This is a RTC additional function. This is only for Nucleo F401RE & F411RE mbed(Added L152RE, F334R8, L476RG & F746xx). If you connected battery backup circuit for internal RTC, you can make a power-off and reset condition. RTC still has proper time and date.
Dependents: Nucleo_rtos_sample PB_Emma_Ethernet
SetRTC.cpp
00001 /* 00002 * mbed Library program 00003 * Check & set RTC function and set proper clock if we can set 00004 * ONLY FOR "Nucleo Board" 00005 * 00006 * Copyright (c) 2014,'15,'16 Kenji Arai / JH1PJL 00007 * http://www.page.sannet.ne.jp/kenjia/index.html 00008 * http://mbed.org/users/kenjiArai/ 00009 * Created: October 24th, 2014 00010 * Revised: July 2nd, 2016 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 * INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00014 * AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #if (defined(TARGET_STM32F401RE) || defined(TARGET_STM32F411RE) \ 00020 || defined(TARGET_STM32L152RE) || defined(TARGET_STM32F334R8) \ 00021 || defined(TARGET_STM32L476RG) \ 00022 || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG) ) 00023 00024 //#define DEBUG // use Communication with PC(UART) 00025 00026 // Include --------------------------------------------------------------------------------------- 00027 #include "mbed.h" 00028 #include "SetRTC.h" 00029 #if (defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG) ) 00030 #include "stm32f7xx_hal.h" 00031 #endif 00032 00033 // Definition ------------------------------------------------------------------------------------ 00034 #ifdef DEBUG 00035 #define BAUD(x) pcr.baud(x) 00036 #define GETC(x) pcr.getc(x) 00037 #define PUTC(x) pcr.putc(x) 00038 #define PRINTF(...) pcr.printf(__VA_ARGS__) 00039 #define READABLE(x) pcr.readable(x) 00040 #else 00041 #define BAUD(x) {;} 00042 #define GETC(x) {;} 00043 #define PUTC(x) {;} 00044 #define PRINTF(...) {;} 00045 #define READABLE(x) {;} 00046 #endif 00047 00048 // Object ---------------------------------------------------------------------------------------- 00049 Serial pcr(USBTX, USBRX); 00050 00051 // RAM ------------------------------------------------------------------------------------------- 00052 00053 // ROM / Constant data --------------------------------------------------------------------------- 00054 00055 // Function prototypes --------------------------------------------------------------------------- 00056 static int32_t set_RTC_LSI(void); 00057 static int32_t set_RTC_LSE(void); 00058 static int32_t rtc_external_osc_init(void); 00059 static uint32_t read_RTC_reg(uint32_t RTC_BKP_DR); 00060 static uint32_t check_RTC_backup_reg( void ); 00061 static int xatoi (char **str, unsigned long *res); 00062 static void get_line (char *buff, int len); 00063 static void set_5v_drop_detect(uint8_t function_use); 00064 00065 //------------------------------------------------------------------------------------------------- 00066 // Control Program 00067 //------------------------------------------------------------------------------------------------- 00068 int32_t SetRTC(uint8_t use_comparator) 00069 { 00070 if (rtc_external_osc_init() == 1) { 00071 