A library that maps the functions used with the mbed microcontroller to be used on with the GPIO ports of a Raspberry pi.
BusIn Class Reference
BusIn is used to create a number of DigitalIn pins that can be read as one value. More...
#include <BusIn.h>
Inherits CPPToPigpio::CPPToPigpio.
Public Member Functions | |
BusIn (PinName p0, PinName p1=NC, PinName p2=NC, PinName p3=NC, PinName p4=NC, PinName p5=NC, PinName p6=NC, PinName p7=NC, PinName p8=NC, PinName p9=NC, PinName p10=NC, PinName p11=NC, PinName p12=NC, PinName p13=NC, PinName p14=NC, PinName p15=NC) | |
Creates a BusIn, connected to the specific pins. | |
BusIn (int p0, int p1=-1, int p2=-1, int p3=-1, int p4=-1, int p5=-1, int p6=-1, int p7=-1, int p8=-1, int p9=-1, int p10=-1, int p11=-1, int p12=-1, int p13=-1, int p14=-1, int p15=-1) | |
Creates a BusIn, connected to the specific pins. | |
BusIn (int busSize, PinName pinNames[16]) | |
Creates a BusIn, connected to the specific pins. | |
BusIn (int busSize, int pinNames[16]) | |
Creates a BusIn, connected to the specific pins. | |
int | read () |
Read value of input pins. | |
void | mode (PinMode pull) |
Sets the input pin mode for all pins in the bus. | |
operator int () | |
A shorthand for read() | |
DigitalIn & | operator[] (int index) |
Access to particular bit in random-iterator fashion. | |
long long | mask () |
Binary mask of bus pins connected to actual pins (not NC pins) If bus pin is in NC state make corresponding bit will be cleared (set to 0), else bit will be set to 1. | |
~BusIn () | |
Desconstructor for object. |
Detailed Description
BusIn is used to create a number of DigitalIn pins that can be read as one value.
Definition at line 9 of file BusIn.h.
Constructor & Destructor Documentation
BusIn | ( | PinName | p0, |
PinName | p1 = NC , |
||
PinName | p2 = NC , |
||
PinName | p3 = NC , |
||
PinName | p4 = NC , |
||
PinName | p5 = NC , |
||
PinName | p6 = NC , |
||
PinName | p7 = NC , |
||
PinName | p8 = NC , |
||
PinName | p9 = NC , |
||
PinName | p10 = NC , |
||
PinName | p11 = NC , |
||
PinName | p12 = NC , |
||
PinName | p13 = NC , |
||
PinName | p14 = NC , |
||
PinName | p15 = NC |
||
) |
Creates a BusIn, connected to the specific pins.
- Parameters:
-
p0 DigitalIn pin to connect to bus bit p1 DigitalIn pin to connect to bus bit p2 DigitalIn pin to connect to bus bit p4 DigitalIn pin to connect to bus bit p5 DigitalIn pin to connect to bus bit p6 DigitalIn pin to connect to bus bit p7 DigitalIn pin to connect to bus bit p8 DigitalIn pin to connect to bus bit p9 DigitalIn pin to connect to bus bit p10 DigitalIn pin to connect to bus bit p11 DigitalIn pin to connect to bus bit p12 DigitalIn pin to connect to bus bit p13 DigitalIn pin to connect to bus bit p14 DigitalIn pin to connect to bus bit p15 DigitalIn pin to connect to bus bit
BusIn | ( | int | p0, |
int | p1 = -1 , |
||
int | p2 = -1 , |
||
int | p3 = -1 , |
||
int | p4 = -1 , |
||
int | p5 = -1 , |
||
int | p6 = -1 , |
||
int | p7 = -1 , |
||
int | p8 = -1 , |
||
int | p9 = -1 , |
||
int | p10 = -1 , |
||
int | p11 = -1 , |
||
int | p12 = -1 , |
||
int | p13 = -1 , |
||
int | p14 = -1 , |
||
int | p15 = -1 |
||
) |
Creates a BusIn, connected to the specific pins.
- Parameters:
-
p0 DigitalIn pin to connect to bus bit p1 DigitalIn pin to connect to bus bit p2 DigitalIn pin to connect to bus bit p4 DigitalIn pin to connect to bus bit p5 DigitalIn pin to connect to bus bit p6 DigitalIn pin to connect to bus bit p7 DigitalIn pin to connect to bus bit p8 DigitalIn pin to connect to bus bit p9 DigitalIn pin to connect to bus bit p10 DigitalIn pin to connect to bus bit p11 DigitalIn pin to connect to bus bit p12 DigitalIn pin to connect to bus bit p13 DigitalIn pin to connect to bus bit p14 DigitalIn pin to connect to bus bit p15 DigitalIn pin to connect to bus bit
BusIn | ( | int | busSize, |
PinName | pinNames[16] | ||
) |
BusIn | ( | int | busSize, |
int | pinNames[16] | ||
) |
Member Function Documentation
long long mask | ( | ) |
void mode | ( | PinMode | pull ) |
operator int | ( | ) |
DigitalIn & operator[] | ( | int | index ) |
Generated on Tue Jul 12 2022 14:39:23 by
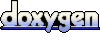