Text console library for ST7032 text LCD controller over I2C interface.
Embed:
(wiki syntax)
Show/hide line numbers
TextLCD_ST7032I2C.h
00001 // ==================================================== Mar 18 2015, kayeks == 00002 // TextLCD_ST7032I2C.h 00003 // =========================================================================== 00004 // Text console library for ST7032 text LCD controller over I2C interface. 00005 00006 #ifndef TEXTLCD_ST7032I2C_H_ 00007 #define TEXTLCD_ST7032I2C_H_ 00008 00009 #include "mbed.h" 00010 00011 #define MIN(x, y) ((x) < (y) ? (x) : (y)) 00012 00013 /** Text console library for ST7032 text LCD controller over I2C interface. */ 00014 class TextLCD_ST7032I2C : public Stream { 00015 public: 00016 /** Bias select: 1/4 or 1/5. */ 00017 enum Bias { 00018 Bias1_4 /** 1/4 bias */ 00019 , Bias1_5 /** 1/5 bias */ 00020 }; 00021 00022 const static uint8_t CLEAR_DISPLAY = 0x01; 00023 const static uint8_t RETURN_HOME = 0x02; 00024 const static uint8_t ENTRY_MODE_DECR_NO_SHIFT = 0x04; 00025 const static uint8_t ENTRY_MODE_DECR_SHIFT = 0x05; 00026 const static uint8_t ENTRY_MODE_INCR_NO_SHIFT = 0x06; 00027 const static uint8_t ENTRY_MODE_INCR_SHIFT = 0x07; 00028 const static uint8_t DISPLAY_OFF_CURSOR_OFF_POS_OFF = 0x08; 00029 const static uint8_t DISPLAY_OFF_CURSOR_OFF_POS_ON = 0x09; 00030 const static uint8_t DISPLAY_OFF_CURSOR_ON_POS_OFF = 0x0a; 00031 const static uint8_t DISPLAY_OFF_CURSOR_ON_POS_ON = 0x0b; 00032 const static uint8_t DISPLAY_ON_CURSOR_OFF_POS_OFF = 0x0c; 00033 const static uint8_t DISPLAY_ON_CURSOR_OFF_POS_ON = 0x0d; 00034 const static uint8_t DISPLAY_ON_CURSOR_ON_POS_OFF = 0x0e; 00035 const static uint8_t DISPLAY_ON_CURSOR_ON_POS_ON = 0x0f; 00036 const static uint8_t FUNCTION_4B_1LINE_7DOT_IS0 = 0x20; 00037 const static uint8_t FUNCTION_4B_1LINE_7DOT_IS1 = 0x21; 00038 const static uint8_t FUNCTION_4B_1LINE_11DOT_IS0 = 0x24; 00039 const static uint8_t FUNCTION_4B_1LINE_11DOT_IS1 = 0x25; 00040 const static uint8_t FUNCTION_4B_2LINE_7DOT_IS0 = 0x28; 00041 const static uint8_t FUNCTION_4B_2LINE_7DOT_IS1 = 0x29; 00042 const static uint8_t FUNCTION_4B_2LINE_11DOT_IS0 = 0x2c; 00043 const static uint8_t FUNCTION_4B_2LINE_11DOT_IS1 = 0x2d; 00044 const static uint8_t FUNCTION_8B_1LINE_7DOT_IS0 = 0x30; 00045 const static uint8_t FUNCTION_8B_1LINE_7DOT_IS1 = 0x31; 00046 const static uint8_t FUNCTION_8B_1LINE_11DOT_IS0 = 0x34; 00047 const static uint8_t FUNCTION_8B_1LINE_11DOT_IS1 = 0x35; 00048 const static uint8_t FUNCTION_8B_2LINE_7DOT_IS0 = 0x38; 00049 const static uint8_t FUNCTION_8B_2LINE_7DOT_IS1 = 0x39; 00050 const static uint8_t FUNCTION_8B_2LINE_11DOT_IS0 = 0x3c; 00051 const static uint8_t FUNCTION_8B_2LINE_11DOT_IS1 = 0x3d; 00052 const static uint8_t DDRAM = 0x80; 00053 const static uint8_t IS0_LEFT_MOVE = 0x10; 00054 const static uint8_t IS0_LEFT_SHIFT = 0x14; 00055 const static uint8_t IS0_RIGHT_MOVE = 0x18; 00056 const static uint8_t IS0_RIGHT_SHIFT = 0x1c; 00057 const static uint8_t IS0_CGRAM = 0x40; 00058 const static uint8_t IS1_BIAS5_OSC = 0x10; 00059 const