
A simple digital lock with attempted audio guidance
Dependencies: FATFileSystem TextLCD mbed
Fork of Digital_Lock_with_audio by
main.cpp
00001 /* Digital Lock System 00002 Components Used: 00003 NGX Baseboord 00004 MPR121 Capacitive touch panel as keypad( p26, p9, p10)// IRQ, SDA, SCL 00005 LCD for display(p24, p25, p27, p28, p29, p30) // rs, e, d4-d7 00006 Motor for opening/closing the lock(p11, p12) // for input in H Bridge 00007 Baseboard SD Card for storing Forest.wav 00008 Baseboard audio jack 3.5 mm 00009 00010 By using touch panel as input we enter the desired password to open the lock. 00011 Initially we store a password in array ORG[] which can be compared with the password(length= 7)entered by the user. 00012 00013 If the entered password is correct then motor opens the door. 00014 In case of wrong password the user is asked to re-enter the password. 3 trials are given after which door is permanently 00015 locked. 00016 Option to change the password is also provided. 00017 The process is guided by display of instruction on the LCD. 00018 00019 00020 Under Progress: 00021 An attempt is being made to guide the user by playing relavent audio files along with the instructions on LCD. 00022 The "forest.wav" file is the one that we are attempting to play along with LCD instructions. 00023 the bit rate of the wave file is 176kbps. 00024 */ 00025 00026 00027 00028 #include "mbed.h" 00029 #include "SDFileSystem.h" 00030 #include <string> 00031 #include <list> 00032 #include <mpr121.h> 00033 #include "TextLCD.h" 00034 #include <stdio.h> 00035 #include <stdlib.h> 00036 #include <sstream> 00037 #include <iostream> 00038 00039 00040 //------------------------------------------------------------------initialize lcd 00041 00042 TextLCD lcd(p24, p25, p27, p28, p29, p30); // rs, e, d4-d7 00043 //-------------------------------------------------------------------- 00044 00045 //------------------------------------------------------------------initial touch pannel 00046 DigitalOut led1(LED1); 00047 DigitalOut led2(LED2); 00048 DigitalOut led3(LED3); 00049 DigitalOut led4(LED4); 00050 DigitalOut servo(p12); 00051 int org[7] = {0,0,0,0,0,0,0}; 00052 int Nums[7] = {0,0,0,0,0,0,0}; 00053 int counter = 0; 00054 int count2 = 0; 00055 int k=0; 00056 DigitalOut servo1(p11); 00057 DigitalOut servo2(p12); 00058 //------------------------------------------- Create the interrupt receiver object on pin 26 00059 InterruptIn interrupt(p26); 00060 00061 00062 //-------------------------------------------------------- Setup the i2c bus on pins 28 and 27 00063 I2C i2c(p9, p10); 00064 00065 00066 //------------------------------------------ constructor(i2c object, i2c address of the mpr121) 00067 Mpr121 mpr121(&i2c, Mpr121::ADD_VSS); 00068 00069 //-------------------------------------------------------------------touch panel initialised 00070 00071 00072 00073 //---------------------------------------------------------------------------------------------playing audio 00074 AnalogOut DACout(p18); 00075 //DigitalOut digout(LED4); 00076 Ticker tick; 00077 SDFileSystem sd(p5, p6, p7, p8, "sd"); // NGX mbed base board 00078 00079 #define SAMPLE_FREQ 40000 00080 #define BUF_SIZE (SAMPLE_FREQ/10) 00081 #define SLICE_BUF_SIZE 1 00082 00083 void