
FRDM-K64F, Avnet M14A2A, Grove Shield, to create smart home system. In use with AT&Ts M2x & Flow.
Dependencies: mbed FXOS8700CQ MODSERIAL
main.cpp
00001 /* =================================================================== 00002 Copyright © 2016, AVNET Inc. 00003 00004 Licensed under the Apache License, Version 2.0 (the "License"); 00005 you may not use this file except in compliance with the License. 00006 You may obtain a copy of the License at 00007 00008 http://www.apache.org/licenses/LICENSE-2.0 00009 00010 Unless required by applicable law or agreed to in writing, 00011 software distributed under the License is distributed on an 00012 "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, 00013 either express or implied. See the License for the specific 00014 language governing permissions and limitations under the License. 00015 00016 ======================================================================== */ 00017 00018 #include "mbed.h" 00019 #include <cctype> 00020 #include <string> 00021 #include "config_me.h" 00022 #include "sensors.h" 00023 #include "cell_modem.h" 00024 #include "hardware.h" 00025 00026 #include "DHT.h" 00027 float current_temp = 0.0f, rel_humid = 0.0f; 00028 00029 I2C i2c(PTC11, PTC10); //SDA, SCL -- define the I2C pins being used 00030 MODSERIAL pc(USBTX, USBRX, 256, 256); // tx, rx with default tx, rx buffer sizes 00031 MODSERIAL mdm(PTD3, PTD2, 4096, 4096); 00032 DigitalOut led_green(LED_GREEN); 00033 DigitalOut led_red(LED_RED); 00034 DigitalOut led_blue(LED_BLUE); 00035 00036 00037 //******************************************************************************************************************************************** 00038 //* Create string with sensor readings that can be sent to flow as an HTTP get 00039 //******************************************************************************************************************************************** 00040 K64F_Sensors_t SENSOR_DATA = 00041 { 00042 .Temperature = "0", 00043 .Humidity = "0", 00044 .AccelX = "0", 00045 .AccelY = "0", 00046 .AccelZ = "0", 00047 .MagnetometerX = "0", 00048 .MagnetometerY = "0", 00049 .MagnetometerZ = "0", 00050 .AmbientLightVis = "0", 00051 .AmbientLightIr = "0", 00052 .UVindex = "0", 00053 .Proximity = "0", 00054 .Temperature_Si7020 = "0", 00055 .Humidity_Si7020 = "0", 00056 .Virtual_Sensor1 = "0", 00057 .Virtual_Sensor2 = "0", 00058 .Virtual_Sensor3 = "0", 00059 .Virtual_Sensor4 = "0", 00060 .Virtual_Sensor5 = "0", 00061 .Virtual_Sensor6 = "0", 00062 .Virtual_Sensor7 = "0", 00063 .Virtual_Sensor8 = "0", 00064 .GPS_Satellites = "0", 00065 .GPS_Latitude = "0", 00066 .GPS_Longitude = "0", 00067 .GPS_Altitude = "0", 00068 .GPS_Speed = "0", 00069 .GPS_Course = "0" 00070 }; 00071 00072 void display_app_firmware_version(void) 00073 { 00074 PUTS("\r\n\r\nApp Firmware: Release 1.0 - built: "__DATE__" "__TIME__"\r\n\r\n"); 00075 } 00076 00077 void GenerateModemString(char * modem_string) 00078 { 00079 switch(iSensorsToReport) 00080 { 00081 case TEMP_HUMIDITY_ONLY: 00082 { 00083 sprintf(modem_string, "GET %s%s?serial=%s&temp=%s&humidity=%s %s%s\r\n\r\n", FLOW_BASE_URL, FLOW_INPUT_NAME, FLOW_DEVICE_NAME, SENSOR_DATA.Temperature, SENSOR_DATA.Humidity, FLOW_URL_TYPE, MY_SERVER_URL); 00084 break; 00085 } 00086 case TEMP_HUMIDITY_ACCELEROMETER: 00087 { 00088 sprintf(modem_string, "GET %s%s?serial=%s&temp=%s&humidity=%s&accelX=%s&accelY=%s&accelZ=%s&alarm=%s %s%s\r\n\r\n", FLOW_BASE_URL, FLOW_INPUT_NAME, FLOW_DEVICE_NAME, SENSOR_DATA.Temperature, SENSOR_DATA.Humidity, SENSOR_DATA.AccelX,SENSOR_DATA.AccelY,SENSOR_DATA.AccelZ,SENSOR_DATA.Proximity, FLOW_URL_TYPE, MY_SERVER_URL); 