Text console library for ST7032 text LCD controller over I2C interface.
Embed:
(wiki syntax)
Show/hide line numbers
TextLCD_ST7032I2C.cpp
00001 // ==================================================== Mar 18 2015, kayeks == 00002 // TextLCD_ST7032I2C.cpp 00003 // =========================================================================== 00004 // Text console library for ST7032 text LCD controller over I2C interface. 00005 00006 #include <string.h> 00007 #include "TextLCD_ST7032I2C.h" 00008 00009 TextLCD_ST7032I2C::TextLCD_ST7032I2C(PinName sda, PinName scl, uint8_t address, 00010 uint8_t columns, uint8_t rows) 00011 : 00012 _i2c(sda, scl) 00013 { 00014 // Copy parameters 00015 _columns = MIN(columns, 16); 00016 _rows = MIN(rows, 2); 00017 _address = address & 0xfe; 00018 00019 // Allocate line buffer 00020 _lineBuffer = new uint8_t*[_rows]; 00021 for (uint8_t i = 0; i < _rows; i++) { 00022 _lineBuffer[i] = new uint8_t[_columns]; 00023 } 00024 } 00025 00026 TextLCD_ST7032I2C::~TextLCD_ST7032I2C() { 00027 for (uint8_t i = 0; i < _rows; i++) { 00028 delete[] _lineBuffer[i]; 00029 } 00030 delete[] _lineBuffer; 00031 } 00032 00033 void TextLCD_ST7032I2C::clear() { 00034 for (uint8_t j = 0; j < _rows; j++) { 00035 locate(0, j); 00036 for (uint8_t i = 0; i < _columns; i++) { 00037 data(' '); 00038 } 00039 } 00040 } 00041 00042 void TextLCD_ST7032I2C::init(uint8_t osc, uint8_t rab, uint8_t contrast, Bias bias) { 00043 // Copy parameters 00044 _osc = osc & 0x07; 00045 _rab = MIN(rab, 7); 00046 _contrast = MIN(contrast, 63); 00047 _bias = bias; 00048 00049 command(FUNCTION_8B_2LINE_7DOT_IS0); 00050 command(FUNCTION_8B_2LINE_7DOT_IS1); 00051 switch (bias) { 00052 case Bias1_4: 00053 command(IS1_BIAS4_OSC + _osc); 00054 break; 00055 case Bias1_5: 00056 command(IS1_BIAS5_OSC + _osc); 00057 break; 00058 } 00059 command(IS1_CONTRAST + (_contrast & 0x0f)); 00060 command(IS1_ICON_ON_BOOST_ON_CONTRAST + (_contrast >> 4)); 00061 command(IS1_FOLLOWER_ON_RAB + _rab); 00062 if (_rows == 1) { 00063 command(FUNCTION_8B_1LINE_7DOT_IS0); 00064 } else if (_rows == 2) { 00065 command(FUNCTION_8B_2LINE_7DOT_IS0); 00066 } 00067 command(DISPLAY_ON_CURSOR_OFF_POS_OFF); 00068 cls(); 00069 } 00070 00071 void TextLCD_ST7032I2C::cls() { 00072 clear(); 00073 for (uint8_t j = 0; j < _rows; j++) { 00074 memset(_lineBuffer[j], ' ', _columns); 00075 } 00076 _column = 0; 00077 _row = 0; 00078 } 00079 00080 void TextLCD_ST7032I2C::locate(uint8_t column, uint8_t row) { 00081 if (column < _columns && row < _rows) { 00082 command(DDRAM + ((row << 6) | column)); 00083 _column = column; 00084 _row = row; 00085 } 00086 } 00087 00088 void TextLCD_ST7032I2C::iconOn() { 00089 is1(); 00090 command(IS1_ICON_ON_BOOST_ON_CONTRAST + (_contrast >> 4)); 00091 } 00092 00093 void TextLCD_ST7032I2C::iconOff() { 00094 is1(); 00095 command(IS1_ICON_OFF_BOOST_ON_CONTRAST + (_contrast >> 4)); 00096 } 00097 00098 void TextLCD_ST7032I2C::setIcon(uint8_t iconAddr, uint8_t bits) { 00099 is1(); 00100 command(IS1_ICON_ADDR + (iconAddr & 0x0f)); 00101 data(bits & 0x1f); 00102 } 00103 00104 int TextLCD_ST7032I2C::_putc(int c) { 00105 switch (c) { 00106 case '\r': // Carriage return 00107 _column = 0; 00108 locate(_column, _row); 00109 break; 00110 case '\n': // Line feed 00111 if (_row == _rows - 1) { 00112 shiftUp(); 00113 } else { 00114 _row++; 00115 } 00116 break; 00117 case '\f': // Form feed 00118 // Clear screen 00119 cls(); 00120 break; 00121 default: 00122 // Break line if the cursor reaches end 00123 if (_column == _columns) { 00124 if (_row == _rows - 1) { 00125 shiftUp(); 00126 } else { 00127 _row++; 00128 } 00129 _column = 0; 00130 } 00131 locate(_column, _row); 00132 data(c); 00133 _lineBuffer[_row][_column] = c; 00134 _column++; 00135 break; 00136 } 00137 return 0; 00138 } 00139 00140 int TextLCD_ST7032I2C::_getc() { 00141 return 0; 00142 } 00143 00144 void TextLCD_ST7032I2C::shiftUp() { 00145 // Move line buffer content 00146 for (uint8_t j = 0; j < _rows - 1; j++) { 00147 memcpy(_lineBuffer[j], _lineBuffer[j + 1], _columns); 00148 } 00149 // Clear last line 00150 memset(_lineBuffer[_rows - 1], ' ', _columns); 00151 00152 // Redraw 00153 for (uint8_t j = 0; j < _rows; j++) { 00154 locate(0, j); 00155 for (uint8_t i = 0; i < _columns; i++) { 00156 data(_lineBuffer[j][i]); 00157 } 00158 } 00159 } 00160 00161 void TextLCD_ST7032I2C::is0() { 00162 if (_rows == 1) { 00163 command(FUNCTION_8B_1LINE_7DOT_IS0); 00164 } else if (_rows == 2) { 00165 command(FUNCTION_8B_2LINE_7DOT_IS0); 00166 } 00167 } 00168 00169 void TextLCD_ST7032I2C::is1() { 00170 if (_rows == 1) { 00171 command(FUNCTION_8B_1LINE_7DOT_IS1); 00172 } else if (_rows == 2) { 00173 command(FUNCTION_8B_2LINE_7DOT_IS1); 00174 } 00175 } 00176 00177 void TextLCD_ST7032I2C::command(uint8_t c) { 00178 char cbuf[2]; 00179 00180 cbuf[0] = 0x00; 00181 cbuf[1] = c; 00182 _i2c.write(_address, cbuf, 2); 00183 } 00184 00185 void TextLCD_ST7032I2C::data(uint8_t d) { 00186 char dbuf[2]; 00187 00188 dbuf[0] = 0x40; 00189 dbuf[1] = d; 00190 _i2c.write(_address, dbuf, 2); 00191 }
Generated on Mon Aug 1 2022 03:55:34 by
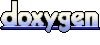