dac_out(void); 00084 void play_wave(char *); 00085 void cleanup(char *); 00086 void fill_adc_buf(short *, unsigned); 00087 void swapword(unsigned *); 00088 00089 // a FIFO for the DAC 00090 short DAC_fifo[256]; 00091 short DAC_wptr; 00092 short DAC_rptr; 00093 short DAC_on; 00094 00095 typedef struct uFMT_STRUCT { 00096 short comp_code; 00097 short num_channels; 00098 unsigned sample_rate; 00099 unsigned avg_Bps; 00100 short block_align; 00101 short sig_bps; 00102 } FMT_STRUCT; 00103 00104 00105 00106 void play_wave(char *wavname) 00107 { 00108 unsigned chunk_id,chunk_size,channel; 00109 // unsigned *data_wptr,data,samp_int,i; 00110 unsigned data,samp_int,i; 00111 short dac_data; 00112 char *slice_buf; 00113 short *data_sptr; 00114 // char *data_bptr; 00115 FMT_STRUCT wav_format; 00116 FILE *wavfile; 00117 long slice,num_slices; 00118 DAC_wptr=0; 00119 DAC_rptr=0; 00120 for (i=0;i<256;i+=2) 00121 { 00122 DAC_fifo[i]=0; 00123 DAC_fifo[i+1]=3000; 00124 } 00125 DAC_wptr=4; 00126 DAC_on=0; 00127 00128 led1=led2=led3=led4=0; 00129 lcd.cls(); 00130 lcd.printf("Playing wave file '%s'\n",wavname); 00131 00132 wavfile=fopen(wavname,"rb"); 00133 if (!wavfile) { 00134 lcd.cls(); 00135 printf("Unable to open wav file '%s'\n",wavname); 00136 exit(1); 00137 } 00138 00139 fread(&chunk_id,4,1,wavfile); 00140 fread(&chunk_size,4,1,wavfile); 00141 while (!feof(wavfile)) { 00142 00143 00144 lcd.cls(); 00145 lcd.printf("Read chunk ID 0x%x, size 0x%x\n",chunk_id,chunk_size); 00146 switch (chunk_id) { 00147 case 0x46464952: 00148 fread(&data,4,1,wavfile); 00149 lcd.cls(); 00150 lcd.printf("RIFF chunk\n"); 00151 wait(1); 00152 lcd.cls(); 00153 lcd.printf(" chunk size %d (0x%x)\n",chunk_size,chunk_size); 00154 lcd.cls(); 00155 lcd.printf(" RIFF type 0x%x\n",data); 00156 break; 00157 case 0x20746d66: 00158 fread(&wav_format,sizeof(wav_format),1,wavfile); 00159 lcd.cls(); 00160 lcd.printf("FORMAT chunk\n"); 00161 wait(1); 00162 lcd.cls(); 00163 lcd.printf(" chunk size %d (0x%x)\n",chunk_size,chunk_size); 00164 wait(1); 00165 lcd.cls(); 00166 lcd.printf(" compression code %d\n",wav_format.comp_code); 00167 wait(1); 00168 lcd.cls(); 00169 lcd.printf(" %d channels\n",wav_format.num_channels); 00170 wait(1); 00171 lcd.cls(); 00172 lcd.printf(" %d samples/sec\n",wav_format.sample_rate); 00173 wait(1); 00174 lcd.cls(); 00175 lcd.printf(" %d bytes/sec\n",wav_format.avg_Bps); 00176 wait(1); 00177 lcd.cls(); 00178 lcd.printf(" block align %d\n",wav_format.block_align); 00179 wait(1); 00180 lcd.cls(); 00181 lcd.printf(" %d bits per sample\n",wav_format.sig_bps); 00182 if (chunk_size > sizeof(wav_format)) 00183 fseek(wavfile,chunk_size-sizeof(wav_format),SEEK_CUR); 00184 // create a slice buffer large enough to hold multiple slices 00185 slice_buf=(char *)malloc(wav_format.block_align*SLICE_BUF_SIZE); 00186 if (!slice_buf) { 00187 lcd.cls(); 00188 lcd.printf("Unable to malloc slice buffer"); 00189 exit(1); 00190 } 00191 break; 00192 case 0x61746164: 00193 slice_buf=(char *)malloc(wav_format.block_align*SLICE_BUF_SIZE); 00194 if (!slice_buf) { 00195 lcd.cls(); 00196 lcd.printf("Unable to malloc slice buffer"); 00197 exit(1); 00198 } num_slices=chunk_size/wav_format.block_align; 00199 lcd.cls(); 00200 lcd.printf("DATA chunk\n"); 00201 lcd.cls(); 00202 lcd.printf(" chunk