static uint8_t IS1_BIAS4_OSC = 0x18; 00060 const static uint8_t IS1_ICON_ADDR = 0x40; 00061 const static uint8_t IS1_ICON_OFF_BOOST_OFF_CONTRAST = 0x50; 00062 const static uint8_t IS1_ICON_OFF_BOOST_ON_CONTRAST = 0x54; 00063 const static uint8_t IS1_ICON_ON_BOOST_OFF_CONTRAST = 0x58; 00064 const static uint8_t IS1_ICON_ON_BOOST_ON_CONTRAST = 0x5c; 00065 const static uint8_t IS1_FOLLOWER_OFF_RAB = 0x60; 00066 const static uint8_t IS1_FOLLOWER_ON_RAB = 0x68; 00067 const static uint8_t IS1_CONTRAST = 0x70; 00068 00069 private: 00070 uint8_t** _lineBuffer; 00071 uint8_t _column, _row; 00072 uint8_t _columns, _rows; 00073 uint8_t _address; 00074 uint8_t _osc, _rab, _contrast; 00075 Bias _bias; 00076 I2C _i2c; 00077 00078 public: 00079 /** Constructor of class TextLCD_ST7032I2C. 00080 * @param sda I2C SPI data output (MOSI) pin. 00081 * @param scl SPI clock output (SCK) pin. 00082 * @param address I2C address of the LCD device. 00083 * @param columns Number of characters in a row. 00084 * @param rows Number of rows. 00085 */ 00086 TextLCD_ST7032I2C(PinName sda, PinName scl, uint8_t address, 00087 uint8_t columns, uint8_t rows); 00088 00089 /** Destructor of class TextLCD_ST7032I2C. */ 00090 virtual ~TextLCD_ST7032I2C(); 00091 00092 /** Hit hardware reset pin. */ 00093 void reset(); 00094 00095 /** Initialize controller. 00096 * @param osc Oscillator configuration (0..7). 00097 * @param rab Rab value setting (0..7). 00098 * @param contrast Contrast setting (0..63). 00099 * @param bias Bias configuration for your LCD. 00100 */ 00101 void init(uint8_t osc, uint8_t rab, uint8_t contrast, Bias bias); 00102 00103 /** Clear display and set cursor to home. */ 00104 void cls(); 00105 00106 /** Set cursor position for next character. 00107 * @param column Column position indexed from 0. 00108 * @param row Row position indexed from 0. 00109 */ 00110 void locate(uint8_t column, uint8_t row); 00111 00112 /** Enable icon display. */ 00113 void iconOn(); 00114 00115 /** Disable icon display. */ 00116 void iconOff(); 00117 00118 /** Set icon display pattern. 00119 * @param iconAddr Icon address (0x00..0x0f). 00120 * @param bits Icon bit pattern (0x00..0x1f). 00121 */ 00122 void setIcon(uint8_t iconAddr, uint8_t bits); 00123 00124 private: 00125 /** Implementation of putc from Stream class. */ 00126 virtual int _putc(int c); 00127 00128 /** Implementation of getc from Stream class. */ 00129 virtual int _getc(); 00130 00131 /** Shift up the lower line for scrolling. */ 00132 void shiftUp(); 00133 00134 /** Clear entire display. */ 00135 void clear(); 00136 00137 /** Set instruction set to 0. */ 00138 void is0(); 00139 00140 /** Set instruction set to 1. */ 00141 void is1(); 00142 00143 /** Write a command byte for LCD controller. 00144 * @param c The command byte. 00145 */ 00146 void command(uint8_t c); 00147 00148 /** Write a data byte for LCD controller. 00149 * @param d The data byte. 00150 */ 00151 void data(uint8_t d); 00152 }; 00153 00154 #endif
Generated on Mon Aug 1 2022 03:55:34 by
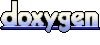