
BlueUSBをつかってみた
Dependencies: FatFileSystem mbed
Fork of PS3_BlueUSB by
TestShell.cpp
00001 00002 /* 00003 Copyright (c) 2010 Peter Barrett 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 /* 00025 Tue Apr 26 2011 Bart Janssens: added PS3 Bluetooth support 00026 */ 00027 00028 #include <stdio.h> 00029 #include <stdlib.h> 00030 #include <string.h> 00031 00032 #include "Utils.h" 00033 #include "USBHost.h" 00034 #include "hci.h" 00035 #include "ps3.h" 00036 00037 #include "mbed.h" 00038 DigitalOut LEDa(LED1); 00039 PwmOut LEDb(LED2); 00040 PwmOut LEDc(LED3); 00041 Serial Dev(p13,p14); 00042 00043 00044 void printf(const BD_ADDR* addr) 00045 { 00046 const u8* a = addr->addr; 00047 printf("%02X:%02X:%02X:%02X:%02X:%02X",a[5],a[4],a[3],a[2],a[1],a[0]); 00048 } 00049 00050 #define MAX_HCL_SIZE 260 00051 #define MAX_ACL_SIZE 400 00052 00053 class HCITransportUSB : public HCITransport 00054 { 00055 int _device; 00056 u8* _hciBuffer; 00057 u8* _aclBuffer; 00058 00059 public: 00060 void Open(int device, u8* hciBuffer, u8* aclBuffer) 00061 { 00062 _device = device; 00063 _hciBuffer = hciBuffer; 00064 _aclBuffer = aclBuffer; 00065 USBInterruptTransfer(_device,0x81,_hciBuffer,MAX_HCL_SIZE,HciCallback,this); 00066 USBBulkTransfer(_device,0x82,_aclBuffer,MAX_ACL_SIZE,AclCallback,this); 00067 //Dev.baud(115200); 00068 00069 } 00070 00071 static void HciCallback(int device, int endpoint, int status, u8* data, int len, void* userData) 00072 { 00073 HCI* t = ((HCITransportUSB*)userData)->_target; 00074 if (t) 00075 t->HCIRecv(data,len); 00076 USBInterruptTransfer(device,0x81,data,MAX_HCL_SIZE,HciCallback,userData); 00077 } 00078 00079 static void AclCallback(int device, int endpoint, int status, u8* data, int len, void* userData) 00080 { 00081 HCI* t = ((HCITransportUSB*)userData)->_target; 00082 if (t) 00083 t->ACLRecv(data,len); 00084 USBBulkTransfer(device,0x82,data,MAX_ACL_SIZE,AclCallback,userData); 00085 } 00086 00087 virtual void HCISend(const u8* data, int len) 00088 { 00089 USBControlTransfer(_device,REQUEST_TYPE_CLASS, 0, 0, 0,(u8*)data,len); 00090 } 00091 00092 virtual void ACLSend(const u8* data, int len) 00093 { 00094 USBBulkTransfer(_device,0x02,(u8*)data,len); 00095 } 00096 }; 00097 00098 00099 #define WII_REMOTE 0x042500 00100 #define PS3_REMOTE 0x080500 00101 char DATA[30]; 00102 class HIDBluetooth 00103 { 00104 int _control; // Sockets for control (out) and interrupt (in) 00105 int _interrupt; 00106 int _devClass; 00107 BD_ADDR _addr; 00108 u8 _pad[2]; // Struct align 00109 int _ready; 00110 Timeout _timeout; 00111 int _count; 00112 00113 public: 00114 HIDBluetooth() : _control(0),_interrupt(0),_devClass(0), _ready(1) {Dev.baud(921600);}; 00115 00116 00117 bool InUse() 00118 { 00119 return _control != 0; 00120 } 00121 00122 void attimeout() 00123 { 00124 printf("Timeout reached\r\n"); 00125 } 00126 00127 static void OnHidInterrupt(int socket, SocketState