This is library for using WizFi250
Dependents: WebSocket_WizFi250_HelloWorld IFTTT_WizFi250 AxedaGo-WizFi250 FANARM_AP_udp_server ... more
WizFi250_sock.cpp
00001 /* 00002 /* Copyright (C) 2013 gsfan, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 /* Copyright (C) 2014 Wiznet, MIT License 00020 * port to the Wiznet Module WizFi250 00021 */ 00022 00023 #include "WizFi250.h" 00024 00025 00026 int WizFi250::getHostByName(const char * host, char *ip) 00027 { 00028 int i, flg = 0; 00029 00030 if(!isAssociated() || _state.status != STAT_READY) return -1; 00031 00032 for(i=0; i<strlen(host); i++) 00033 { 00034 if( (host[i] < '0' || host[i] > '9') && host[i] != '.') 00035 { 00036 flg = 1; 00037 break; 00038 } 00039 } 00040 if (!flg) 00041 { 00042 strncpy(ip, host, 16); 00043 return 0; 00044 } 00045 00046 if ( cmdFDNS(host) ) 00047 { 00048 wait_ms(1000); 00049 if( cmdFDNS(host) ) return -1; 00050 } 00051 strncpy(ip, _state.resolv, 16); 00052 return 0; 00053 } 00054 00055 int WizFi250::open(Protocol proto, const char *ip, int remotePort, int localPort, void(*func)(int)) 00056 { 00057 int cid; 00058 00059 if (!isAssociated() || _state.status != STAT_READY) return -1; 00060 00061 _state.cid = -1; 00062 00063 if (proto == PROTO_TCP) 00064 { 00065 if( cmdSCON( "O","TCN",ip, remotePort, localPort, "0" ) ) return -1; 00066 } 00067 else if(proto == PROTO_UDP) 00068 { 00069 if( cmdSCON( "O","UCN",ip, remotePort, localPort, "0" ) ) return -1; 00070 } 00071 if(_state.cid < 0) return -1; 00072 00073 initCon(_state.cid, true); 00074 cid = _state.cid; 00075 _con[cid].protocol = proto; 00076 _con[cid].type = TYPE_CLIENT; 00077 _con[cid].func = func; 00078 return cid; 00079 } 00080 00081 int WizFi250::listen (Protocol proto, int port, void(*func)(int)) 00082 { 00083 int cid; 00084 00085 if(!isAssociated() || _state.status != STAT_READY) return -1; 00086 00087 _state.cid = -1; 00088 00089 if(proto == PROTO_TCP) 00090 { 00091 if( sendCommand("AT+MEVTMSG=1") ) return -1; 00092 if( cmdSCON("O","TSN",port) ) return -1; 00093 } 00094 else 00095 { 00096 if( cmdSCON("O","USN",port) ) return -1; 00097 } 00098 00099 if (_state.cid < 0) return -1; 00100 cid = _state.cid; 00101 _con[cid].protocol = proto; 00102 _con[cid].type = TYPE_SERVER; 00103 _con[cid].func = func; 00104 00105 return cid; 00106 } 00107 00108 int WizFi250::close (int cid) 00109 { 00110 // if(!isConnected(cid)) return -1; 00111 00112 _con[cid].connected = false; 00113 return cmdCLOSE(cid); 00114 } 00115 00116 00117 void WizFi250::initCon ( int cid, bool connected ) 00118 { 00119 _con[cid].parent = -1; // It will be delete because It is not need 00120 _con[cid].func = NULL; 00121 _con[cid].accept = false; 00122 00123 //#ifndef CFG_ENABLE_RTOS 00124 if ( _con[cid].buf == NULL ) 00125 { 00126 _con[cid].buf = new CircBuffer<char>(CFG_DATA_SIZE); 00127 if ( _con[cid].buf == NULL ) error("Can't allocate memory"); 00128 } 00129 //#endif 00130 if ( _con[cid].buf != NULL ) 00131 { 00132 _con[cid].buf->flush(); 00133 } 00134 _con[cid].connected = connected; 00135 } 00136 00137 int WizFi250::send(int cid, const char *buf, int len) 00138 { 00139 if(!isConnected(cid)) return -1; 00140 00141 if((_con[cid].protocol == PROTO_TCP) || 00142 (_con[cid].protocol == PROTO_UDP && _con[cid].type == TYPE_CLIENT) ) 00143 { 00144 // if ( len > CFG_DATA_SIZE) len = CFG_DATA_SIZE; 00145 return cmdSSEND(buf,cid,len); 00146 } 00147 else 00148 { 00149 return -1; 00150 } 00151 } 00152 00153 int WizFi250::sendto (int cid, const char *buf, int len, const char *ip, int port) 00154 { 00155 if(!isConnected(cid)) return -1; 00156 00157 if((_con[cid].protocol == PROTO_UDP && _con[cid].type == TYPE_SERVER)) 00158 { 00159 if ( len > CFG_DATA_SIZE ) len = CFG_DATA_SIZE; 00160 return cmdSSEND(buf,cid,len,ip,port); 00161 } 00162 else 00163 { 00164 return -1; 00165 } 00166 } 00167 00168 int WizFi250::recv (int cid, char *buf, int len) 00169 { 00170 int i; 00171 00172 if (!isConnected(cid)) return -1; 00173 00174 if (_con[cid].buf == NULL ) return 0; 00175 while (!_con[cid].received && _state.mode != MODE_COMMAND); 00176 _con[cid].received = false; 00177 for(i=0; i<len; i++) 00178 { 00179 if(_con[cid].buf->dequeue(&buf[i]) == false) break; 00180 } 00181 setRts(true); // release 00182 return i; 00183 } 00184 00185 int WizFi250::recvfrom (int cid, char *buf, int len, char *ip, int *port) 00186 { 00187 int i; 00188 00189 if (!isConnected(cid)) return -1; 00190 00191 if (_con[cid].buf == NULL) return 0; 00192 00193 while (!_con[cid].received && _state.mode != MODE_COMMAND); 00194 00195 _con[cid].received = false; 00196 for(i=0; i<len; i++) 00197 { 00198 if( _con[cid].buf->dequeue(&buf[i]) == false ) break; 00199 } 00200 //buf[i] = '\0'; 00201 strncpy(ip, _con[cid].ip, 16); 00202 *port = _con[cid].port; 00203 setRts(true); // release 00204 00205 return i; 00206 } 00207 00208 int WizFi250::readable (int cid) 00209 { 00210 if (!isConnected(cid)) return -1; 00211 00212 if(_con[cid].buf == NULL) return -1; 00213 return _con[cid].buf->available(); 00214 } 00215 00216 bool WizFi250::isConnected (int cid) 00217 { 00218 if ( cid < 0 || cid >=8 ) return false; 00219 00220 return _con[cid].connected; 00221 } 00222 00223 int WizFi250::accept (int cid) 00224 { 00225 if(!isConnected(cid)) return -1; 00226 00227 if(_con[cid].connected && _con[cid].accept) 00228 { 00229 _con[cid].accept = false; 00230 return cid; 00231 } 00232 00233 return -1; 00234 } 00235 00236 int WizFi250::getRemote(int cid, char **ip, int *port) 00237 { 00238 if (!isConnected(cid)) return -1; 00239 00240 *ip = _con[cid].ip; 00241 *port = _con[cid].port; 00242 return 0; 00243 }
Generated on Fri Jul 15 2022 19:39:25 by
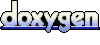