LTC2942 interface with interrupt and battery register calculations
Embed:
(wiki syntax)
Show/hide line numbers
LTC294X.h
00001 #ifndef LTC294X_H 00002 #define LTC294X_H 00003 00004 #include "mbed.h" 00005 00006 #define LTC_CONSOLE_OUTOUT 1 /* Set this if you need debug messages on the console; */ 00007 /* it will have an impact on code-size and power consumption. */ 00008 00009 #if LTC_CONSOLE_OUTOUT 00010 //Serial usb(USBTX, USBRX); // tx, rx 00011 extern Serial pc; 00012 #define DEBUG(...) { pc.printf(__VA_ARGS__); } 00013 #else 00014 #define DEBUG(...) /* nothing */ 00015 #endif /* #if NEED_CONSOLE_OUTPUT */ 00016 00017 //gets a bit position by shifting 1 00018 #define _BV(x) (1 << x) 00019 00020 // sbi sets a particular bit and cbi clears the bit (target byte-word, bit position) 00021 #define sbi(y,x); y|=_BV(x) 00022 #define cbi(y,x); y&=~_BV(x) 00023 00024 00025 //BIT 7-6 ADC MODE SELECTOR 00026 #define ADC_AUTO 0x00 00027 #define ADC_VOLT 0x01 00028 #define ADC_TEMP 0x02 00029 #define ADC_SLEEP 0x03 00030 00031 //BIT 2-1 AL/CC MODE SELECTOR 00032 #define ALCC_ALERT 0x04 00033 #define ALCC_CCOMP 0x05 00034 #define ALCC_OFF 0x06 00035 00036 //STATUS REGISTER.. READ BIT VALUES ONLY 00037 #define CHIP_ID _BV(7) 00038 #define RESERVE _BV(6) 00039 #define CHARGE_OVUV_ALERT _BV(5) 00040 #define TEMPERATURE_ALERT _BV(4) 00041 #define CHARGE_HIGH_ALERT _BV(3) 00042 #define CHARGE_LOW_ALERT _BV(2) 00043 #define VOLT_ALERT _BV(1) 00044 #define UVLO_ALERT _BV(0) 00045 00046 00047 //prescaler options 00048 #define PRESCALE_128 7 00049 #define PRESCALE_64 6 00050 #define PRESCALE_32 5 00051 #define PRESCALE_16 4 00052 #define PRESCALE_8 3 00053 #define PRESCALE_4 2 00054 #define PRESCALE_2 1 00055 #define PRESCALE_1 0 00056 00057 //CONTROL REGISTER.. BIT LOCATIONS 00058 #define ADC_VOLT_MODE 7 00059 #define ADC_TEMP_MODE 6 00060 #define PRESCALER_2 5 00061 #define PRESCALER_1 4 00062 #define PRESCALER_0 3 00063 #define ALCC_ALERT_MODE 2 00064 #define ALCC_CHRGC_MODE 1 00065 #define IC_SHUTDOWN 0 00066 00067 //I2C REGISTER ADDRESSES 00068 #define REG_STATUS 0x00 // 00h A Status R See Below 00069 #define REG_CONTROL 0x01 // 01h B Control R/W 3Ch 00070 #define ACC_MSB 0x02 // 02h C Accumulated Charge MSB R/W 7Fh 00071 #define ACC_LSB 0x03 // 03h D Accumulated Charge LSB R/W FFh 00072 #define CHRG_THH_MSB 0x04 // 04h E Charge Threshold High MSB R/W FFh 00073 #define CHRG_THH_LSB 0x05 // 05h F Charge Threshold High LSB R/W FFh 00074 #define CHRG_THL_MSB 0x06 // 06h G Charge Threshold Low MSB R/W 00h 00075 #define CHRG_THL_LSB 0x07 // 07h H Charge Threshold Low LSB R/W 00h 00076 #define VOLT_MSB 0x08 // 08h I Voltage MSB R XXh 00077 #define VOLT_LSB 0x09 // 09h J Voltage LSB R XXh 00078 #define VOLT_THH 0x0A // 0Ah K Voltage Threshold High R/W FFh 00079 #define VOLT_THL 0x0B // 0Bh L Voltage Threshold Low R/W 00h 00080 #define TEMP_MSB 0x0C // 0Ch M Temperature MSB R XXh 00081 #define TEMP_LSB 0x0D // 0Dh N Temperature LSB R XXh 00082 #define TEMPERATURE_THH 0x0E // 0Eh O Temperature Threshold High R/W FFh 00083 #define TEMPERATURE_THL 0x0F // 0Fh P Temperature Threshold Low R/W 00h 00084 00085 00086 /** LTC29421 class. 00087 * interface LTC29421 coloumb counter on i2c port with interrupt, function name (and battery capacity in Ah Amp-hour). 00088 * 00089 * Example: 00090 * @code 00091 * #include "mbed.h" 00092 * #include "LTC29421.h" 00093 * 00094 * LTC29421 ltc(PTC9, PTC8, PTA4, &interrDOWN, 0.560); 00095 * 00096 * int main() { 00097 * float chLevel = ltc.accumulatedCharge(); 00098 * } 00099 * @endcode 00100 */ 00101 00102 class LTC29421{ 00103 public: 00104 /** create LTC29421 instance based on I2C, WARNING! use setPrescAndBattCap() to set Battery Capacity 00105 * 00106 * @param pin sda 00107 * @param pin scl 00108 * @param pin al/cc interrupt 00109 */ 00110 LTC29421(PinName, PinName, PinName, void (*interruptFunc)(void)); 00111 00112 /** create LTC29421 instance based on I2C, 00113 * this will computer internal M prescale value and set internal max charge level 00114 * 00115 * @param pin sda 00116 * @param pin scl 00117 * @param pin al/cc interrupt 00118 * @param float battery capacity in Ah (Amp-hour) 00119 */ 00120 