124 // 00125 // for(i=0; i<tones_num; i++) { 00126 // Auto_tunes(Buzzer, tones[i], 4); // Auto performance 00127 // Stop_tunes(Buzzer); 00128 // } 00129 // 00130 //} 00131 00132 00133 00134 00135
Generated on Sun Jul 31 2022 16:09:01 by
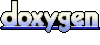