Motor2
Fork of Motor by
Embed:
(wiki syntax)
Show/hide line numbers
Motor.h
00001 00002 #ifndef Motor_H 00003 #define Motor_H 00004 00005 #include "mbed.h" 00006 #define Front 1 00007 #define Back 2 00008 #define Stop 3 00009 #define Free 4 00010 class Motor { 00011 protected : PinName Pin[3]; 00012 float bias; 00013 Motor(const Motor& m); 00014 Motor& operator=(const Motor &m) { 00015 return *this; 00016 } 00017 public: 00018 00019 Motor(PinName _pin1, PinName _pin2,PinName _pwm); 00020 00021 00022 Motor& operator= (float duty) 00023 { 00024 if(duty<-0.01F) 00025 { 00026 duty*=-1; 00027 run(Back,duty); 00028 } 00029 else if(float(duty)>0.01F) 00030 { 00031 run(Front,duty); 00032 } 00033 else run(Stop,0); 00034 return *this; 00035 } 00036 void run(int i,float duty); 00037 float min,max; 00038 void setbias_motor(float b){bias=b;} 00039 void duty_max(float Max){max=Max;} 00040 void duty_min(float Min){min=Min;} 00041 float getduty() 00042 { 00043 float a = PwmPin.read(); 00044 return a; 00045 } 00046 private: 00047 int state; 00048 float Duty; 00049 BusOut mode; 00050 PwmOut PwmPin; 00051 }; 00052 00053 #endif /* PID_H */
Generated on Fri Jul 15 2022 19:58:09 by
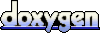