
Control three VL53L0X sensors
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * Mbed Application program -> Three of VL53L0X's are working together 00003 * Time-of-Flight ranging and gesture detection sensor / STMicro VL53L0X 00004 * http://www.st.com/ja/imaging-and-photonics-solutions/vl53l0x.html 00005 * 00006 * 1) AKIZUKI AE-VL53L0X 00007 * http://akizukidenshi.com/catalog/g/gM-12590/ 00008 * 2) SWITCH SCIENCE Pololu VL53L0X (POLOLU-2490) 00009 * https://www.switch-science.com/catalog/2869/ 00010 * 3) SWITCH SCIENCE VL53L0X (SSCI-028943) 00011 * https://www.switch-science.com/catalog/2894/ 00012 * 4) Strawberry Linux 00013 * https://strawberry-linux.com/catalog/items?code=15310 00014 * 00015 * ---- Tested AE-VL53L0X BOARD and handmade circuit ---- 00016 * Tested on below Mbed boards and works fine on mbed-os5 00017 * Nucleo-F446RE 00018 * 00019 * Copyright (c) 2018 Kenji Arai / JH1PJL 00020 * http://www.page.sannet.ne.jp/kenjia/index.html 00021 * http://mbed.org/users/kenjiArai/ 00022 * Created: January 21st, 2018 00023 * Revised: Feburary 6th, 2018 (with updated VL53L0X_simple library) 00024 */ 00025 00026 // Include -------------------------------------------------------------------- 00027 #include "mbed.h" 00028 #include "VL53L0X.h" // revision=7:174594 (as of today) 00029 00030 // Definition ----------------------------------------------------------------- 00031 #warning "New VL53L0X simple lib. was released and this is an example for it. 00032 #warning "Current revision has a trouble with I2C LCD together. 00033 #warning "Investigation is ongoing. 00034 00035 // Constructor ---------------------------------------------------------------- 00036 DigitalOut myled(LED1); 00037 Serial pc(USBTX, USBRX); 00038 //I2C i2c(P0_30, P0_7); // only for TY51822r3 00039 I2C i2c(I2C_SDA, I2C_SCL); 00040 VL53L0X tof_center(i2c, D8); // XSHUT is mandatory for multi setting 00041 VL53L0X tof_left(i2c, D9); // XSHUT is mandatory for multi setting 00042 VL53L0X tof_right(i2c, D10); // XSHUT is mandatory for multi setting 00043 Timer t; 00044 00045 // RAM ------------------------------------------------------------------------ 00046 00047 // ROM / Constant data -------------------------------------------------------- 00048 char *const msg0 = "VL53L0X is running correctly!!\r\n"; 00049 char *const msg1 = "VL53L0X -> something is wrong!!\r\n"; 00050 char *const msg2 = "#,"; 00051 char *const msg3 = "d[mm]=,"; 00052 char *const msg4 = "err, "; 00053 char *const msg5 = "VL53[mS]=, "; 00054 char *const msg6 = "all[mS]=, "; 00055 char *const msg_c = "Center"; 00056 char *const msg_l = "Left"; 00057 char *const msg_r = "Right"; 00058 00059 // Function prototypes -------------------------------------------------------- 00060 00061 //------------------------------------------------------------------------------ 00062 // Control Program 00063 //------------------------------------------------------------------------------ 00064 int main() 00065 { 00066 int status = VL53L0X_ERROR_NONE; 00067 uint32_t data_c = 0; 00068 uint32_t data_l = 0; 00069 uint32_t data_r = 0; 00070 uint32_t count = 0; 00071 uint32_t tm_sensor; 00072 uint32_t tm_all_work; 00073 uint8_t error_num; 00074 00075 status = tof_center.init_sensor(0x22 << 1U);// new addres(not equal others) 00076 pc.printf("--%s--\r\n", msg_c); 00077 if (status == VL53L0X_ERROR_NONE) { 00078 pc.printf(msg0); 00079 } else { 00080 pc.printf(msg1); 00081 } 00082 status = tof_left.init_sensor(0x33 << 1U); // new addres(not equal others) 00083 pc.printf("--%s--\r\n", msg_l); 00084 if (status == VL53L0X_ERROR_NONE) { 00085 pc.printf(msg0); 00086 } else { 00087 pc.printf(msg1); 00088 } 00089 status = tof_right.init_sensor(0x44 << 1U); // new addres(not equal others) 00090 pc.printf("--%s--\r\n", msg_r); 00091 if (status == VL53L0X_ERROR_NONE) { 00092 pc.printf(msg0); 00093 } else { 00094 pc.printf(msg1); 00095 } 00096 status = tof_center.set_mode(range_long_distance_33ms_200cm); 00097 //status = tof_center.set_mode(range_hi_accurate_200ms_120cm); 00098 //status = tof_center.set_mode(range_hi_speed_20ms_120cm); 00099 if (status == VL53L0X_ERROR_NONE) { 00100 pc.printf(msg0); 00101 } else { 00102 pc.printf(msg1); 00103 } 00104 status = tof_left.set_mode(range_long_distance_33ms_200cm); 00105 if (status == VL53L0X_ERROR_NONE) { 00106 pc.printf(msg0); 00107 } else { 00108 pc.printf(msg1); 00109 } 00110 status = tof_right.set_mode(range_long_distance_33ms_200cm); 00111 if (status == VL53L0X_ERROR_NONE) { 00112 pc.printf(msg0); 00113 } else { 00114 pc.printf(msg1); 00115 } 00116 while(true) { 00117 t.reset(); 00118 t.start(); 00119 myled = !myled; 00120 error_num = 0; 00121 status = tof_center.get_distance(&data_c); 00122 if (status) { 00123 error_num = 1U << 0; 00124 } 00125 status = tof_left.get_distance(&data_l); 00126 if (status) { 00127 error_num |= 1U << 1; 00128 } 00129 status |= tof_right.get_distance(&data_r); 00130 if (status) { 00131 error_num |= 1U << 2; 00132 } 00133 tm_sensor = t.read_ms(); 00134 pc.printf("%s%5d,%s%5d,%5d,%5d,", 00135 msg2, count++, msg3, data_c, data_l, data_r); 00136 pc.printf("%s%d,%s%d,%s%1d\r\n", 00137 msg5, tm_sensor, msg6, tm_all_work, msg4,error_num); 00138 tm_all_work = t.read_ms(); 00139 if (tm_all_work < 199) { 00140 wait_ms(200 - tm_all_work); 00141 } 00142 } 00143 }
Generated on Wed Jul 20 2022 02:04:16 by
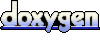