Input library for the STMstation P.1. Facilitates button state checking and battery voltage checking.
Embed:
(wiki syntax)
Show/hide line numbers
STMstation_input.cpp
00001 #include "STMstation_input.h" 00002 #include "mbed.h" 00003 00004 STMstation_input::STMstation_input(): D_UP(UP_PIN), D_DOWN(DOWN_PIN), D_LEFT(LEFT_PIN), D_RIGHT(RIGHT_PIN), 00005 D_A(A_PIN), D_B(B_PIN), D_X(X_PIN), D_Y(Y_PIN), 00006 D_START(START_PIN), D_SELECT(SELECT_PIN), bat(BAT_PIN) 00007 { 00008 } 00009 00010 void STMstation_input::updateButtons(){ 00011 bool oldState[10]; 00012 00013 for(int i=0; i<10; i++){ 00014 oldState[i] = buttonPress[i]; 00015 } 00016 buttonPress[0] = D_UP; 00017 buttonPress[1] = D_DOWN; 00018 buttonPress[2] = D_LEFT; 00019 buttonPress[3] = D_RIGHT; 00020 buttonPress[4] = D_A; 00021 buttonPress[5] = D_B; 00022 buttonPress[6] = D_X; 00023 buttonPress[7] = D_Y; 00024 buttonPress[8] = D_START; 00025 buttonPress[9] = D_SELECT; 00026 for(int i=0; i<10; i++){ 00027 if(oldState[i] == 0 & buttonPress[i] == 1){ 00028 buttonDown[i] = 1; 00029 buttonUp[i] = 0; 00030 } 00031 else if(oldState[i] == 1 & buttonPress[i] == 0){ 00032 buttonDown[i] = 0; 00033 buttonUp[i] = 1; 00034 } 00035 else{ 00036 buttonDown[i] = 0; 00037 buttonUp[i] = 0; 00038 } 00039 } 00040 } 00041 00042 bool STMstation_input::keyDown(Button b){ 00043 if(buttonDown[b] == 0){ 00044 return 0; 00045 } 00046 else{ 00047 return 1; 00048 } 00049 } 00050 00051 bool STMstation_input::keyUp(Button b){ 00052 if(buttonUp[b] == 0){ 00053 return 0; 00054 } 00055 else{ 00056 return 1; 00057 } 00058 } 00059 00060 bool STMstation_input::keyPress(Button b){ 00061 if(buttonPress[b] == 0){ 00062 return 0; 00063 } 00064 else{ 00065 return 1; 00066 } 00067 } 00068 00069 float STMstation_input::batCheck(){ 00070 float voltage = bat.read()*3.3f*159.0f/120; 00071 return voltage; 00072 }
Generated on Sat Jul 23 2022 20:05:01 by
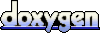