set_5v_drop_detect(use_comparator); 00072 return 1; 00073 } else { 00074 return 0; 00075 } 00076 } 00077 00078 int32_t set_RTC_LSE(void) 00079 { 00080 uint32_t timeout = 0; 00081 00082 //---------------------------- LSE Configuration ------------------------- 00083 // Enable Power Clock 00084 __PWR_CLK_ENABLE(); 00085 // Enable write access to Backup domain 00086 #if (defined(TARGET_STM32L476RG) || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG)) 00087 PWR->CR1 |= PWR_CR1_DBP; 00088 #else 00089 PWR->CR |= PWR_CR_DBP; 00090 #endif 00091 // Wait for Backup domain Write protection disable 00092 timeout = HAL_GetTick() + DBP_TIMEOUT_VALUE; 00093 PRINTF("Time-Out %d\r\n", timeout); 00094 #if (defined(TARGET_STM32L476RG) || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG)) 00095 while((PWR->CR1 & PWR_CR1_DBP) == RESET) { 00096 #else 00097 while((PWR->CR & PWR_CR_DBP) == RESET) { 00098 #endif 00099 if(HAL_GetTick() >= timeout) { 00100 PRINTF("Time-Out 1\r\n"); 00101 return 0; 00102 } else { 00103 PRINTF("GetTick: %d\r\n",HAL_GetTick()); 00104 } 00105 } 00106 // Reset LSEON and LSEBYP bits before configuring the LSE ---------------- 00107 __HAL_RCC_LSE_CONFIG(RCC_LSE_OFF); 00108 // Get timeout 00109 timeout = HAL_GetTick() + TIMEOUT; 00110 PRINTF("Time-Out %d\r\n", timeout); 00111 // Wait till LSE is ready 00112 while(__HAL_RCC_GET_FLAG(RCC_FLAG_LSERDY) != RESET) { 00113 if(HAL_GetTick() >= timeout) { 00114 PRINTF("Time-Out 2\r\n"); 00115 return 0; 00116 } else { 00117 PRINTF("GetTick: %d\r\n",HAL_GetTick()); 00118 } 00119 } 00120 // Set the new LSE configuration ----------------------------------------- 00121 __HAL_RCC_LSE_CONFIG(RCC_LSE_ON); 00122 // Get timeout 00123 timeout = HAL_GetTick() + TIMEOUT; 00124 PRINTF("Time-Out %d\r\n", timeout); 00125 // Wait till LSE is ready 00126 #if (defined(TARGET_STM32F401RE) || defined(TARGET_STM32F411RE) \ 00127 || defined(TARGET_STM32F334R8) || defined(TARGET_STM32L476RG) \ 00128 || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG) ) 00129 while((RCC->BDCR & 0x02) != 2){ 00130 #elif defined(TARGET_STM32L152RE) 00131 while(__HAL_RCC_GET_FLAG(RCC_FLAG_LSERDY) == RESET) { 00132 #endif 00133 if(HAL_GetTick() >= timeout) { 00134 PRINTF("Time-Out 3\r\n"); 00135 return 0; 00136 } else { 00137 PRINTF("GetTick: %d\r\n",HAL_GetTick()); 00138 } 00139 } 00140 PRINTF("OK\r\n"); 00141 return 1; 00142 } 00143 00144 int32_t set_RTC_LSI(void) 00145 { 00146 uint32_t timeout = 0; 00147 00148 // Enable Power clock 00149 __PWR_CLK_ENABLE(); 00150 // Enable access to Backup domain 00151 HAL_PWR_EnableBkUpAccess(); 00152 // Reset Backup domain 00153 __HAL_RCC_BACKUPRESET_FORCE(); 00154 __HAL_RCC_BACKUPRESET_RELEASE(); 00155 // Enable Power Clock 00156 __PWR_CLK_ENABLE(); 00157 // Enable write access to Backup domain 00158 #if (defined(TARGET_STM32L476RG) || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG)) 00159 PWR->CR1 |= PWR_CR1_DBP; 00160 #else 00161 PWR->CR |= PWR_CR_DBP; 00162 #endif 00163 // Wait for Backup domain Write protection disable 00164 timeout = HAL_GetTick() + DBP_TIMEOUT_VALUE; 00165 #if (defined(TARGET_STM32L476RG) || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG)) 