Simple read/write API for the W25X40BV SPI Flash IC wiki: http://mbed.org/cookbook/W25X40BV
Embed:
(wiki syntax)
Show/hide line numbers
W25X40BV.h
00001 // W25X40BV.h 00002 00003 #ifndef W25X40BV_H 00004 #define W25X40BV_H 00005 00006 #include "mbed.h" 00007 00008 #define SPI_FREQ 1000000 00009 #define SPI_MODE 0 00010 #define SPI_NBIT 8 00011 00012 #define WE_INST 0x06 00013 #define WD_INST 0x04 00014 #define R_INST 0x03 00015 #define W_INST 0x02 00016 #define C_ERASE_INST 0x60 00017 00018 #define DUMMY_ADDR 0x00 00019 #define WAIT_TIME 1 00020 00021 #define ADDR_BMASK2 0x00ff0000 00022 #define ADDR_BMASK1 0x0000ff00 00023 #define ADDR_BMASK0 0x000000ff 00024 00025 #define ADDR_BSHIFT2 16 00026 #define ADDR_BSHIFT1 8 00027 #define ADDR_BSHIFT0 0 00028 00029 class W25X40BV: public SPI { 00030 public: 00031 W25X40BV(PinName mosi, PinName miso, PinName sclk, PinName cs); 00032 00033 int readByte(int addr); // takes a 24-bit (3 bytes) address and returns the data (1 byte) at that location 00034 int readByte(int a2, int a1, int a0); // takes the address in 3 separate bytes A[23,16], A[15,8], A[7,0] 00035 void readStream(int addr, char* buf, int count); // takes a 24-bit address, reads count bytes, and stores results in buf 00036 00037 void writeByte(int addr, int data); // takes a 24-bit (3 bytes) address and a byte of data to write at that location 00038 void writeByte(int a2, int a1, int a0, int data); // takes the address in 3 separate bytes A[23,16], A[15,8], A[7,0] 00039 void writeStream(int addr, char* buf, int count); // write count bytes of data from buf to memory, starting at addr 00040 00041 void chipErase(); // erase all data on chip 00042 00043 private: 00044 void writeEnable(); // write enable 00045 void writeDisable(); // write disable 00046 void chipEnable(); // chip enable 00047 void chipDisable(); // chip disable 00048 00049 // SPI _spi; 00050 DigitalOut _cs; 00051 }; 00052 00053 #endif
Generated on Wed Jul 13 2022 00:28:16 by
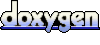