
GPS Data Logger with SD File system. Logger can save RMC & GGA data from GPS and five channels of analog data. Based on http://mbed.org/users/prf/ "GPS_Logger_01" by Peter Forden
main.cpp
00001 //------------------------------------------------------------------------------------------------- 00002 // GPS DATA LOGGER Ver.1 00003 // (c)2010 Kenji Arai / JH1PJL 00004 // http://www.page.sannet.ne.jp/kenjia/index.html 00005 // http://mbed.org/users/kenjiArai/ 00006 // April 17th,2010 Started 00007 // April 17th,2010 00008 //------------------------------------------------------------------------------------------------- 00009 // Reference ----- GPS_logger_01 by Peter Foden ------ 00010 // http://mbed.org/users/prf/ 00011 // http://mbed.org/users/prf/programs/GPS_Logger_01/f5c2b003ae38423640de0fc5e0727190/ 00012 //------------------------------------------------------------------------------------------------- 00013 // Function 00014 // GPS data and five channeles ADC data records into a file which is located in SD-Card 00015 // Connection 00016 // GPS receiver PIN 8,9 00017 // Analog input PIN 15,16,17,19,20 00018 // SD Card I/F PIN 11,12,13,14 00019 // LCD PIN 5,6,7,21,22,23 00020 // -> CAUTION!! pin assignment is different 00021 // with " http://mbed.org/projects/cookbook/wiki/TextLCD " 00022 // RTC PIN 3 needs to connect 3V Battery 00023 // -> Please refer my program " RTC_w_COM" for time adjustment 00024 // at " http://mbed.org/users/kenjiArai/programs/RTC_w_COM/5yi9a/ " 00025 //------------------------------------------------------------------------------------------------- 00026 #include "mbed.h" 00027 #include "TextLCD.h" 00028 #include "SDFileSystem.h" 00029 00030 // Data logging configration 00031 // Save RMC any condition 00032 #define CONFG_ANA 1 // 1= Save Analog data 00033 #define DEBUG 1 // 1= Shows progress on PC via USB ( virtual COM line) 00034 #define USE_LCD 1 // 1= Display the data on LCD 00035 00036 // Commands for GPS to turn on or off data strings 00037 #define RMC_ON "$PSRF103,4,0,1,1*21\r\n" 00038 #define RMC_OFF "$PSRF103,4,0,0,1*20\r\n" 00039 #define GGA_ON "$PSRF103,0,0,1,1*25\r\n" 00040 #define GGA_OFF "$PSRF103,0,0,0,1*24\r\n" 00041 #define GSA_ON "$PSRF103,2,0,1,1*27\r\n" 00042 #define GSA_OFF "$PSRF103,2,0,0,1*26\r\n" 00043 #define GSV_ON "$PSRF103,3,0,1,1*26\r\n" 00044 #define GSV_OFF "$PSRF103,3,0,0,1*27\r\n" 00045 #define WAAS_ON "$PSRF151,1*3F\r\n" 00046 #define WAAS_OFF "$PSRF151,0*3E\r\n" 00047 00048 // kind of GPS data 00049 #define NONE 0 00050 #define RMC 1 00051 #define GGA 2 00052 #define GSA 3 00053 #define GSV 4 00054 00055 // SD Recording status 00056 #define IDLE 0 00057 #define RECD 1 00058 #define SD_FAIL 0xff 00059 00060 // Buffer size 00061 #define BSIZ 256 00062 00063 //------------------------------------------------------------------------------------------------- 00064 // Set up hardware 00065 // MBED USB port used for console debug messages 00066 // SZP950T GPS unit connects to UART 00067 // LED & Switch 00068 // 40chr x 2 line Text LCD 00069 // SD card interface 00070 // Analog input 00071 // G-sensor data, Baterry volt, Temperature sensor data 00072 #if DEBUG 00073 Serial pc(USBTX, USBRX); // tx, rx - Default 9600 baud 00074 #endif 00075 Serial gps(p9, p10); // tx, rx - 4800 baud required 00076 DigitalOut RCV_GPS(LED1); // Receive GPS data 00077 DigitalOut GPS_LOCK(LED2); // GPS got valid data 00078 DigitalOut ON_REC(LED4); // Data sent LED 00079 DigitalIn SW_REC(p26); // Data recode switch 00080 #if USE_LCD 00081 TextLCD lcd(p22, p23, p21, p8, p7, p6, p5, 40, 2); // rs,rw,e,d0,d1,d2,d3,40char's x 2 lines 00082 #endif 00083 SDFileSystem sd(p11, p12, p13, p14, "sd"); // do,di,clk,cs 00084 #if CONFG_ANA 00085 AnalogIn ain_G_X(p15); // G Sensor 00086 AnalogIn ain_G_Y(p16); // G Sensor 00087 AnalogIn ain_G_Z(p17); // G Sensor 00088 AnalogIn ain_BAT(p19); // Battery Volt 00089 AnalogIn ain_TEMP(p20); // Temperature Sensor 00090 #endif 00091 00092 //------------------------------------------------------------------------------------------------- 00093 // Data rea 00094 char msg[BSIZ]; //GPS data buffer 00095 char MsgBuf_RMC[BSIZ]; // GPS/GGA data 00096 char MsgBuf_GGA[BSIZ]; // GPS/RMC data 00097 #if CONFG_ANA 00098 char MsgBuf_ANA[128]; // Analog data buffer 00099 float x,y,z,b,t; // Analog data 00100 #endif 00101 char gps_dat; // Kind of GPS data 00102 char recode_status; 00103 FILE *fp; // File pointer 00104 char buf[40]; // data buffer for text 00105 time_t seconds; // RTC data based on seconds 00106 00107 //------------------------------------------------------------------------------------------------- 00108 // --------------- CONTROL PROGRAM -------------------- 00109 00110 // Select GPS data 00111 void setgps() { 00112 gps.printf(RMC_ON); // use RMC 00113 gps.printf(GGA_ON); // use GGA 00114 gps.printf(GSA_OFF); 00115 gps.printf(GSV_OFF); 00116 gps.printf(WAAS_OFF); 00117 return; 00118 } 00119 00120 // Get line of data from GPS 00121 void getline() { 00122 while (gps.getc() != '$'); // Wait for start of line 00123 msg[0] = '$'; 00124 #if DEBUG 00125 pc.putc('$'); 00126 #endif 00127 for (int i=1; i<512; i++) { 00128 msg[i] = gps.getc(); 00129 #if DEBUG 00130 pc.putc(msg[i]); 00131 #endif 00132 if (msg[i] == '\r' || msg[i] == '\n') { 00133 msg[i] = '\r'; 00134 msg[i+1] = '\n'; 00135 msg[i+2] = 0; 00136 #if DEBUG 00137 pc.printf("\r\n"); 00138 #endif 00139 return; 00140 } 00141 } 00142 } 00143 00144 int main(void) { 00145 gps.baud(4800); // baud rate 4800 00146 #if DEBUG 00147 pc.printf("\r\n\r\nGPS logger on mbed by K.Arai/JH1PJL (c)2010\r\n"); 00148 #endif 00149 #if USE_LCD 00150 lcd.cls(); 00151 lcd.locate(0, 0); 00152 // 1234567890123456789012345678901234567890 00153 lcd.printf("GPS Logger Running! .... "); 00154 #endif 00155 setgps(); 00156 while (1) { 00157 RCV_GPS = 1; 00158 getline(); // Get GPS data from UART 00159 if (strncmp(msg, "$GPRMC",6) == 0) { 00160 for (int i=0; i<BSIZ ; MsgBuf_RMC[i++]=0); // Clear buffer 00161 for (int i=0; msg[i] != 0; i++) { // Copy msg to RMC buffer 00162 MsgBuf_RMC[i] = msg[i]; 00163 } 00164 gps_dat = RMC; 00165 // Get analog data from each port 00166 #if CONFG_ANA 00167 x=ain_G_X.read(); 