Read nRF51822(BLE Chip) VDD voltage and return 100 to 0% charging level
Dependents: BLE_Temp_Vdd_via_UART_TY BLE_EddystoneBeacon_w_ACC_TY51822 BLE_LoopbackUART_low_pwr_w_RTC1 BLE_Paired_Server ... more
nRF51_Vdd.h
00001 /* 00002 * mbed library program 00003 * Read nRF51822 VDD volatage and return 100 to 0% charging level 00004 * https://www.nordicsemi.com/eng/Products/Bluetooth-Smart-Bluetooth-low-energy/nRF51822 00005 * 00006 * Copyright (c) 2016,'18 Kenji Arai / JH1PJL 00007 * http://www.page.sannet.ne.jp/kenjia/index.html 00008 * https://os.mbed.com/users/kenjiArai/ 00009 * Created: January 23rd, 2016 00010 * Revised: April 14th, 2018 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00014 * AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #ifndef NRF51_VDD 00020 #define NRF51_VDD 00021 00022 #include "mbed.h" 00023 00024 #define ONLY4VDD 1 00025 #define USE_ADC 0 00026 00027 /** Read nRF51822 VDD volatage and return 0 to 100% charging level 00028 * 00029 * @code 00030 * #include "mbed.h" 00031 * #include "nRF51_Vdd.h" 00032 * 00033 * nRF51_Vdd vdd(3.6f, 1.8f); 00034 * // or follows if you don't use ADC function (more low current) 00035 * // nRF51_Vdd vdd(3.6f, 1.8f, ONLY4VDD); 00036 * 00037 * int main() { 00038 * uint8_t batteryLevel; 00039 * float batt; 00040 * 00041 * while(true){ 00042 * wait(1.0); 00043 * batteryLevel = vdd.read(); 00044 * batt = vdd.read_real_value(); 00045 * } 00046 * } 00047 * @endcode 00048 */ 00049 00050 class nRF51_Vdd 00051 { 00052 public: 00053 /** Configure data pin 00054 * @param 100% voltage reference value 00055 * @param 0% voltage reference value 00056 * @param LOW POWER MODE (none zero) Use PWR_DWN or USE_ADC 00057 */ 00058 nRF51_Vdd(float vdd100percent, float vddzeropercent, uint8_t adc_use); 00059 00060 /** Configure data pin 00061 * @param 100% voltage reference value 00062 * @param 0% voltage reference value 00063 */ 00064 nRF51_Vdd(float vdd100percent, float vddzeropercent); 00065 00066 /** Configure data pin (with other devices on I2C line) 00067 * @param none 00068 */ 00069 nRF51_Vdd(void); 00070 00071 /** Read Voltage value (percentage) 00072 * @param none 00073 * @return 0 to 100% 00074 */ 00075 uint8_t read(void); 00076 00077 /** Read Voltage value (real voltage) 00078 * @param none 00079 * @return real voltage (example 3.30V) 00080 */ 00081 float read_real_value(void); 00082 00083 private: 00084 float wrk_vdd, v100p, v0p; // working buffer 00085 uint32_t reg0,reg1,reg2; // Save current Configuration data 00086 uint32_t wrk; // save ADC(Vdd) data 00087 uint8_t use_adc_for_others; // save PWR_DWN or USE_ADC 00088 }; 00089 00090 #endif // NRF51_VDD
Generated on Thu Jul 14 2022 11:17:19 by
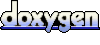