...
Embed:
(wiki syntax)
Show/hide line numbers
Shape.h
00001 #ifndef MY_SHAPE 00002 #define MY_SHAPE 00003 00004 #include "mbed.h" 00005 00006 /** 00007 * A library to create square and rectangle objects. calculates the area and perimeter 00008 * 00009 * Example: 00010 * @code 00011 * 00012 * #include "mbed.h" 00013 * #include "Shape.h" 00014 * 00015 * Shape rect(3,4); 00016 * Shape squr(1); 00017 * 00018 * int main() { 00019 * printf(" area of rect = %d \n", rect.getArea()); 00020 * printf("perimeter of square = %d \n", squr.getPerimeter()); 00021 * } 00022 * 00023 * @endcode 00024 * 00025 */ 00026 00027 class Shape{ 00028 public: 00029 /** create an object for square 00030 * @param x integer value for a side on square 00031 */ 00032 Shape(int x); 00033 00034 00035 /** create an object for rectangle 00036 * @param x integer value for length of rectangle 00037 * @param y integer value for breadth of rectangle 00038 */ 00039 Shape(int x, int y); 00040 00041 /** returns to area of rectangle/square 00042 * @return returns the area of rectangle/square 00043 */ 00044 int getArea(); 00045 00046 00047 /** returns to perimeter of rectangle/square 00048 * @return returns the perimeter of rectangle/square 00049 */ 00050 int getPerimeter(); 00051 00052 private: 00053 int _x; 00054 int _y; 00055 int _area; 00056 int _perim; 00057 }; 00058 00059 #endif
Generated on Wed Jul 13 2022 07:28:13 by
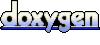