00166 while((PWR->CR1 & PWR_CR1_DBP) == RESET) { 00167 #else 00168 while((PWR->CR & PWR_CR_DBP) == RESET) { 00169 #endif 00170 if(HAL_GetTick() >= timeout) { 00171 return 0; 00172 } 00173 } 00174 __HAL_RCC_LSE_CONFIG(RCC_LSE_OFF); 00175 // Enable LSI 00176 __HAL_RCC_LSI_ENABLE(); 00177 timeout = HAL_GetTick() + TIMEOUT; 00178 // Wait till LSI is ready 00179 while(__HAL_RCC_GET_FLAG(RCC_FLAG_LSIRDY) == RESET) { 00180 if(HAL_GetTick() >= timeout) { 00181 return 0; 00182 } 00183 } 00184 // Connect LSI to RTC 00185 #if !(defined(TARGET_STM32F334R8) || defined(TARGET_STM32L476RG) \ 00186 || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG) ) 00187 __HAL_RCC_RTC_CLKPRESCALER(RCC_RTCCLKSOURCE_LSI); 00188 #endif 00189 __HAL_RCC_RTC_CONFIG(RCC_RTCCLKSOURCE_LSI); 00190 return 1; 00191 } 00192 00193 int32_t rtc_external_osc_init(void) 00194 { 00195 uint32_t timeout = 0; 00196 time_t seconds; 00197 uint8_t external_ok = 1; 00198 00199 // Enable Power clock 00200 __PWR_CLK_ENABLE(); 00201 // Enable access to Backup domain 00202 HAL_PWR_EnableBkUpAccess(); 00203 // Check backup condition 00204 if ( check_RTC_backup_reg() ) { 00205 #if (defined(TARGET_STM32F401RE) || defined(TARGET_STM32F411RE) \ 00206 || defined(TARGET_STM32F334R8) || defined(TARGET_STM32L476RG) \ 00207 || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG) ) 00208 if ((RCC->BDCR & 0x8307) == 0x8103){ 00209 #else 00210 if ((RCC->CSR & 0x430703) == 0x410300) { 00211 #endif 00212 //if (__HAL_RCC_GET_FLAG(RCC_FLAG_LSERDY) != RESET) { 00213 timeout = HAL_GetTick() + TIMEOUT / 5; 00214 seconds = time(NULL); 00215 while (seconds == time(NULL)){ 00216 if(HAL_GetTick() >= timeout) { 00217 PRINTF("Not available External Xtal\r\n"); 00218 external_ok = 0; 00219 break; 00220 } 00221 } 00222 if (external_ok){ 00223 PRINTF("OK everything\r\n"); 00224 return 1; 00225 } 00226 } 00227 } 00228 PRINTF("Reset RTC LSE config.\r\n"); 00229 // Reset Backup domain 00230 __HAL_RCC_BACKUPRESET_FORCE(); 00231 __HAL_RCC_BACKUPRESET_RELEASE(); 00232 // Enable LSE Oscillator 00233 if (set_RTC_LSE() == 1) { 00234 // Connect LSE to RTC 00235 #if !(defined(TARGET_STM32F334R8) || defined(TARGET_STM32L476RG) \ 00236 || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG) \ 00237 || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG) ) 00238 __HAL_RCC_RTC_CLKPRESCALER(RCC_RTCCLKSOURCE_LSE); 00239 #endif 00240 __HAL_RCC_RTC_CONFIG(RCC_RTCCLKSOURCE_LSE); 00241 PRINTF("Set LSE/External\r\n"); 00242 return 1; 00243 } else { 00244 set_RTC_LSI(); 00245 PRINTF("Set LSI/Internal\r\n"); 00246 return 0; 00247 } 00248 } 00249 00250 uint32_t read_RTC_reg(uint32_t RTC_BKP_DR) 00251 { 00252 __IO uint32_t tmp = 0; 00253 00254 // Check the parameters 00255 assert_param(IS_RTC_BKP(RTC_BKP_DR)); 00256 tmp = RTC_BASE + 0x50; 00257 tmp += (RTC_BKP_DR * 4); 00258 // Read the specified register 00259 return (*(__IO uint32_t *)tmp); 00260 } 00261 00262 // Check RTC Backup registers contents 00263 uint32_t check_RTC_backup_reg( void ) 00264 { 00265 if ( read_RTC_reg( RTC_BKP_DR0 ) == RTC_DAT0 ) { 00266 if ( read_RTC_reg( RTC_BKP_DR1 ) == RTC_DAT1 ) { 00267 return 1; 00268 } 00269 } 00270 return 0; 00271 } 00272 00273 void show_RTC_reg( void ) 00274 { 00275 // Show registers 00276 pcr.printf( "\r\nShow RTC registers\r\n" ); 00277 pcr.printf( " Reg0 =0x%08x, Reg1 =0x%08x\r\n", 00278 read_RTC_reg( RTC_BKP_DR0 ), 00279 read_RTC_reg( RTC_BKP_DR1 ) 00280 ); 00281 pcr.printf( " TR =0x..