state,const u8* data, int len, void* userData) 00128 { 00129 HIDBluetooth* t = (HIDBluetooth*)userData; 00130 t->_ready = 0; 00131 if (data) 00132 { 00133 //printf("devClass = %06X \r\n",t->_devClass); 00134 if (t->_devClass == WII_REMOTE && data[1] == 0x30) 00135 { 00136 printf("================wii====================\r\n"); 00137 t->WIILed(); 00138 t->WIIHid(); // ask for accelerometer 00139 t->_devClass = 0; 00140 00141 00142 const u8* d = data; 00143 switch (d[1]) 00144 { 00145 case 0x02: 00146 { 00147 int x = (signed char)d[3]; 00148 int y = (signed char)d[4]; 00149 printf("Mouse %2X dx:%d dy:%d\r\n",d[2],x,y); 00150 } 00151 break; 00152 00153 case 0x37: // Accelerometer http://wiki.wiimoteproject.com/Reports 00154 { 00155 int pad = (d[2] & 0x9F) | ((d[3] & 0x9F) << 8); 00156 int x = (d[2] & 0x60) >> 5 | d[4] << 2; 00157 int y = (d[3] & 0x20) >> 4 | d[5] << 2; 00158 int z = (d[3] & 0x40) >> 5 | d[6] << 2; 00159 printf("WII %04X %d %d %d\r\n",pad,x,y,z); 00160 } 00161 break; 00162 default: 00163 printHex(data,len); 00164 } 00165 } 00166 if (t->_devClass == PS3_REMOTE) 00167 { 00168 00169 u16 ButtonState = ((ps3report*)(data + 1))->ButtonState; 00170 u8 x = (ButtonState&0xff00)>>8, x1 = ButtonState & 0xff ; 00171 DATA[0] = x ; 00172 DATA[1] = x1; 00173 DATA[2] = ((ps3report*)(data+1))->LeftStickX; 00174 DATA[3] = ((ps3report*)(data+1))->LeftStickY; 00175 DATA[4] = ((ps3report*)(data+1))->RightStickX; 00176 DATA[5] = ((ps3report*)(data+1))->RightStickY; 00177 DATA[6] = ((ps3report*)(data+1))->PressureUp; 00178 DATA[7] = ((ps3report*)(data+1))->PressureRight; 00179 DATA[8] = ((ps3report*)(data+1))->PressureDown; 00180 DATA[9] = ((ps3report*)(data+1))->PressureLeft; 00181 DATA[10] = ((ps3report*)(data+1))->PressureL2; 00182 DATA[11] = ((ps3report*)(data+1))->PressureR2; 00183 DATA[12] = ((ps3report*)(data+1))->PressureL1; 00184 DATA[13] = ((ps3report*)(data+1))->PressureR1; 00185 DATA[14] = ((ps3report*)(data+1))->PressureTriangle; 00186 DATA[15] = ((ps3report*)(data+1))->PressureCircle; 00187 DATA[16] = ((ps3report*)(data+1))->PressureCross; 00188 DATA[17] = ((ps3report*)(data+1))->PressureSquare; 00189 DATA[18] = ((ps3report*)(data+1))->PSButtonState; 00190 DATA[19] = (((ps3report*)(data+1))->AccelX&0xff00)>>8; 00191 DATA[20] = ((ps3report*)(data+1))->AccelX & 0xff; 00192 DATA[21] = (((ps3report*)(data+1))->AccelY&0xff00)>>8; 00193 DATA[22] = ((ps3report*)(data+1))->AccelY & 0xff; 00194 DATA[23] = (((ps3report*)(data+1))->AccelZ&0xff00)>>8; 00195 DATA[24] = ((ps3report*)(data+1))->AccelZ & 0xff; 00196 DATA[25] = (((ps3report*)(data+1))->GyroZ&0xff00)>>8; 00197 DATA[26] = ((ps3report*)(data+1))->GyroZ & 0xff; 00198 DATA[27] = ((ps3report*)(data+1))->Charge; 00199 DATA[28] = ((ps3report*)(data+1))->Power; 00200 DATA[29] = ((ps3report*)(data+1))->Connection; 00201 //Dev.putc(0xff); 00202 //wait(0.003); 00203 for(int i = 0;i<6;i++) 00204 { 00205 //if(i%2 == 1) 00206 wait(0.0003); 00207 Dev.putc(DATA[i]); 00208 printf(": %3d " , DATA[i]); 00209 } 00210 wait(0.001); 00211 printf("\n"); 00212 char flag=0; 00213 char Cis = ((ps3report*)(data+1))->RightStickY; 00214 float cis = LEDb = (float)(Cis)/255; 00215 char Cross = ((ps3report*)(data+1))->RightStickX; 00216 float cross = LEDc = (float)(Cross)/255; 00217 //printf("Cis , %f , %f\n", cis,cross); 00218 /*if (Cis >= 180) flag=1; 00219 else if (Cis <= 80) flag=2; 00220 else if (Cross >= 180) flag=11; 00221 else if (Cross <= 80) flag=12; 00222 else if (((ps3report*)(data+1))->LeftStickX >= 180) flag=21; 00223 else if (((ps3report*)(data+1))->LeftStickX <= 80) flag=22; 00224 else if (((ps3report*)(data+1))->LeftStickY >= 180) flag=23; 00225 else if (((ps3report*)(data+1))->LeftStickY <= 80) flag=24; 00226 else if (x & 0x01) flag=3; 00227 else if (x & 0x02) flag=4; 00228 else if (x & 0x04) flag=5; 00229 else if (x & 0x08) flag=6; 00230 else if (x & 16) flag=7; 00231 else if (x & 32) flag=8; 00232 else if (x & 64) flag=9; 00233 else if (x & 128) flag=10; 00234 else if (x1 & 0x01) flag=13; 00235 else if (x1 & 0x02) flag=14; 00236 else if (x1 & 0x04) flag=15; 00237 else if (x1 & 0x08) flag=16; 00238 else if (x1 & 16) flag=17; 00239 else if (x1 & 32) flag=18; 00240 else if (x1 & 64) flag=19; 00241 else if (x1 & 128) flag=20; 00242 Dev.putc(flag);*/ 00243 if(ButtonState & 0x0002) { //L3 00244 //L3が押された時の処理 00245 LEDa=1; 00246 } else { 00247 LEDa=0; 00248 //L3が離された時の処理 00249 } 00250 if(ButtonState & 0x0004) { //R3 00251 //R3が押された時の処理 00252 } else { 00253 //R3が離された時の処理 00254 } 00255 t->_count ++; 00256 if (t->_count == 25) t->_count = 1; 00257 //ParsePs3Result((data + 1), sizeof(ps3report),t->_count); 00258 } 00259 else { 00260 printf("Not yet implemented \r\n"); 00261 00262 } 00263 } 00264 00265 } 00266 00267 static void OnHidControl(int socket, SocketState state, const u8* data, int len, void* userData) 00268 { 00269 //HIDBluetooth* t = (HIDBluetooth*)userData; 00270 00271 //printf("OnHidControl\r\n"); 00272 00273 } 00274 00275 static void OnAcceptCtrlSocket(int socket, SocketState state, const u8* data, int len, void* userData) 00276 { 00277 HIDBluetooth* t = (HIDBluetooth*)userData; 00278 00279 t->_control = socket; 00280 00281 //printf("Ctrl Socket number = %d \r\n", socket); 00282 00283 Socket_Accept(socket,OnHidControl,userData); 00284 u8 enable[6] = { 00285 0x53, /* HIDP_TRANS_SET_REPORT | HIDP_DATA_RTYPE_FEATURE */ 00286 0xf4, 0x42, 0x03, 0x00, 0x00 }; 00287 Socket_Send(socket,enable,6); 00288 00289 00290 } 00291 00292 static void OnAcceptDataSocket(int socket, SocketState state, const u8* data, int len, void* userData) 00293 { 00294 HIDBluetooth* t = (HIDBluetooth*)userData; 00295 t->_interrupt = socket; 00296 00297 printf("OnAcceptDataSocket: Data Socket accept here \r\n"); 00298 printf("OnAcceptDataSocket: Data Socket number = %d \r\n", socket); 00299 00300 //printf("OnAcceptDataSocket: Ctrl Socket = %d Data Socket accept = %d \r\n", t->_control, t->_interrupt); 00301 00302 Socket_Accept(socket,OnHidInterrupt,userData); 00303 00304 //if (data) 00305 // printHex(data,len); 00306 } 00307 00308 void Open(BD_ADDR* bdAddr, inquiry_info* info) 00309 { 00310 printf("L2CAPAddr size %d\r\n",sizeof(L2CAPAddr)); 00311 _addr = *bdAddr; 00312 L2CAPAddr sockAddr; 00313 sockAddr.bdaddr = _addr; 00314 sockAddr.psm = L2CAP_PSM_HID_INTR; 00315 printf("Socket_Open size %d\r\n",sizeof(L2CAPAddr)); 00316 _interrupt = Socket_Open(SOCKET_L2CAP,&sockAddr.hdr,OnHidInterrupt,this); 00317 sockAddr.psm = L2CAP_PSM_HID_CNTL; 00318 _control = Socket_Open(SOCKET_L2CAP,&sockAddr.hdr,OnHidControl,this); 00319 00320 printfBytes("OPEN DEVICE CLASS",info->dev_class,3); 00321 _devClass = (info->dev_class[0] << 16) | (info->dev_class[1] << 8) | info->dev_class[2]; 00322 } 00323 00324 void Listen(BD_ADDR* bdAddr, inquiry_info* info) 00325 { 00326 int result; 00327 //printf("L2CAPAddr size %d\r\n",sizeof(L2CAPAddr)); 00328 _addr = *bdAddr; 00329 L2CAPAddr sockAddr; 00330 sockAddr.bdaddr = _addr; 00331 00332 _count = 1; 00333 _ready = 1; 00334 00335 // set a buffer for the led&rumble report 00336 u8 abuffer[37] = { 00337 0x52, /* HIDP_TRANS_SET_REPORT | HIDP_DATA_RTYPE_OUTPUT */ 00338 0x01, 00339 0x00, 0x00, 0x00, 0x00, 0x00, 00340 0x00, 0x00, 0x00, 0x00, 0x1E, 00341 0xff, 0x27, 0x10, 0x00, 0x32, 00342 0xff, 0x27, 0x10, 0x00, 0x32, 00343 0xff, 0x27, 0x10, 0x00, 0x32, 00344 0xff, 0x27, 0x10, 0x00, 0x32, 00345 0x00, 0x00, 0x00, 0x00, 0x00, 00346 }; 00347 memcpy(_ledrumble,abuffer,37); 00348 00349 result = Socket_Listen(SOCKET_L2CAP,L2CAP_PSM_HID_CNTL,OnAcceptCtrlSocket,this); 00350 printf("listen return code ctrl socket = %d \r\n", result); 00351 00352 00353 result = Socket_Listen(SOCKET_L2CAP,L2CAP_PSM_HID_INTR,OnAcceptDataSocket,this); 00354 printf("listen return code data socket = %d \r\n", result); 00355 00356 printfBytes("OPEN DEVICE CLASS",info->dev_class,3); 00357 _devClass = (info->dev_class[0] << 16) | (info->dev_class[1] << 8) | info->dev_class[2]; 00358 00359 while (_ready){ // wait till we receive data from PS3Hid 00360 USBLoop(); 00361 } 00362 USBLoop(); 00363 00364 00365 00366 } 00367 00368 void Close() 00369 { 00370 if (_control) 00371 Socket_Close(_control); 00372 if (_interrupt) 00373 Socket_Close(_interrupt); 00374 _control = _interrupt = 0; 00375 } 00376 00377 void WIILed(int id = 0x10) 00378 { 00379 u8 led[3] = {0x52, 0x11, id}; 00380 if (_control) 00381 Socket_Send(_control,led,3); 00382 } 00383 00384 void WIIHid(int report = 0x37) 00385 { 00386 u8 hid[4] = { 0x52, 0x12, 0x00, report }; 00387 if (_control != -1) 00388 Socket_Send(_control,hid,4); 00389 } 00390 00391 00392 00393 void Ps3Hid_Led(int i) 00394 { 00395 printf("Ps3Hid led %d\r\n",i); 00396 u8 ledpattern[7] = {0x02, 0x04, 0x08, 0x10, 0x12, 0x14, 0x18 }; 00397 u8 buf[37]; 