Debounce InterruptIn
Dependents: led_sigfox Allumag_lampe_sigfox Case_study_02_Turnstile B18_MP3_PLAYER ... more
DebouncedInterrupt.cpp
00001 /** 00002 * DebouncedInterrupt.cpp 00003 **/ 00004 #include "DebouncedInterrupt.h" 00005 00006 Timeout timeout; 00007 00008 DebouncedInterrupt::DebouncedInterrupt(PinName pin) 00009 { 00010 _in = new InterruptIn(pin); 00011 _din = new DigitalIn(pin); 00012 } 00013 00014 DebouncedInterrupt::~DebouncedInterrupt() 00015 { 00016 delete _in; 00017 delete _din; 00018 } 00019 00020 void DebouncedInterrupt::attach(void (*fptr)(void), const gpio_irq_event trigger, const unsigned int& debounce_ms) 00021 { 00022 if(fptr) { 00023 _fAttach.attach(fptr); 00024 _last_bounce_count = _bounce_count = 0; 00025 _debounce_us = 1000*debounce_ms; 00026 _trigger = trigger; 00027 00028 switch(trigger) 00029 { 00030 case IRQ_RISE: 00031 _in->rise(this, &DebouncedInterrupt::_onInterrupt); 00032 break; 00033 case IRQ_FALL: 00034 _in->fall(this, &DebouncedInterrupt::_onInterrupt); 00035 break; 00036 case IRQ_NONE: 00037 reset(); // Unexpected. Clear callbacks. 00038 break; 00039 } 00040 } else { 00041 reset(); 00042 } 00043 } 00044 00045 void DebouncedInterrupt::reset() 00046 { 00047 timeout.detach(); 00048 } 00049 00050 unsigned int DebouncedInterrupt::get_bounce() 00051 { 00052 return _last_bounce_count; 00053 } 00054 00055 void DebouncedInterrupt::_callback() 00056 { 00057 _last_bounce_count = _bounce_count; 00058 _bounce_count = 0; 00059 if(_din->read() == (_trigger==IRQ_RISE)) { 00060 _fAttach.call(); 00061 } 00062 } 00063 00064 void DebouncedInterrupt::_onInterrupt() 00065 { 00066 _bounce_count++; 00067 timeout.attach_us(this, &DebouncedInterrupt::_callback, _debounce_us); 00068 }
Generated on Fri Jul 15 2022 02:31:04 by
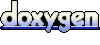