Debounce InterruptIn
Dependents: led_sigfox Allumag_lampe_sigfox Case_study_02_Turnstile B18_MP3_PLAYER ... more
DebouncedInterrupt.h
00001 00002 #ifndef DEBOUNCED_INTERRUPT_H 00003 #define DEBOUNCED_INTERRUPT_H 00004 00005 #include <stdint.h> 00006 #include "mbed.h" 00007 #include "FunctionPointer.h" 00008 00009 /** 00010 typedef enum { 00011 IRQ_NONE, 00012 IRQ_RISE, 00013 IRQ_FALL 00014 } gpio_irq_event; 00015 **/ 00016 00017 /** A replacement for InterruptIn that debounces the interrupt 00018 * 00019 * Example: 00020 * @code 00021 * 00022 * #include "DebouncedInterrupt.h" 00023 * 00024 * DebouncedInterrupt up_button(p15); 00025 * 00026 * void onUp() 00027 * { 00028 * // Do Something 00029 * } 00030 * 00031 * int main() 00032 * { 00033 * up_button.attach(&onUp,IRQ_FALL, 100); 00034 * while(1) { 00035 * ... 00036 * } 00037 * } 00038 * @endcode 00039 */ 00040 class DebouncedInterrupt { 00041 private: 00042 unsigned int _debounce_us; 00043 InterruptIn *_in; 00044 DigitalIn *_din; 00045 gpio_irq_event _trigger; 00046 00047 // Diagnostics 00048 volatile unsigned int _bounce_count; 00049 volatile unsigned int _last_bounce_count; 00050 00051 void _onInterrupt(void); 00052 void _callback(void); 00053 public: 00054 DebouncedInterrupt(PinName pin); 00055 ~DebouncedInterrupt(); 00056 00057 // Start monitoring the interupt and attach a callback 00058 void attach(void (*fptr)(void), const gpio_irq_event trigger, const uint32_t& debounce_ms=10); 00059 00060 template<typename T> 00061 void attach(T* tptr, void (T::*mptr)(void), const gpio_irq_event trigger, const uint32_t& debounce_ms=10) { 00062 _fAttach.attach(tptr, mptr); 00063 _last_bounce_count = _bounce_count = 0; 00064 _debounce_us = 1000*debounce_ms; 00065 _trigger = trigger; 00066 00067 switch(trigger) 00068 { 00069 case IRQ_RISE: 00070 _in->rise(tptr, &DebouncedInterrupt::_onInterrupt); 00071 break; 00072 case IRQ_FALL: 00073 _in->fall(tptr, &DebouncedInterrupt::_onInterrupt); 00074 break; 00075 case IRQ_NONE: 00076 reset(); // Unexpected. Clear callbacks. 00077 break; 00078 } 00079 } 00080 00081 // Stop monitoring the interrupt 00082 void reset(); 00083 00084 00085 /* 00086 * Get number of bounces 00087 * @return: bounce count 00088 */ 00089 unsigned int get_bounce(); 00090 protected: 00091 // https://github.com/mbedmicro/mbed/blob/master/libraries/mbed/api/FunctionPointer.h 00092 FunctionPointer _fAttach; 00093 }; 00094 #endif
Generated on Fri Jul 15 2022 02:31:04 by
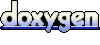