00398 00399 if (i < 7) _ledrumble[11] = ledpattern[i]; 00400 memcpy(buf, _ledrumble, 37); 00401 00402 if (_control != -1) 00403 Socket_Send(_control,buf,37); 00404 wait_ms(0.1); 00405 } 00406 00407 void Ps3Hid_Rumble(u8 duration_right, u8 power_right, u8 duration_left, u8 power_left ) 00408 { 00409 printf("Ps3Hid rumble \r\n"); 00410 u8 buf[37]; 00411 00412 memcpy(buf, _ledrumble, 37); 00413 buf[3] = duration_right; 00414 buf[4] = power_right; 00415 buf[5] = duration_left; 00416 buf[6] = power_left; 00417 00418 if (_control != -1) 00419 Socket_Send(_control,buf,37); 00420 wait_ms(0.1); 00421 } 00422 00423 int CheckHID() 00424 { 00425 printf("CheckHID \r\n"); 00426 printf("Ctrl = %d Intr = %d \r\n", _control, _interrupt); 00427 if (_control < 1) { 00428 printf("Ps3 not ready \r\n"); 00429 return 1; 00430 } else { 00431 printf("Ps3 ready %d \r\n",_control); 00432 return 0; 00433 } 00434 } 00435 private: 00436 u8 _ledrumble[37] ; 00437 }; 00438 00439 00440 HCI* gHCI = 0; 00441 00442 #define MAX_HID_DEVICES 8 00443 00444 int GetConsoleChar(); 00445 class ShellApp 00446 { 00447 char _line[64]; 00448 HIDBluetooth _hids[MAX_HID_DEVICES]; 00449 00450 public: 00451 void Ready() 00452 { 00453 printf("HIDBluetooth %d\r\n",sizeof(HIDBluetooth)); 00454 memset(_hids,0,sizeof(_hids)); 00455 //Inquiry(); 00456 Scan(); 00457 } 00458 00459 // We have connected to a device 00460 void ConnectionComplete(HCI* hci, connection_info* info) 00461 { 00462 printf("ConnectionComplete "); 00463 BD_ADDR* a = &info->bdaddr; 00464 printf(a); 00465 BTDevice* bt = hci->Find(a); 00466 HIDBluetooth* hid = NewHIDBluetooth(); 00467 printf("%08x %08x\r\n",bt,hid); 00468 if (hid) 00469 hid->Listen(a,&bt->_info); // use Listen for PS3, Open for WII 00470 hid->Ps3Hid_Led(0); // set led 1 00471 hid->Ps3Hid_Rumble(0x20,0xff,0x20,0xff); // rumble 00472 00473 } 00474 00475 HIDBluetooth* NewHIDBluetooth() 00476 { 00477 for (int i = 0; i < MAX_HID_DEVICES; i++) 00478 if (!_hids[i].InUse()) 00479 return _hids+i; 00480 return 0; 00481 } 00482 00483 void ConnectDevices() 00484 { 00485 BTDevice* devs[8]; 00486 int count = gHCI->GetDevices(devs,8); 00487 for (int i = 0; i < count; i++) 00488 { 00489 printfBytes("DEVICE CLASS",devs[i]->_info.dev_class,3); 00490 if (devs[i]->_handle == 0) 00491 { 00492 BD_ADDR* bd = &devs[i]->_info.bdaddr; 00493 printf("Connecting to "); 00494 printf(bd); 00495 printf("\r\n"); 00496 gHCI->CreateConnection(bd); 00497 } 00498 } 00499 } 00500 00501 const char* ReadLine() 00502 { 00503 int i; 00504 for (i = 0; i < 255; ) 00505 { 00506 USBLoop(); 00507 int c = GetConsoleChar(); 00508 if (c == -1) 00509 continue; 00510 if (c == '\n' || c == 13) 00511 break; 00512 _line[i++] = c; 00513 } 00514 _line[i] = 0; 00515 return _line; 00516 } 00517 00518 void Inquiry() 00519 { 00520 printf("Inquiry..