LTC29421(PinName, PinName, PinName, void (*interruptFunc)(void), float); 00121 00122 00123 /** select an ADC measurement option for voltage / temperature 00124 * 00125 * @param ADC_x 00126 */ 00127 void setADCMode(int); 00128 00129 /** select a prescaler value (not required if object initialized with battery capacity 00130 * 00131 * @param PRESCALE_x 00132 */ 00133 void setPrescaler(int); 00134 00135 /** returns prescale value in integer 00136 * 00137 * @returns int prescale value 00138 */ 00139 int getPrescaler(); 00140 00141 /** configure the AL/CC interrupt: TODO works as Alarm only 00142 * 00143 * @param ALCC_x 00144 */ 00145 void configALCC(int); 00146 00147 /** shutsdown the analog section of LTC2942, also used to read accumulated charge registers 00148 * 00149 * @param none 00150 */ 00151 void shutdown(); 00152 00153 /** wakes the analog section of LTC2942 00154 * 00155 * @param none 00156 */ 00157 void wake(); 00158 00159 /** wakes the analog section of LTC2942 00160 * 00161 * @param none 00162 */ 00163 00164 /** amend the battery capacity, also computers prescaler M and adjusts internal Max battery capacity 00165 * 00166 * @param battery capacity in Ah (Amp-hour) 00167 */ 00168 void setPrescAndBattCap(float); 00169 00170 /** returns the 2 Byte accumulated charge register 00171 * 00172 * @return accumulated charge read as 2 byte hex value 00173 */ 00174 int accumulatedChargeReg(); 00175 00176 /** write into the 2 Byte accumulated charge register 00177 * 00178 * @param accumulated charge write as 2 byte hex value 00179 */ 00180 void accumulatedChargeReg(int); 00181 00182 /** reads the accumulated charge register as a percent value computed from max battery capacity 00183 * 00184 * @return accumulated charge read as percent 00185 */ 00186 float accumulatedCharge(); 00187 00188 /** writes the accumulated charge register as a percent value computed from max battery capacity 00189 * use this to initialise the battery capacity if known, 00190 * or set it to 50% or set it to 100% if battery capacity reaches max 00191 * 00192 * @param accumulated charge as percent (0-100) 00193 */ 00194 void accumulatedCharge(float); 00195 00196 /** set low battery capacity threshhold 00197 * 00198 * @param float percent remaining value(0-100) 00199 */ 00200 void setChargeThresholdLow(float); 00201 00202 /** set high battery capacity threshhold 00203 * 00204 * @param float percent remaining value(0-100) 00205 */ 00206 void setChargeThresholdHigh(float); 00207 00208 /** obtains battery voltage at (sense-) pin 00209 * if required ADC is set 00210 * 00211 * @return battery level in Volts 00212 */ 00213 float voltage(); 00214 00215 /** set low battery level threshhold 00216 * 00217 * @param Voltage level in Volts 00218 */ 00219 void setVoltageThresholdLow(float); 00220 00221 /** set high battery level threshhold 00222 * 00223 * @param Voltage level in Volts 00224 */ 00225 void setVoltageThresholdHigh(float); 00226 00227 /** obtains IC temperature, if required ADC is set 00228 * 00229 * @return temperature in centigrade 00230 */ 00231 float temperature(); 00232 00233 /** set IC temperature low threshold 00234 * 00235 * @param temperauture in centigrade 00236 */ 00237 void setTemperatureThresholdLow(float); 00238 00239 /** set IC temperature high threshold 00240 * 00241 * @param temperauture in centigrade 00242 */ 00243 void setTemperatureThresholdHigh(float); 00244 00245 00246 /** reads all LTC2942 register values prints on serial terminal 00247 * 00248 * @param none 00249 */ 00250 void readAll(); 00251 00252 /** reads the status register first 00253 * uses the ARA alert response protocol for SMBus to clear AL/CC pin 00254 * 00255 * int resp = ic.alertResponse(); 00256 * if((resp & TEMPERATURE_ALERT) == TEMPERATURE_ALERT) { } 00257 * 00258 * @return STATUS register 00259 */ 00260 int alertResponse(); 00261 00262 private: 00263 char getStatusReg(); 00264 char getControlReg(); 00265 void setControlReg(char); 00266 00267 I2C _i2c; 00268 InterruptIn _alcc; 00269 00270 char _addr; 00271 float _battCap; 00272 float _battMax; 00273 float _presc; 00274 }; 00275 00276 00277 #endif
Generated on Sat Jul 16 2022 08:04:36 by
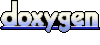