
BlueUSBをつかってみた
Dependencies: FatFileSystem mbed
Fork of PS3_BlueUSB by
main.cpp
00001 /* 00002 Copyright (c) 2010 Peter Barrett 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #include "mbed.h" 00024 //#include "rtos.h" 00025 00026 #include "USBHost.h" 00027 #include "Utils.h" 00028 #include "FATFileSystem.h" 00029 00030 00031 int MassStorage_ReadCapacity(int device, u32* blockCount, u32* blockSize); 00032 int MassStorage_Read(int device, u32 blockAddr, u32 blockCount, u8* dst, u32 blockSize); 00033 int MassStorage_Write(int device, u32 blockAddr, u32 blockCount, u8* dst, u32 blockSize); 00034 00035 class USBFileSystem : public FATFileSystem 00036 { 00037 int _device; 00038 u32 _blockSize; 00039 u32 _blockCount; 00040 00041 public: 00042 USBFileSystem() : FATFileSystem("usb"),_device(0),_blockSize(0),_blockCount(0) 00043 { 00044 } 00045 00046 void SetDevice(int device) 00047 { 00048 _device = device; 00049 } 00050 00051 virtual int disk_initialize() 00052 { 00053 return MassStorage_ReadCapacity(_device,&_blockCount,&_blockSize); 00054 } 00055 00056 virtual int disk_write(const char *buffer, int block_number) 00057 { 00058 return MassStorage_Write(_device,block_number,1,(u8*)buffer,_blockSize); 00059 } 00060 00061 virtual int disk_read(char *buffer, int block_number) 00062 { 00063 return MassStorage_Read(_device,block_number,1,(u8*)buffer,_blockSize); 00064 } 00065 00066 virtual int disk_sectors() 00067 { 00068 return _blockCount; 00069 } 00070 }; 00071 00072 void DumpFS(int depth, int count) 00073 { 00074 DIR *d = opendir("/usb"); 00075 if (!d) 00076 { 00077 printf("USB file system borked\r\n"); 00078 return; 00079 } 00080 00081 printf("\nDumping root dir\r\n"); 00082 struct dirent *p; 00083 for(;;) 00084 { 00085 p = readdir(d); 00086 if (!p) 00087 break; 00088 int len = sizeof( dirent); 00089 printf("%s %d\n", p->d_name, len); 00090 } 00091 closedir(d); 00092 } 00093 00094 int OnDiskInsert(int device) 00095 { 00096 USBFileSystem fs; 00097 fs.SetDevice(device); 00098 DumpFS(0,0); 00099 return 0; 00100 } 00101 00102 /* 00103 Simple test shell to exercise mouse,keyboard,mass storage and hubs. 00104 Add 2 15k pulldown resistors between D+/D- and ground, attach a usb socket and have at it. 00105 */ 00106 00107 Serial pc(USBTX, USBRX); 00108 int GetConsoleChar() 00109 { 00110 if (!pc.readable()) 00111 return -1; 00112 char c = pc.getc(); 00113 pc.putc(c); // echo 00114 return c; 00115 } 00116 00117 void TestShell(); 00118 00119 00120 int main() 00121 { 00122 00123 pc.baud(230400); 00124 printf("BlueUSB\nNow get a bunch of usb or bluetooth things and plug them in\r\n"); 00125 TestShell(); 00126 00127 }
Generated on Wed Jul 13 2022 12:19:22 by
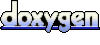