00089 break; 00090 } 00091 case TEMP_HUMIDITY_ACCELEROMETER_GPS: 00092 { 00093 sprintf(modem_string, "GET %s%s?serial=%s&temp=%s&humidity=%s&accelX=%s&accelY=%s&accelZ=%s&gps_satellites=%s&latitude=%s&longitude=%s&altitude=%s&speed=%s&course=%s %s%s\r\n\r\n", FLOW_BASE_URL, FLOW_INPUT_NAME, FLOW_DEVICE_NAME, SENSOR_DATA.Temperature, SENSOR_DATA.Humidity, SENSOR_DATA.AccelX,SENSOR_DATA.AccelY,SENSOR_DATA.AccelZ,SENSOR_DATA.GPS_Satellites,SENSOR_DATA.GPS_Latitude,SENSOR_DATA.GPS_Longitude,SENSOR_DATA.GPS_Altitude,SENSOR_DATA.GPS_Speed,SENSOR_DATA.GPS_Course, FLOW_URL_TYPE, MY_SERVER_URL); 00094 break; 00095 } 00096 case TEMP_HUMIDITY_ACCELEROMETER_PMODSENSORS: 00097 { 00098 sprintf(modem_string, "GET %s%s?serial=%s&temp=%s&humidity=%s&accelX=%s&accelY=%s&accelZ=%s&proximity=%s&light_uv=%s&light_vis=%s&light_ir=%s %s%s\r\n\r\n", FLOW_BASE_URL, FLOW_INPUT_NAME, FLOW_DEVICE_NAME, SENSOR_DATA.Temperature, SENSOR_DATA.Humidity, SENSOR_DATA.AccelX,SENSOR_DATA.AccelY,SENSOR_DATA.AccelZ, SENSOR_DATA.Proximity, SENSOR_DATA.UVindex, SENSOR_DATA.AmbientLightVis, SENSOR_DATA.AmbientLightIr, FLOW_URL_TYPE, MY_SERVER_URL); 00099 break; 00100 } 00101 case TEMP_HUMIDITY_ACCELEROMETER_PMODSENSORS_VIRTUALSENSORS: 00102 { 00103 sprintf(modem_string, "GET %s%s?serial=%s&temp=%s&humidity=%s&accelX=%s&accelY=%s&accelZ=%s&proximity=%s&light_uv=%s&light_vis=%s&light_ir=%s&virt_sens1=%s&virt_sens2=%s&virt_sens3=%s&virt_sens4=%s&virt_sens5=%s&virt_sens6=%s&virt_sens7=%s&virt_sens8=%s %s%s\r\n\r\n", FLOW_BASE_URL, FLOW_INPUT_NAME, FLOW_DEVICE_NAME, SENSOR_DATA.Temperature, SENSOR_DATA.Humidity, SENSOR_DATA.AccelX,SENSOR_DATA.AccelY,SENSOR_DATA.AccelZ, SENSOR_DATA.Proximity, SENSOR_DATA.UVindex, SENSOR_DATA.AmbientLightVis, SENSOR_DATA.AmbientLightIr, SENSOR_DATA.Virtual_Sensor1, SENSOR_DATA.Virtual_Sensor2, SENSOR_DATA.Virtual_Sensor3, SENSOR_DATA.Virtual_Sensor4, SENSOR_DATA.Virtual_Sensor5, SENSOR_DATA.Virtual_Sensor6, SENSOR_DATA.Virtual_Sensor7, SENSOR_DATA.Virtual_Sensor8, FLOW_URL_TYPE, MY_SERVER_URL); 00104 break; 00105 } 00106 default: 00107 { 00108 sprintf(modem_string, "Invalid sensor selected\r\n\r\n"); 00109 break; 00110 } 00111 } //switch(iSensorsToReport) 00112 } //GenerateModemString 00113 00114 00115 //Periodic timer 00116 Ticker OneMsTicker; 00117 volatile bool bTimerExpiredFlag = false; 00118 int OneMsTicks = 0; 00119 int iTimer1Interval_ms = 1000; 00120 //******************************************************************************************************************************************** 00121 //* Periodic 1ms timer tick 00122 //******************************************************************************************************************************************** 00123 void OneMsFunction() 00124 { 00125 OneMsTicks++; 00126 if ((OneMsTicks % iTimer1Interval_ms) == 0) 00127 { 00128 bTimerExpiredFlag = true; 00129 } 00130 } //OneMsFunction() 00131 00132 //******************************************************************************************************************************************** 00133 //* Set the RGB LED's Color 00134 //* LED Color 0=Off to 7=White. 3 bits represent BGR (bit0=Red, bit1=Green, bit2=Blue) 00135 //******************************************************************************************************************************************** 00136 void SetLedColor(unsigned char ucColor) 00137 { 00138 //Note that when an LED is on, you write a 0 to it: 00139 led_red = !(ucColor & 0x1); //bit 0 00140 led_green = !