
Arduino board run on Mbed-os6.8.1 (only test purpose not official). Need Mbed Studio(1.3.1) not online compiler. If you compile on the online compiler, you can get a hex file but it does NOT work!!!
Dependencies: APDS_9960 LPS22HB LSM9DS1 HTS221
main0.cpp
00001 /* 00002 * Mbed Application program 00003 * Nano 33 BLE Sense board runs on mbed-OS6 00004 * 3 LED (Y+G+RGB) blinky 00005 * 00006 * Copyright (c) 2020,'21 Kenji Arai / JH1PJL 00007 * http://www7b.biglobe.ne.jp/~kenjia/ 00008 * https://os.mbed.com/users/kenjiArai/ 00009 * Started: January 22nd, 2020 00010 * Revised: February 28th, 2021 00011 */ 00012 00013 // Pre-selection -------------------------------------------------------------- 00014 #include "select_example.h" 00015 //#define EXAMPLE_0_BLINKY_LED 00016 #ifdef EXAMPLE_0_BLINKY_LED 00017 00018 // Include -------------------------------------------------------------------- 00019 #include "mbed.h" 00020 #include "nano33blesense_iodef.h" 00021 00022 // Definition ----------------------------------------------------------------- 00023 00024 // Constructor ---------------------------------------------------------------- 00025 DigitalOut led_y(PIN_YELLOW, 0); 00026 DigitalOut led_g(PIN_GREEN, 0); 00027 DigitalOut lr(PIN_LR, 1); 00028 DigitalOut lg(PIN_LG, 1); 00029 DigitalOut lb(PIN_LB, 1); 00030 00031 // RAM ------------------------------------------------------------------------ 00032 00033 // ROM / Constant data -------------------------------------------------------- 00034 00035 // Function prototypes -------------------------------------------------------- 00036 00037 // subroutin (must be at this below line and do NOT change order) ------------- 00038 #include "usb_serial_as_stdio.h" 00039 #include "check_revision.h" 00040 #include "common.h" 00041 00042 //------------------------------------------------------------------------------ 00043 // Control Program 00044 //------------------------------------------------------------------------------ 00045 int main() 00046 { 00047 usb_serial_initialize(); 00048 print_revision(); 00049 ThisThread::sleep_for(100ms); 00050 uint32_t counter = 0; 00051 while (true) { 00052 print_usb("Blinky LED's %u\r\n", ++counter); 00053 led_y = 1; 00054 led_g = 0; 00055 lr = 1; 00056 lg = 1; 00057 lb = 1; 00058 ThisThread::sleep_for(1s); 00059 led_y = 0; 00060 led_g = 1; 00061 lr = 1; 00062 lg = 1; 00063 lb = 1; 00064 ThisThread::sleep_for(1s); 00065 led_y = 0; 00066 led_g = 0; 00067 lr = 0; 00068 lg = 1; 00069 lb = 1; 00070 ThisThread::sleep_for(1s); 00071 led_y = 0; 00072 led_g = 0; 00073 lr = 1; 00074 lg = 0; 00075 lb = 1; 00076 ThisThread::sleep_for(1s); 00077 led_y = 0; 00078 led_g = 0; 00079 lr = 1; 00080 lg = 1; 00081 lb = 0; 00082 ThisThread::sleep_for(1s); 00083 led_y = 1; 00084 led_g = 1; 00085 lr = 0; 00086 lg = 0; 00087 lb = 0; 00088 ThisThread::sleep_for(1s); 00089 led_y = 0; 00090 led_g = 0; 00091 lr = 1; 00092 lg = 1; 00093 lb = 1; 00094 ThisThread::sleep_for(1s); 00095 } 00096 } 00097 00098 #if 0 00099 /* 00100 https://cpplover.blogspot.com/2010/03/variadic-templates.html 00101 */ 00102 template <typename ... Args> 00103 void print_usb(const char *format, Args const & ... args) 00104 { 00105 if (usb == NULL) { 00106 return; 00107 } else { 00108 if (usb->connected() == true) { 00109 usb->printf(format, args ...); 00110 } 00111 } 00112 } 00113 00114 uint8_t readable() 00115 { 00116 return usb->readable(); 00117 } 00118 00119 void putc(uint8_t c) 00120 { 00121 if (usb == NULL) { 00122 return; 00123 } else { 00124 usb->putc(c); 00125 } 00126 } 00127 00128 uint8_t getc() 00129 { 00130 if (usb == NULL) { 00131 while(true){ ;} // infinit loop 00132 } else { 00133 return usb->getc(); 00134 } 00135 } 00136 #endif 00137 00138 #endif // EXAMPLE_0_BLINKY_LED
Generated on Thu Jul 14 2022 06:04:06 by
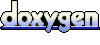