\r\n"); 00521 gHCI->Inquiry(); 00522 } 00523 00524 void List() 00525 { 00526 #if 0 00527 printf("%d devices\r\n",_deviceCount); 00528 for (int i = 0; i < _deviceCount; i++) 00529 { 00530 printf(&_devices[i].info.bdaddr); 00531 printf("\r\n"); 00532 } 00533 #endif 00534 } 00535 00536 void Scan() 00537 { 00538 printf("Scanning...\r\n"); 00539 gHCI->WriteScanEnable(); 00540 } 00541 00542 void Connect() 00543 { 00544 ConnectDevices(); 00545 } 00546 00547 00548 void Disconnect() 00549 { 00550 gHCI->DisconnectAll(); 00551 } 00552 00553 void CloseMouse() 00554 { 00555 } 00556 00557 void Quit() 00558 { 00559 CloseMouse(); 00560 } 00561 00562 void Run() 00563 { 00564 for(;;) 00565 { 00566 const char* cmd = ReadLine(); 00567 if (strcmp(cmd,"scan") == 0 || strcmp(cmd,"inquiry") == 0) 00568 Inquiry(); 00569 else if (strcmp(cmd,"ls") == 0) 00570 List(); 00571 else if (strcmp(cmd,"connect") == 0) 00572 Connect(); 00573 else if (strcmp(cmd,"disconnect") == 0) 00574 Disconnect(); 00575 else if (strcmp(cmd,"q")== 0) 00576 { 00577 Quit(); 00578 break; 00579 } else { 00580 printf("eh? %s\r\n",cmd); 00581 } 00582 } 00583 } 00584 }; 00585 00586 // Instance 00587 ShellApp gApp; 00588 00589 static int HciCallback(HCI* hci, HCI_CALLBACK_EVENT evt, const u8* data, int len) 00590 { 00591 switch (evt) 00592 { 00593 case CALLBACK_READY: 00594 printf("CALLBACK_READY\r\n"); 00595 gApp.Ready(); 00596 break; 00597 00598 case CALLBACK_INQUIRY_RESULT: 00599 printf("CALLBACK_INQUIRY_RESULT "); 00600 printf((BD_ADDR*)data); 00601 printf("\r\n"); 00602 break; 00603 00604 case CALLBACK_INQUIRY_DONE: 00605 printf("CALLBACK_INQUIRY_DONE\r\n"); 00606 gApp.ConnectDevices(); 00607 break; 00608 00609 case CALLBACK_REMOTE_NAME: 00610 { 00611 BD_ADDR* addr = (BD_ADDR*)data; 00612 const char* name = (const char*)(data + 6); 00613 printf(addr); 00614 printf(" % s\r\n",name); 00615 } 00616 break; 00617 00618 case CALLBACK_CONNECTION_COMPLETE: 00619 gApp.ConnectionComplete(hci,(connection_info*)data); 00620 break; 00621 }; 00622 return 0; 00623 } 00624 00625 // these should be placed in the DMA SRAM 00626 typedef struct 00627 { 00628 u8 _hciBuffer[MAX_HCL_SIZE]; 00629 u8 _aclBuffer[MAX_ACL_SIZE]; 00630 } SRAMPlacement; 00631 00632 HCITransportUSB _HCITransportUSB; 00633 HCI _HCI; 00634 00635 u8* USBGetBuffer(u32* len); 00636 int OnBluetoothInsert(int device) 00637 { 00638 printf("Bluetooth inserted of %d\r\n",device); 00639 u32 sramLen; 00640 u8* sram = USBGetBuffer(&sramLen); 00641 sram = (u8*)(((u32)sram + 1023) & ~1023); 00642 SRAMPlacement* s = (SRAMPlacement*)sram; 00643 _HCITransportUSB.Open(device,s->_hciBuffer,s->_aclBuffer); 00644 _HCI.Open(&_HCITransportUSB,HciCallback); 00645 RegisterSocketHandler(SOCKET_L2CAP,&_HCI); 00646 gHCI = &_HCI; 00647 //gApp.Inquiry(); 00648 //gApp.Scan(); 00649 gApp.Connect(); 00650 return 0; 00651 } 00652 00653 void TestShell() 00654 { 00655 USBInit(); 00656 gApp.Run(); 00657 }
Generated on Wed Jul 13 2022 12:19:22 by
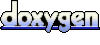