(ucColor & 0x2); //bit 1 00141 led_blue = !(ucColor & 0x4); //bit 2 00142 } //SetLedColor() 00143 00144 //******************************************************************************************************************************************** 00145 //* Process the JSON response. In this example we are only extracting a LED color. 00146 //******************************************************************************************************************************************** 00147 bool parse_JSON(char* json_string) 00148 { 00149 char* beginquote; 00150 char token[] = "\"LED\":\""; 00151 beginquote = strstr(json_string, token ); 00152 if ((beginquote != 0)) 00153 { 00154 char cLedColor = beginquote[strlen(token)]; 00155 PRINTF(GRN "LED Found : %c" DEF "\r\n", cLedColor); 00156 switch(cLedColor) 00157 { 00158 case 'O': 00159 { //Off 00160 SetLedColor(0); 00161 break; 00162 } 00163 case 'R': 00164 { //Red 00165 SetLedColor(1); 00166 break; 00167 } 00168 case 'G': 00169 { //Green 00170 SetLedColor(2); 00171 break; 00172 } 00173 case 'Y': 00174 { //Yellow 00175 SetLedColor(3); 00176 break; 00177 } 00178 case 'B': 00179 { //Blue 00180 SetLedColor(4); 00181 break; 00182 } 00183 case 'M': 00184 { //Magenta 00185 SetLedColor(5); 00186 break; 00187 } 00188 case 'T': 00189 { //Turquoise 00190 SetLedColor(6); 00191 break; 00192 } 00193 case 'W': 00194 { //White 00195 SetLedColor(7); 00196 break; 00197 } 00198 default: 00199 { 00200 break; 00201 } 00202 } //switch(cLedColor) 00203 return true; 00204 } 00205 else 00206 { 00207 return false; 00208 } 00209 } //parse_JSON 00210 00211 //DigitalIn btn_temp_up(D4); 00212 //DigitalIn btn_temp_down(D8); 00213 DigitalIn btn_silence(D4); 00214 DigitalIn motion(D3); 00215 DigitalOut buzzer(D5); 00216 00217 AnalogIn pot_temp_set(A2); 00218 AnalogIn Alarm_Off(A3); 00219 //DHT sensor(D4, DHT11); 00220 00221 int main() { 00222 static unsigned ledOnce = 0; 00223 int error = 0; 00224 float h = 0.0f, c = 0.0f, f = 0.0f, k = 0.0f, dp = 0.0f, dpf = 0.0f; 00225 float value = 0.0f; 00226 float alm_off; 00227 float temp_setting = 0.0f, diff = 0.0f; 00228 int mycolor = 0x0; 00229 bool alarms = false; 00230 int mycounter = 0; 00231 int movement; 00232 00233 //delay so that the debug terminal can open after power-on reset: 00234 wait (5.0); 00235 pc.baud(115200); 00236 00237 display_app_firmware_version(); 00238 00239 PRINTF(GRN "Hello World from the Cellular IoT Kit!\r\n\r\n"); 00240 00241 //Initialize the I2C sensors that are present 00242 sensors_init(); 00243 read_sensors(); 00244 00245 // Set LED to RED until init finishes 00246 SetLedColor(0x1); //Red 00247 // Initialize the modem 00248 PRINTF("\r\n"); 00249 cell_modem_init(); 00250 display_wnc_firmware_rev(); 00251 00252 // Set LED BLUE for partial init 00253 SetLedColor(0x4); //Blue 00254 00255 //Create a 1ms timer tick function: 00256 iTimer1Interval_ms = SENSOR_UPDATE_INTERVAL_MS; 00257 OneMsTicker.attach(OneMsFunction, 0.001f) ; 00258 00259 // Send and receive data perpetually 00260 00261 mycolor = 0x0; 00262 SetLedColor(mycolor); 00263 00264 buzzer = 0; 00265 00266 while(1) { 00267 #ifdef USE_VIRTUAL_SENSORS 00268 ProcessUsbInterface(); 00269 #endif 00270 if (bTimerExpiredFlag) 00271 { 00272 bTimerExpiredFlag = false; 00273 read_sensors(); //read available external sensors from a PMOD and the on-board motion sensor 00274 char modem_string[512]; 00275 GenerateModemString(&modem_string[0]); 00276 char myJsonResponse[512]; 00277 if (cell_modem_Sendreceive(&modem_string[0], &myJsonResponse[0])) 00278 { 00279 if (!ledOnce) 00280 { 00281 ledOnce = 1; 00282 