%06x, DR =0x..%06x, CR =0x..%06x\r\n", 00282 RTC->TR, RTC->DR, RTC->CR 00283 ); 00284 pcr.printf( " ISR =0x...%05x, PRER =0x..%06x, WUTR =0x....%04x\r\n", 00285 RTC->ISR, RTC->PRER, RTC->WUTR 00286 ); 00287 #if (defined(TARGET_STM32F401RE) || defined(TARGET_STM32F411RE) \ 00288 || defined(TARGET_STM32L152RE) ) 00289 pcr.printf( " CALIBR=0x....%04x, ALRMAR=0x%08x, ALRMBR=0x%08x\r\n", 00290 RTC->CALIBR, RTC->ALRMAR, RTC->ALRMBR 00291 ); 00292 #endif 00293 pcr.printf( 00294 " WPR =0x......%02x, SSR =0x....%04x, SHIFTR=0x....%04x\r\n", 00295 RTC->WPR, RTC->SSR, RTC->SHIFTR 00296 ); 00297 pcr.printf( 00298 " TSTR =0x..%06x, TSDR =0x....%04x, TSSSR =0x....%04x\r\n", 00299 RTC->TSTR, RTC->TSDR, RTC->TSSSR 00300 ); 00301 pcr.printf( "Show RCC registers (only RTC Related reg.)\r\n" ); 00302 #if (defined(TARGET_STM32F401RE) || defined(TARGET_STM32F411RE) \ 00303 || defined(TARGET_STM32F334R8) || defined(TARGET_STM32L476RG) \ 00304 || defined(TARGET_STM32F746NG) || defined(TARGET_STM32F746ZG) ) 00305 pcr.printf( " RCC_BDCR=0x...%05x\r\n", RCC->BDCR); 00306 #else 00307 pcr.printf( " RCC_CSR =0x%08x\r\n", RCC->CSR); 00308 #endif 00309 pcr.printf( "\r\n"); 00310 } 00311 00312 // Change string -> integer 00313 int xatoi (char **str, unsigned long *res) 00314 { 00315 unsigned long val; 00316 unsigned char c, radix, s = 0; 00317 00318 while ((c = **str) == ' ') (*str)++; 00319 if (c == '-') { 00320 s = 1; 00321 c = *(++(*str)); 00322 } 00323 if (c == '0') { 00324 c = *(++(*str)); 00325 if (c <= ' ') { 00326 *res = 0; 00327 return 1; 00328 } 00329 if (c == 'x') { 00330 radix = 16; 00331 c = *(++(*str)); 00332 } else { 00333 if (c == 'b') { 00334 radix = 2; 00335 c = *(++(*str)); 00336 } else { 00337 if ((c >= '0')&&(c <= '9')) { 00338 radix = 8; 00339 } else { 00340 return 0; 00341 } 00342 } 00343 } 00344 } else { 00345 if ((c < '1')||(c > '9')) { 00346 return 0; 00347 } 00348 radix = 10; 00349 } 00350 val = 0; 00351 while (c > ' ') { 00352 if (c >= 'a') c -= 0x20; 00353 c -= '0'; 00354 if (c >= 17) { 00355 c -= 7; 00356 if (c <= 9) return 0; 00357 } 00358 if (c >= radix) return 0; 00359 val = val * radix + c; 00360 c = *(++(*str)); 00361 } 00362 if (s) val = -val; 00363 *res = val; 00364 return 1; 00365 } 00366 00367 // Get key input data 00368 void get_line (char *buff, int len) 00369 { 00370 char c; 00371 int idx = 0; 00372 00373 for (;;) { 00374 c = pcr.getc(); 00375 if (c == '\r') { 00376 buff[idx++] = c; 00377 break; 00378 } 00379 if ((c == '\b') && idx) { 00380 idx--; 00381 pcr.putc(c); 00382 pcr.putc(' '); 00383 pcr.putc(c); 00384 } 00385 if (((uint8_t)c >= ' ') && (idx < len - 1)) { 00386 buff[idx++] = c; 00387 pcr.putc(c); 00388 } 00389 } 00390 buff[idx] = 0; 00391 pcr.putc('\n'); 00392 } 00393 00394 // RTC related subroutines 00395 void chk_and_set_time(char *ptr) 00396 { 00397 unsigned long