
Example of library ST7565SPI: Driver library for ST7565 graphics LCD controller over SPI interface.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "ST7565SPI.h" 00003 00004 ST7565SPI lcd(/*MOSI*/ p5, /*SCK*/ p7, /*CS*/ p8, 00005 /*RS*/ p11, /*RST*/ p12, /*Frequency*/ 1000000); 00006 00007 // Dithered gray patterns 00008 uint8_t const pattern[8][4] = { 00009 { 0xff, 0xff, 0xff, 0xff }, // 100% 00010 { 0x77, 0xff, 0xdd, 0xff }, // 87.5% 00011 { 0x55, 0xff, 0x55, 0xff }, // 75% 00012 { 0x55, 0xbb, 0x55, 0xee }, // 62.5% 00013 { 0x55, 0xaa, 0x55, 0xaa }, // 50% 00014 { 0x55, 0x22, 0x55, 0x88 }, // 37.5% 00015 { 0x55, 0x00, 0x55, 0x00 }, // 25% 00016 { 0x11, 0x00, 0x44, 0x00 } // 12.5% 00017 }; 00018 00019 int main(void) { 00020 // Initialize LCD 00021 lcd.init(/*V0*/ 3, /*Contrast*/ 48, /*Bias*/ ST7565SPI::Bias1_9); 00022 00023 // Draw horizontal stripes 00024 for (uint8_t j = 0; j < 8; j++) { 00025 lcd.setPage(j); 00026 lcd.setColumn(0); 00027 for (uint8_t i = 0; i < 128 / 4; i++) { 00028 lcd.data(pattern[j][0]); 00029 lcd.data(pattern[j][1]); 00030 lcd.data(pattern[j][2]); 00031 lcd.data(pattern[j][3]); 00032 } 00033 } 00034 00035 // Scroll entire display by changing line offset values 00036 uint8_t c = 0; 00037 while (1) { 00038 lcd.command(ST7565SPI::COMMON_OFFSET + c); 00039 if (c == 0) { 00040 c = 63; 00041 } else { 00042 c--; 00043 } 00044 wait(.25); 00045 } 00046 }
Generated on Fri Jul 15 2022 01:55:54 by
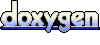