Read nRF51822(BLE Chip) VDD voltage and return 100 to 0% charging level
Dependents: BLE_Temp_Vdd_via_UART_TY BLE_EddystoneBeacon_w_ACC_TY51822 BLE_LoopbackUART_low_pwr_w_RTC1 BLE_Paired_Server ... more
nRF51_Vdd.cpp
00001 /* 00002 * mbed library program 00003 * Read nRF51822 VDD volatage and return 100 to 0% charging level 00004 * https://www.nordicsemi.com/eng/Products/Bluetooth-Smart-Bluetooth-low-energy/nRF51822 00005 * 00006 * Copyright (c) 2016,'18 Kenji Arai / JH1PJL 00007 * http://www.page.sannet.ne.jp/kenjia/index.html 00008 * https://os.mbed.com/users/kenjiArai/ 00009 * Created: January 23rd, 2016 00010 * Revised: April 14th, 2018 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00014 * AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "nRF51_Vdd.h" 00020 #include "nrf_delay.h" 00021 00022 nRF51_Vdd::nRF51_Vdd(float vdd100percent, float vddzeropercent, uint8_t adc_use){ 00023 v100p = vdd100percent; 00024 v0p = vddzeropercent; 00025 use_adc_for_others = adc_use; 00026 } 00027 00028 nRF51_Vdd::nRF51_Vdd(float vdd100percent, float vddzeropercent){ 00029 v100p = vdd100percent; 00030 v0p = vddzeropercent; 00031 use_adc_for_others = USE_ADC; 00032 } 00033 00034 nRF51_Vdd::nRF51_Vdd(void){ 00035 v100p = 3.3f; 00036 v0p = 1.8f; 00037 use_adc_for_others = USE_ADC; 00038 } 00039 00040 float nRF51_Vdd::read_real_value(void){ 00041 NRF_ADC->POWER = 1; 00042 nrf_delay_us(5); 00043 NRF_ADC->EVENTS_END = 0; 00044 NRF_ADC->TASKS_STOP = 1; 00045 reg0 = NRF_ADC->ENABLE; // save register value 00046 reg1 = NRF_ADC->CONFIG; // save register value 00047 reg2 = NRF_ADC->RESULT; // save register value 00048 NRF_ADC->ENABLE = ADC_ENABLE_ENABLE_Enabled; 00049 NRF_ADC->CONFIG = (ADC_CONFIG_RES_10bit << ADC_CONFIG_RES_Pos) | 00050 (ADC_CONFIG_INPSEL_SupplyOneThirdPrescaling << ADC_CONFIG_INPSEL_Pos) | 00051 (ADC_CONFIG_REFSEL_VBG << ADC_CONFIG_REFSEL_Pos) | 00052 (ADC_CONFIG_PSEL_Disabled << ADC_CONFIG_PSEL_Pos) | 00053 (ADC_CONFIG_EXTREFSEL_None << ADC_CONFIG_EXTREFSEL_Pos); 00054 NRF_ADC->EVENTS_END = 0; 00055 NRF_ADC->TASKS_START = 1; 00056 while (!NRF_ADC->EVENTS_END) {;} 00057 wrk = NRF_ADC->RESULT; // 10 bit result 00058 NRF_ADC->EVENTS_END = 0; 00059 NRF_ADC->TASKS_STOP = 1; 00060 if (use_adc_for_others == USE_ADC){ 00061 NRF_ADC->ENABLE = reg0; // recover register value 00062 NRF_ADC->CONFIG = reg1; // recover register value 00063 NRF_ADC->RESULT = reg2; // recover register value 00064 NRF_ADC->EVENTS_END = 0; 00065 } else { 00066 NRF_ADC->CONFIG = 0x18; 00067 NRF_ADC->EVENTS_END = 1; 00068 NRF_ADC->TASKS_STOP = 1; 00069 NRF_ADC->ENABLE = 0; 00070 nrf_delay_us(5); 00071 NRF_ADC->POWER = 0; 00072 nrf_delay_us(5); 00073 } 00074 return ((float)wrk / 1024.0f * 1.2f * 3.0f); 00075 } 00076 00077 uint8_t nRF51_Vdd::read(void){ 00078 wrk_vdd = read_real_value(); 00079 if (wrk_vdd <= v0p){ 00080 return 0; 00081 } else if (wrk_vdd >= v100p){ 00082 return 100; 00083 } 00084 wrk_vdd = (wrk_vdd - v0p) / (v100p - v0p); 00085 return (uint8_t)(wrk_vdd * 100); 00086 }
Generated on Thu Jul 14 2022 11:17:19 by
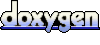