size %d (0x%x)\n",chunk_size,chunk_size); 00203 lcd.cls(); 00204 lcd.printf(" %d slices\n",num_slices); 00205 lcd.cls(); 00206 lcd.printf(" Ideal sample interval=%d\n",(unsigned)(1000000.0/wav_format.sample_rate)); 00207 samp_int=1000000/(wav_format.sample_rate); 00208 lcd.cls(); 00209 lcd.printf(" programmed interrupt tick interval=%d\n",samp_int); 00210 // starting up ticker to write samples out -- no printfs until tick.detach is called 00211 tick.attach_us(&dac_out, samp_int); 00212 DAC_on=1; 00213 led2=1; 00214 for (slice=0;slice<num_slices;slice+=SLICE_BUF_SIZE) { 00215 fread(slice_buf,wav_format.block_align*SLICE_BUF_SIZE,1,wavfile); 00216 if (feof(wavfile)) { 00217 lcd.cls(); 00218 lcd.printf("Oops -- not enough slices in the wave file\n"); 00219 exit(1); 00220 } 00221 data_sptr=(short *)slice_buf; 00222 for (i=0;i<SLICE_BUF_SIZE;i++) { 00223 dac_data=0; 00224 00225 // for a stereo wave file average the two channels. 00226 for (channel=0;channel<wav_format.num_channels;channel++) { 00227 switch (wav_format.sig_bps) { 00228 case 16: 00229 dac_data+=( ((int)(*data_sptr++)) +32768)>>5; 00230 break; 00231 } 00232 } 00233 dac_data>>=1; 00234 DAC_fifo[DAC_wptr]=dac_data; 00235 DAC_wptr=(DAC_wptr+1) & 0xff; 00236 while (DAC_wptr==DAC_rptr) { 00237 led1=1; 00238 } 00239 led1=0; 00240 } 00241 } 00242 DAC_on=0; 00243 led2=0; 00244 tick.detach(); 00245 lcd.cls(); 00246 lcd.printf("Ticker detached\n"); 00247 led3=1; 00248 free(slice_buf); 00249 break; 00250 case 0x5453494c: 00251 lcd.cls(); 00252 lcd.printf("INFO chunk, size %d\n",chunk_size); 00253 fseek(wavfile,chunk_size,SEEK_CUR); 00254 break; 00255 default: 00256 lcd.cls(); 00257 lcd.printf("unknown chunk type 0x%x, size %d\n",chunk_id,chunk_size); 00258 data=fseek(wavfile,chunk_size,SEEK_CUR); 00259 break; 00260 } 00261 fread(&chunk_id,4,1,wavfile); 00262 fread(&chunk_size,4,1,wavfile); 00263 } 00264 lcd.cls(); 00265 lcd.printf("Done with wave file\n"); 00266 fclose(wavfile); 00267 led1=0; 00268 } 00269 00270 00271 void dac_out() 00272 { 00273 if (DAC_on) { 00274 led4=1; 00275 DACout.write_u16(DAC_fifo[DAC_rptr]); 00276 DAC_rptr=(DAC_rptr+1) & 0xff; 00277 led4=0; 00278 } 00279 } 00280 00281 00282 00283 00284 //--------------------------------------------------------------------------------------------- 00285 00286 //-------------------------------------------------------------------------------obtaining input 00287 00288 00289 void fallInterrupt() { 00290 int key_code=0; 00291 int i=0; 00292 int value=mpr121.read(0x00); 00293 value +=mpr121.read(0x01)<<8; 00294 00295 i=0; 00296 // puts key number out to LEDs for demo 00297 for (i=0; i<12; i++) 00298 { 00299 if (((value>>i)&0x01)==1) 00300 { 00301 if(counter==0) 00302 { 00303 lcd.cls(); 00304 lcd.printf(""); 00305 } 00306 key_code=i; 00307 lcd.printf("%d", i); 00308 if(counter < 7) 00309 { 00310 00311 Nums[counter] = i; 00312 counter++; 00313 } 00314 } 00315 } 00316 led4=key_code & 0x01; 00317 led3=(key_code>>1) & 0x01; 00318 led2=(key_code>>2) & 0x01; 00319 led1=(key_code>>3) & 0x01; 00320 } 00321 00322 00323 //------------------------------------------------------------------------------MAIN 00324 00325 00326 int main() 00327 { 00328 00329 //------------------------------------------------to start enter new