
WS2812 example
Dependencies: WS2812_LED mbed PixelArray
main.cpp
00001 #include "mbed.h" 00002 #include "WS2812.h" 00003 00004 LowPowerTicker toggleTicker; 00005 00006 Color color_red = {255, 0, 0}; 00007 Color color_green = {0, 255, 0}; 00008 Color color_blue = {0, 0, 255}; 00009 Color color_black = {0,0,0}; 00010 00011 bool update_led_flag; 00012 bool ledstrip_busy; 00013 int state = 0; 00014 00015 void update(void) { 00016 update_led_flag = true; 00017 } 00018 00019 void send_complete(int event) { 00020 (void)event; 00021 ledstrip_busy = false; 00022 } 00023 00024 event_callback_t callback; 00025 00026 int main(void) { 00027 00028 SPI spi(PE10, NC, PE12); 00029 00030 callback.attach(send_complete); 00031 00032 WS2812 ledstrip(spi, callback); 00033 00034 toggleTicker.attach(&update, 1.0f); 00035 00036 while(1) { 00037 sleep(); 00038 if(update_led_flag) 00039 { 00040 update_led_flag=false; 00041 switch(state){ 00042 case 0: 00043 ledstrip.set_all(&color_green); 00044 state++; 00045 break; 00046 case 1: 00047 ledstrip.set_all(&color_blue); 00048 state++; 00049 break; 00050 case 2: 00051 ledstrip.set_all(&color_red); 00052 state=0; 00053 break; 00054 } 00055 ledstrip_busy = true; 00056 ledstrip.update_strip(); 00057 } 00058 } 00059 }
Generated on Thu Jul 14 2022 04:25:17 by
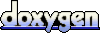