This is library for using WizFi250
Dependents: WebSocket_WizFi250_HelloWorld IFTTT_WizFi250 AxedaGo-WizFi250 FANARM_AP_udp_server ... more
WizFi250_hal.cpp
00001 /* 00002 * Copyright (C) 2013 gsfan, MIT License 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00005 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00006 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00007 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00008 * furnished to do so, subject to the following conditions: 00009 * 00010 * The above copyright notice and this permission notice shall be included in all copies or 00011 * substantial portions of the Software. 00012 * 00013 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00014 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00015 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00016 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00017 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00018 */ 00019 /* Copyright (C) 2014 Wiznet, MIT License 00020 * port to the Wiznet Module WizFi250 00021 */ 00022 00023 #include "WizFi250.h" 00024 00025 void WizFi250::setReset(bool flg) 00026 { 00027 if( flg ) 00028 { 00029 // low 00030 _reset.output(); 00031 _reset = 0; 00032 } 00033 else 00034 { 00035 // high z 00036 _reset.input(); 00037 _reset.mode(PullNone); 00038 } 00039 } 00040 00041 void WizFi250::isrUart() 00042 { 00043 char c; 00044 00045 c = getUart(); 00046 00047 recvData(c); 00048 //S_UartPutc(c); 00049 } 00050 00051 int WizFi250::getUart() 00052 { 00053 return _wizfi.getc(); 00054 } 00055 00056 void WizFi250::putUart (char c) 00057 { 00058 _wizfi.putc(c); 00059 } 00060 00061 void WizFi250::setRts (bool flg) 00062 { 00063 if (flg) 00064 { 00065 if(_flow == 2) 00066 { 00067 if(_rts) 00068 { 00069 _rts->write(0); // low 00070 } 00071 } 00072 } 00073 else 00074 { 00075 if(_flow == 2) 00076 { 00077 if(_rts) 00078 { 00079 _rts->write(1); // high 00080 } 00081 } 00082 } 00083 } 00084 00085 int WizFi250::lockUart (int ms) 00086 { 00087 Timer t; 00088 00089 if(_state.mode != MODE_COMMAND) 00090 { 00091 t.start(); 00092 while(_state.mode != MODE_COMMAND) 00093 { 00094 if(t.read_ms() >= ms) 00095 { 00096 WIZ_WARN("lock timeout (%d)\r\n", _state.mode); 00097 return -1; 00098 } 00099 } 00100 } 00101 00102 #ifdef CFG_ENABLE_RTOS 00103 if (_mutexUart.lock(ms) != osOK) return -1; 00104 #endif 00105 00106 if(_flow == 2) 00107 { 00108 if(_cts && _cts->read()) 00109 { 00110 // CTS check 00111 t.start(); 00112 while (_cts->read()) 00113 { 00114 if(t.read_ms() >= ms) 00115 { 00116 WIZ_DBG("cts timeout\r\n"); 00117 return -1; 00118 } 00119 } 00120 } 00121 } 00122 00123 setRts(false); // blcok 00124 return 0; 00125 } 00126 00127 void WizFi250::unlockUart() 00128 { 00129 setRts(true); // release 00130 #ifdef CFG_ENABLE_RTOS 00131 _mutexUart.unlock(); 00132 #endif 00133 } 00134 00135 void WizFi250::initUart (PinName cts, PinName rts, PinName alarm, int baud) 00136 { 00137 _baud = baud; 00138 if (_baud) _wizfi.baud(_baud); 00139 00140 _wizfi.attach(this, &WizFi250::isrUart, Serial::RxIrq); 00141 00142 _cts = NULL; 00143 _rts = NULL; 00144 _flow = 0; 00145 00146 if(cts != NC) 00147 { 00148 _cts = new DigitalIn(cts); 00149 } 00150 if(rts != NC) 00151 { 00152 _rts = new DigitalOut(rts); 00153 _flow = 2; 00154 } 00155 }
Generated on Fri Jul 15 2022 19:39:25 by
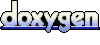