SetLedColor(0x2); //Green 00283 } 00284 //parse_JSON(&myJsonResponse[0]); 00285 } 00286 } //bTimerExpiredFlag 00287 //------------------------------------------------------------------------------ 00288 //------------------------------------------------------------------------------ 00289 //------------------------------------------------------------------------------ 00290 //-------------------------------BEGIN NEW CODE--------------------------------- 00291 //------------------------------------------------------------------------------ 00292 //------------------------------------------------------------------------------ 00293 /* error = sensor.readData(); 00294 if (0 == error) 00295 { 00296 c = sensor.ReadTemperature(CELCIUS); 00297 f = sensor.ReadTemperature(FARENHEIT); 00298 k = sensor.ReadTemperature(KELVIN); 00299 h = sensor.ReadHumidity(); 00300 dp = sensor.CalcdewPoint(c, h); 00301 dpf = sensor.CalcdewPointFast(c, h); 00302 //printf("Temperature in Kelvin: %4.2f, Celcius: %4.2f, Farenheit %4.2f\n", k, c, f); 00303 //printf("Humidity is %4.2f, Dewpoint: %4.2f, Dewpoint fast: %4.2f\n", h, dp, dpf); 00304 } 00305 else 00306 { 00307 //printf("Error: %d\n", error); 00308 } 00309 */ 00310 value = pot_temp_set; 00311 //printf("Slide location %3.6f\r\n", value); 00312 temp_setting = value * 40.0f + 50.0f; 00313 // printf(WHT "Thermostat is set to %3.2f degrees.\r\n", temp_setting); 00314 00315 //current_temp and rel_humid are assigned in sensors.cpp 00316 // printf(WHT "Current temperature is %3.2f degrees.\r\n", current_temp); 00317 // printf(WHT "Relative humidity is %3.2f percent.\r\n", rel_humid); 00318 00319 printf(WHT "\r\n"); 00320 00321 diff = current_temp - temp_setting; 00322 if ((diff > -2.0f && diff < 2.0f && rel_humid < 40.0f) && mycolor != 0x0) //if temperature is near optimal, and it is not humid, turn off heat and AC. 00323 { 00324 mycolor = 0x0; 00325 SetLedColor(mycolor); 00326 } 00327 if ((diff > -2.0f && diff < 2.0f && rel_humid > 40.0f) && mycolor != 0x2) //if temperature is near optimal, and it is humid, use fan only. 00328 { 00329 mycolor = 0x2; 00330 SetLedColor(mycolor); 00331 } 00332 if (diff > 4.0f && mycolor != 0x4) //if it is too hot, turn on AC. 00333 { 00334 mycolor = 0x4; 00335 SetLedColor(mycolor); 00336 } 00337 if (diff < -4.0f && mycolor != 0x1) //if it is too cold, turn on heat. 00338 { 00339 mycolor = 0x1; 00340 SetLedColor(mycolor); 00341 } 00342 00343 //if motion sensor detects motion, sound an alarm 00344 00345 if (motion && !alarms) 00346 { 00347 alarms = true; 00348 strcpy(SENSOR_DATA.Proximity, "1"); 00349 printf(WHT "--------------------------------\r\n"); 00350 printf(WHT "Alert! Home intrusion detected!\r\n"); 00351 printf(WHT "--------------------------------\r\n"); 00352 printf(WHT "\r\n"); 00353 } 00354 alm_off = Alarm_Off;//Switching to integer because AnalogIn acts weird in If statement 00355 if((alm_off > 0.5f) && alarms) 00356 { 00357 alarms = false; 00358 strcpy(SENSOR_DATA.Proximity, "0"); 00359 buzzer = 0; 00360 printf(WHT "Alarm disengaged. Resuming normal operation.\r\n"); 00361 printf(WHT "\r\n"); 00362 } 00363 if (alarms) 00364 { 00365 buzzer = !buzzer; 00366 } 00367 00368 00369 /*wait(1.0); 00370 00371 mycounter = mycounter + 1; 00372 if (mycounter >= 20) 00373 { 00374 mycounter = 0; 00375 } 00376 if (mycounter >= 15) 00377 { 00378 strcpy(SENSOR_DATA.Proximity, "1"); 00379 } 00380 else 00381 { 00382 strcpy(SENSOR_DATA.Proximity, "0"); 00383 } */ 00384 00385 } //forever loop 00386 }
Generated on Wed Jul 13 2022 15:25:27 by
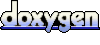