00168 y=ain_G_Y.read(); 00169 z=ain_G_Z.read(); 00170 b=ain_BAT.read(); 00171 t=ain_TEMP.read(); 00172 sprintf(MsgBuf_ANA, "$ANA,%f,%f,%f,%f,%f,,*00\r\n", x, y, z, b, t); 00173 #if DEBUG 00174 pc.printf(MsgBuf_ANA); 00175 #endif 00176 #endif 00177 } else if (strncmp(msg, "$GPGGA",6) == 0) { 00178 for (int i=0; i<BSIZ ; MsgBuf_GGA[i++]=0); // Clear buffer 00179 for (int i=0; msg[i] != 0; i++) { // Copy msg to GGA buffer 00180 MsgBuf_GGA[i] = msg[i]; 00181 } 00182 gps_dat = GGA; 00183 } else { 00184 gps_dat = NONE; 00185 } 00186 RCV_GPS = 0; 00187 if (SW_REC){ 00188 if (recode_status == RECD){ 00189 // Recording -> on going 00190 ON_REC = 1; // LED ON for recording indication 00191 switch(gps_dat){ 00192 case RMC: { 00193 fprintf(fp,MsgBuf_RMC); // save data 00194 #if CONFG_ANA 00195 fprintf(fp,MsgBuf_ANA); // save data 00196 #endif 00197 break; 00198 } 00199 case GGA: { 00200 fprintf(fp,MsgBuf_GGA); // save data 00201 break; 00202 } 00203 default: {;} 00204 } 00205 } else if (recode_status == IDLE){ 00206 // Start recoding -> File open 00207 seconds = time(NULL); 00208 seconds %= 100000000; // Adjust 8 charcters file name 00209 sprintf(buf,"/sd/%d.txt",seconds); // File name based on time from 1970/1/1 00210 fp = fopen(buf, "w"); // File open 00211 #if DEBUG 00212 pc.printf("\r\n %s \r\n", buf); // File name on the screen 00213 #endif 00214 if(fp == NULL) { 00215 // Try again 00216 fp = fopen(buf, "w"); 00217 if(fp == NULL) { 00218 // File not open then give up 00219 #if USE_LCD 00220 lcd.locate(0, 0); 00221 // 1234567890123456789012345678901234567890 00222 lcd.printf(" Could not open file for write "); 00223 #endif 00224 #if DEBUG 00225 pc.printf( "\r\n Could not open file for write\r\n"); 00226 #endif 00227 recode_status = SD_FAIL; 00228 } 00229 } 00230 if (fp){ 00231 // File open successful 00232 fprintf(fp, "GPS logger on mbed by K.Arai/JH1PJL (c)2010\r\n"); 00233 #if USE_LCD 00234 lcd.locate(0, 0); 00235 // 1234567890123456789012345678901234567890 00236 lcd.printf(" Start recording "); 00237 #endif 00238 #if DEBUG 00239 pc.printf( "GPS logger on mbed by K.Arai/JH1PJL (c)2010"); 00240 pc.printf("\r\nStart recording\r\n"); 00241 #endif 00242 recode_status = RECD; 00243 } 00244 } 00245 } else { 00246 if (recode_status == RECD){ 00247 // File close 00248 fclose(fp); 00249 recode_status = IDLE; // back to idle state 00250 #if USE_LCD 00251 lcd.locate(0, 0); 00252 // 1234567890123456789012345678901234567890 00253 lcd.printf("Finish data save "); 00254 #endif 00255 #if DEBUG 00256 pc.printf( "\r\n Finish data save\r\n"); 00257 #endif 00258 } else if (recode_status == SD_FAIL){ 00259 // When file access failed 00260 recode_status = IDLE; // back to idle state 00261 #if USE_LCD 00262 lcd.locate(0, 0); 00263 // 1234567890123456789012345678901234567890 00264 lcd.printf("Could not save the data "); 00265 #endif 00266 #if DEBUG 00267 pc.printf( "\r\n Could not save the data\r\n"); 00268 #endif 00269 } 00270 ON_REC = 0; // LED off for IDLE 00271 } 00272 } 00273 } 00274
Generated on Thu Jul 14 2022 20:02:46 by
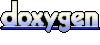