p1; 00398 struct tm t; 00399 time_t seconds; 00400 00401 if (xatoi(&ptr, &p1)) { 00402 t.tm_year = (uint8_t)p1 + 100; 00403 PRINTF("Year:%d ",p1); 00404 xatoi( &ptr, &p1 ); 00405 t.tm_mon = (uint8_t)p1 - 1; 00406 PRINTF("Month:%d ",p1); 00407 xatoi( &ptr, &p1 ); 00408 t.tm_mday = (uint8_t)p1; 00409 PRINTF("Day:%d ",p1); 00410 xatoi( &ptr, &p1 ); 00411 t.tm_hour = (uint8_t)p1; 00412 PRINTF("Hour:%d ",p1); 00413 xatoi( &ptr, &p1 ); 00414 t.tm_min = (uint8_t)p1; 00415 PRINTF("Min:%d ",p1); 00416 xatoi( &ptr, &p1 ); 00417 t.tm_sec = (uint8_t)p1; 00418 PRINTF("Sec: %d \r\n",p1); 00419 } else { 00420 return; 00421 } 00422 seconds = mktime(&t); 00423 set_time(seconds); 00424 // Show Time with several example 00425 // ex.1 00426 pcr.printf( 00427 "Date: %04d/%02d/%02d, %02d:%02d:%02d\r\n", 00428 t.tm_year + 1900, t.tm_mon + 1, t.tm_mday, t.tm_hour, t.tm_min, t.tm_sec 00429 ); 00430 #if 0 00431 time_t seconds; 00432 char buf[40]; 00433 00434 seconds = mktime(&t); 00435 // ex.2 00436 strftime(buf, 40, "%x %X", localtime(&seconds)); 00437 pcr.printf("Date: %s\r\n", buf); 00438 // ex.3 00439 strftime(buf, 40, "%I:%M:%S %p (%Y/%m/%d)", localtime(&seconds)); 00440 pcr.printf("Date: %s\r\n", buf); 00441 // ex.4 00442 strftime(buf, 40, "%B %d,'%y, %H:%M:%S", localtime(&seconds)); 00443 pcr.printf("Date: %s\r\n", buf); 00444 #endif 00445 } 00446 00447 void time_enter_mode(void) 00448 { 00449 char *ptr; 00450 char linebuf[64]; 00451 00452 pcr.printf("\r\nSet time into RTC\r\n"); 00453 pcr.printf(" e.g. >16 5 28 10 11 12 -> May 28th, '16, 10:11:12\r\n"); 00454 pcr.printf(" If time is fine, just hit enter\r\n"); 00455 pcr.putc('>'); 00456 ptr = linebuf; 00457 get_line(ptr, sizeof(linebuf)); 00458 pcr.printf("\r"); 00459 chk_and_set_time(ptr); 00460 } 00461 00462 #if defined(TARGET_STM32L152RE) 00463 void goto_standby(void) 00464 { 00465 RCC->AHBENR |= (RCC_AHBENR_GPIOAEN | RCC_AHBENR_GPIOBEN | RCC_AHBENR_GPIOCEN | 00466 RCC_AHBENR_GPIODEN | RCC_AHBENR_GPIOHEN); 00467 #if 0 00468 GPIO_InitTypeDef GPIO_InitStruct; 00469 // All other ports are analog input mode 00470 GPIO_InitStruct.Pin = GPIO_PIN_All; 00471 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00472 GPIO_InitStruct.Pull = GPIO_NOPULL; 00473 GPIO_InitStruct.Speed = GPIO_SPEED_LOW; 00474 HAL_GPIO_Init(GPIOA, &GPIO_InitStruct); 00475 HAL_GPIO_Init(GPIOB, &GPIO_InitStruct); 00476 HAL_GPIO_Init(GPIOC, &GPIO_InitStruct); 00477 HAL_GPIO_Init(GPIOD, &GPIO_InitStruct); 00478 HAL_GPIO_Init(GPIOE, &GPIO_InitStruct); 00479 HAL_GPIO_Init(GPIOH, &GPIO_InitStruct); 00480 #else 00481 GPIOA->MODER = 0xffffffff; 00482 GPIOB->MODER = 0xffffffff; 00483 GPIOC->MODER = 0xffffffff; 00484 GPIOD->MODER = 0xffffffff; 00485 GPIOE->MODER = 0xffffffff; 00486 GPIOH->MODER = 0xffffffff; 00487 #endif 00488 RCC->AHBENR &= ~(RCC_AHBENR_GPIOAEN | RCC_AHBENR_GPIOBEN |RCC_AHBENR_GPIOCEN | 00489 RCC_AHBENR_GPIODEN | RCC_AHBENR_GPIOHEN); 00490 while(1) { 00491 #if 0 00492 // Stop mode 00493 HAL_PWR_EnterSTOPMode(PWR_LOWPOWERREGULATOR_ON, PWR_STOPENTRY_WFI); 00494 #else 00495 // Standby mode 00496 HAL_PWR_EnterSTANDBYMode(); 00497 #endif 00498 } 00499 } 00500 #else // defined(TARGET_STM32L152RE) 