
There are many examples on MBED for ST7735 controlled LCDs, but they're mostly for the AdaFruit LCD. This is a working example of the library used for the cheap-as-dirt KMR-1.8 SPI 128x160 TFT LCD, on a "Black Pill" STM32F407VE developement board.
Dependencies: 24_TFT_STMNUCLEO mbed
main.cpp
00001 // WORKS with KMR-1.8 128x160 TFT LCD 00002 // 00003 // Hookup: 00004 // VCC, LED+ - 3.3V 00005 // CS - PC_4 00006 // SCLK - PA_5 00007 // SDA - PA_7 00008 // A0 - PC_5 00009 // RESET - PA_4 00010 00011 00012 #include "mbed.h" 00013 #include "st7735.h" 00014 #include "terminus.h" 00015 00016 // create the lcd instance 00017 // ST7735_LCD( PinName CS, PinName RESET, PinName RS, PinName SCL, PinName SDA, PinName BL = NC, backlight_t blType = Constant, float defaultBackLightLevel = 1.0 ); 00018 ST7735_LCD lcd( PC_4, PA_4, PC_5, PA_5, PA_7 ); // control pins 00019 00020 int main() 00021 { 00022 // initialize display - place it in standard portrait mode and set background to black and 00023 lcd.Initialize( LANDSCAPE_REV, RGB16 ); 00024 lcd.ClearScreen(); 00025 // various graphics primitives 00026 lcd.DrawPixel(1,1,0xFF9FFF); 00027 lcd.DrawLine(10,10,150,10,0xFF29A9); 00028 lcd.FillCircle(70,60,10,0x00FF00); 00029 lcd.DrawRect(30,80,50,100,0x0000FF); 00030 lcd.DrawRect(60,80,80,100,0xFF00FF); // any RGB mix XX-XX-XX 00031 lcd.DrawRect(90,80,110,100,0x00FFFF); // any RGB mix XX-XX-XX 00032 lcd.DrawRect(120,80,140,100,0xFFFF00); // any RGB mix XX-XX-XX 00033 lcd.SetFont( &TerminusFont ); 00034 // print something on the screen 00035 lcd.Print( "Hello, World!", CENTER, 25 ); // align text to center horizontally and use starndard colors 00036 while ( 1 ) { } 00037 }
Generated on Thu Jul 14 2022 07:32:52 by
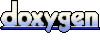