We connected an OLED to I2C of weather:bit.
Dependencies: BME280 SSD1308_128x64_I2C microbit
Fork of mbed_oled by
main.cpp
00001 /* 00002 * micro:bit weather:bit + SSD1306 OLED 128x64 OLED Test program 00003 * 00004 * Copyright (c) 2017 Kazuhiro Ouchi (@kanpapa) 00005 * Released under the MIT License: http://mbed.org/license/mit 00006 * 00007 * version 0.1 Initial Release 00008 */ 00009 #include "MicroBit.h" 00010 #include "mbed_logo.h" 00011 #include "SSD1308.h" // https://os.mbed.com/users/wim/code/SSD1308_128x64_I2C/ 00012 #include "BME280.h" // https://os.mbed.com/users/MACRUM/code/BME280/ 00013 00014 MicroBit uBit; 00015 MicroBitI2C i2c(I2C_SDA0, I2C_SCL0); 00016 00017 // Instantiate OLED SSD1306 00018 // I2C address 0x78 00019 SSD1308 oled = SSD1308(i2c, SSD1308_SA0); 00020 00021 // I2C address 0x76(default) 00022 // Instantiate BME280 sensor 00023 BME280 sensor = BME280(i2c); 00024 00025 int main() { 00026 // Initialise the micro:bit runtime. 00027 uBit.init(); 00028 00029 // micro:bit LED test 00030 uBit.display.scroll("HELLO WORLD! :)"); 00031 00032 // OLED test 00033 oled.writeString(0, 0, "Hello World !"); 00034 00035 oled.fillDisplay(0xAA); 00036 oled.setDisplayOff(); 00037 wait(1); 00038 oled.setDisplayOn(); 00039 00040 // weather info display 00041 while (1) { 00042 oled.clearDisplay(); 00043 oled.setDisplayInverse(); 00044 wait(0.5); 00045 oled.setDisplayNormal(); 00046 00047 oled.writeBitmap((uint8_t*) mbed_logo); 00048 wait(1); 00049 00050 oled.clearDisplay(); 00051 oled.printf("%2.2f degC, %04.2f hPa, %2.2f %%\n", sensor.getTemperature(), sensor.getPressure(), sensor.getHumidity()); 00052 wait(10); 00053 } 00054 } //main
Generated on Sun Jul 17 2022 11:36:27 by
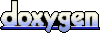