
Arduino board run on Mbed-os6.8.1 (only test purpose not official). Need Mbed Studio(1.3.1) not online compiler. If you compile on the online compiler, you can get a hex file but it does NOT work!!!
Dependencies: APDS_9960 LPS22HB LSM9DS1 HTS221
usb_serial_as_stdio.h
00001 /* 00002 * mbed Application program 00003 * Redirect Standard Input/Output 00004 * 00005 * Copyright (c) 2021 Kenji Arai / JH1PJL 00006 * http://www7b.biglobe.ne.jp/~kenjia/ 00007 * https://os.mbed.com/users/kenjiArai/ 00008 * Created: January 13th, 2021 00009 * Revised: February 28th, 2021 00010 */ 00011 00012 // Include -------------------------------------------------------------------- 00013 //#include "mbed.h" 00014 #include "USBSerial.h" 00015 00016 // Definition ----------------------------------------------------------------- 00017 00018 // Constructor ---------------------------------------------------------------- 00019 USBSerial *usb = NULL; 00020 00021 // RAM ------------------------------------------------------------------------ 00022 00023 // ROM / Constant data -------------------------------------------------------- 00024 00025 // Function prototypes -------------------------------------------------------- 00026 00027 //------------------------------------------------------------------------------ 00028 // Control Program 00029 //------------------------------------------------------------------------------ 00030 /* 00031 https://cpplover.blogspot.com/2010/03/variadic-templates.html 00032 */ 00033 template <typename ... Args> 00034 void print_usb(const char *format, Args const & ... args) 00035 { 00036 if (usb == NULL) { 00037 return; 00038 } else { 00039 if (usb->connected() == true) { 00040 usb->printf(format, args ...); 00041 } 00042 } 00043 } 00044 00045 void usb_serial_initialize(void) 00046 { 00047 usb = new USBSerial; 00048 } 00049 00050 uint8_t readable() 00051 { 00052 return usb->readable(); 00053 } 00054 00055 void putc(uint8_t c) 00056 { 00057 if (usb == NULL) { 00058 return; 00059 } else { 00060 usb->putc(c); 00061 } 00062 } 00063 00064 uint8_t getc() 00065 { 00066 if (usb == NULL) { 00067 while(true){ ;} // infinit loop 00068 } else { 00069 return usb->getc(); 00070 } 00071 }
Generated on Thu Jul 14 2022 06:04:06 by
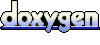