password which user wants to set 00330 lcd.cls(); 00331 lcd.printf("enter your new password\n"); 00332 00333 //play_wave("/sd/forest.wav"); 00334 wait(0.5); 00335 00336 int k; 00337 //----------------------------------------------enter password 00338 while(1) 00339 { 00340 interrupt.fall(&fallInterrupt); 00341 interrupt.mode(PullUp); 00342 if(counter==7){ 00343 break;} 00344 } 00345 00346 00347 for (k=0; k<7; k++) 00348 { 00349 org[k]=Nums[k]; 00350 } 00351 00352 wait(1); 00353 00354 AGAIN: 00355 lcd.cls(); 00356 lcd.printf("1-open door\n2-reset password"); 00357 play_wave("/sd/forest.wav"); 00358 interrupt.fall(&fallInterrupt); 00359 interrupt.mode(PullUp); 00360 00361 while (1) 00362 { 00363 counter=0; 00364 if(Nums[0]==1) 00365 { 00366 lcd.cls(); 00367 //---------------------------------------------------enter the password 00368 lcd.printf("enter your password\n"); 00369 play_wave("/sd/forest.wav"); 00370 wait(1); 00371 00372 while(1) 00373 { 00374 interrupt.fall(&fallInterrupt); 00375 interrupt.mode(PullUp); 00376 if(counter==7){break;} 00377 } 00378 if(counter == 7) 00379 { 00380 wait(1); 00381 //lcd.printf("%d",Nums[4]); 00382 for(k=0;k<7;k++) 00383 { 00384 lcd.printf("%d",Nums[k]); 00385 wait(1); 00386 } 00387 if((Nums[0] == org[0]) && (Nums[1] == org[1]) && (Nums[2] == org[2])&&(Nums[3] == org[3]) && (Nums[4] == org[4]) &&(Nums[5] == org[5])&& (Nums[6] == org[6])) 00388 { 00389 lcd.cls(); 00390 lcd.printf("password is correct, door open\n"); 00391 servo=1; 00392 wait(1); 00393 lcd.cls(); 00394 lcd.printf("opening door...\n"); 00395 play_wave("/sd/forest.wav"); 00396 servo1 = 1; 00397 servo2 = 0; 00398 wait(5); 00399 servo1 = 0; 00400 servo2 = 1; 00401 wait(5); 00402 goto AGAIN; 00403 } 00404 else 00405 { 00406 00407 lcd.cls(); 00408 lcd.printf("password incorrect.retry\n"); 00409 play_wave("/sd/forest.wav"); 00410 wait(10); 00411 goto AGAIN; 00412 } 00413 } 00414 } 00415 00416 00417 wait(1); 00418 if(Nums[0]==2) 00419 { 00420 wait(1); 00421 counter=0; 00422 lcd.cls(); 00423 lcd.printf("enter your old password\n"); 00424 play_wave("/sd/forest.wav"); 00425 while(1) 00426 { 00427 interrupt.fall(&fallInterrupt); 00428 interrupt.mode(PullUp); 00429 if(counter==7){break;} 00430 } 00431 00432 if(counter == 7) 00433 { 00434 00435 if((Nums[0] == org[0]) && (Nums[1] == org[1]) && (Nums[2] == org[2])&&(Nums[3] == org[3]) && (Nums[4] == org[4]) &&(Nums[5] == org[5])&& (Nums[6] == org[6])) 00436 { 00437 counter=0; 00438 lcd.cls(); 00439 lcd.printf("enter your new password"); 00440 play_wave("/sd/forest.wav"); 00441 while(1) 00442 { 00443 interrupt.fall(&fallInterrupt); 00444 interrupt.mode(PullUp); 00445 if(counter==7){break;} 00446 } 00447 00448 for (k=0; k<7; k++) 00449 { 00450 org[k]=Nums[k]; 00451 } 00452 00453 lcd.cls(); 00454 lcd.printf("password changed"); 00455 play_wave("/sd/forest.wav"); 00456 goto AGAIN; 00457 } 00458 else 00459 { 00460 lcd.cls(); 00461 lcd.printf("password incorrect.retry\n"); 00462 k++; 00463 if(k==3) 00464 { 00465 lcd.cls(); 00466 lcd.printf("Locked"); 00467 wait(30); 00468 } 00469 play_wave("/sd/forest.wav"); 00470 goto AGAIN; 00471 } 00472 } 00473 } 00474 else 00475 { 00476 goto AGAIN; 00477 } 00478 } 00479 }
Generated on Thu Jul 14 2022 14:59:21 by
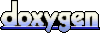