simple MCP4XXX digital potentiometer library
Embed:
(wiki syntax)
Show/hide line numbers
MCP4XXX.cpp
00001 #include "MCP4XXX.h" 00002 00003 MCP4XXX::MCP4XXX(PinName miso, PinName mosi, PinName clk, PinName cs) { 00004 _cs = new DigitalOut(cs); 00005 _spi = new SPI(miso, mosi, clk); 00006 _spi->format(8,0); 00007 _spi->frequency(1000000); 00008 _lvl = 0x00; 00009 } 00010 00011 MCP4XXX::~MCP4XXX() 00012 { 00013 delete _cs; 00014 delete _spi; 00015 } 00016 00017 void MCP4XXX::setLevel(unsigned char lvl, bool isP0) { 00018 *_cs = 0; 00019 if (isP0) 00020 _spi->write(P0_ADDR); 00021 else 00022 _spi->write(P1_ADDR); 00023 _spi->write(lvl); 00024 *_cs = 1; 00025 _lvl = lvl; 00026 } 00027 00028 unsigned char MCP4XXX::getLevel() { 00029 return _lvl; 00030 }
Generated on Thu Jul 21 2022 11:44:55 by
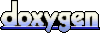