00501 void goto_standby(void) 00502 { 00503 deepsleep(); // Not Standby Mode but Deep Sleep Mode 00504 } 00505 #endif // defined(TARGET_STM32L152RE) 00506 00507 #if defined(TARGET_STM32L152RE) 00508 #if defined(USE_IRQ_FOR_RTC_BKUP) 00509 // COMP2 Interrupt routine 00510 void irq_comp2_handler(void) 00511 { 00512 __disable_irq(); 00513 goto_standby(); 00514 } 00515 00516 COMP_HandleTypeDef COMP_HandleStruct; 00517 GPIO_InitTypeDef GPIO_InitStruct; 00518 00519 // Set BOR level3 (2.54 to 2.74V) 00520 void set_BOR_level3(void) 00521 { 00522 FLASH_OBProgramInitTypeDef my_flash; 00523 00524 HAL_FLASHEx_OBGetConfig(&my_flash); // read current configuration 00525 if (my_flash.BORLevel != OB_BOR_LEVEL3) { 00526 my_flash.BORLevel = OB_BOR_LEVEL3; 00527 HAL_FLASHEx_OBProgram(&my_flash); 00528 } 00529 } 00530 00531 void set_5v_drop_detect(uint8_t function_use) 00532 { 00533 if (function_use == 0){ return;} 00534 set_BOR_level3(); 00535 // Set Analog voltage input (PB5 or PB6) 00536 #if defined(USE_PB5_FOR_COMP) 00537 GPIO_InitStruct.Pin = GPIO_PIN_5; // PB5 comp input 00538 #elif defined(USE_PB6_FOR_COMP) 00539 GPIO_InitStruct.Pin = GPIO_PIN_6; // PB6 comp input 00540 #else 00541 #error "Please define USE_PB5_FOR_COMP or USE_PB6_FOR_COMP in SetRTC.h" 00542 #endif 00543 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00544 GPIO_InitStruct.Pull = GPIO_NOPULL; 00545 GPIO_InitStruct.Speed = GPIO_SPEED_HIGH; 00546 HAL_GPIO_Init(GPIOB, &GPIO_InitStruct); 00547 // COMP2 sets for low volatage detection 00548 __COMP_CLK_ENABLE(); 00549 COMP_HandleStruct.Instance = COMP2; 00550 COMP_HandleStruct.Init.InvertingInput = COMP_INVERTINGINPUT_VREFINT; 00551 #if defined(USE_PB5_FOR_COMP) 00552 COMP_HandleStruct.Init.NonInvertingInput = COMP_NONINVERTINGINPUT_PB5; 00553 #elif defined(USE_PB6_FOR_COMP) 00554 COMP_HandleStruct.Init.NonInvertingInput = COMP_NONINVERTINGINPUT_PB6; 00555 #endif 00556 COMP_HandleStruct.Init.Output = COMP_OUTPUT_NONE; 00557 COMP_HandleStruct.Init.Mode = COMP_MODE_HIGHSPEED; 00558 COMP_HandleStruct.Init.WindowMode = COMP_WINDOWMODE_DISABLED; 00559 COMP_HandleStruct.Init.TriggerMode = COMP_TRIGGERMODE_IT_FALLING; 00560 HAL_COMP_Init(&COMP_HandleStruct); 00561 // Interrupt configuration 00562 NVIC_SetVector(COMP_IRQn, (uint32_t)irq_comp2_handler); 00563 HAL_NVIC_SetPriority(COMP_IRQn, 0, 0); 00564 HAL_NVIC_ClearPendingIRQ(COMP_IRQn); 00565 HAL_COMP_Start_IT(&COMP_HandleStruct); 00566 HAL_NVIC_EnableIRQ(COMP_IRQn); 00567 } 00568 #else // defined(USE_IRQ_FOR_RTC_BKUP) 00569 void set_5v_drop_detect(uint8_t function_use) 00570 { 00571 ; // No implementation 00572 } 00573 #endif // defined(USE_IRQ_FOR_RTC_BKUP) 00574 00575 #else // defined(TARGET_STM32L152RE) 00576 void set_5v_drop_detect(uint8_t function_use) 00577 { 00578 ; // No implementation 00579 } 00580 #endif // defined(TARGET_STM32L152RE) 00581 #else // defined(TARGET_STM32F401RE,_F411RE,_L152RE,_F334R8,_L476RG, _F746xx) 00582 #error "No suport this mbed, only for Nucleo mbed" 00583 #endif // defined(TARGET_STM32F401RE,_F411RE,_L152RE,_F334R8,_L476RG, _F746xx) 00584
Generated on Tue Jul 12 2022 20:39:04 by
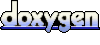