
FRDM-K64F, Avnet M14A2A, Grove Shield, to create smart home system. In use with AT&Ts M2x & Flow.
Dependencies: mbed FXOS8700CQ MODSERIAL
itm_output.cpp
00001 /* =================================================================== 00002 Copyright © 2016, AVNET Inc. 00003 00004 Licensed under the Apache License, Version 2.0 (the "License"); 00005 you may not use this file except in compliance with the License. 00006 You may obtain a copy of the License at 00007 00008 http://www.apache.org/licenses/LICENSE-2.0 00009 00010 Unless required by applicable law or agreed to in writing, 00011 software distributed under the License is distributed on an 00012 "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, 00013 either express or implied. See the License for the specific 00014 language governing permissions and limitations under the License. 00015 00016 ======================================================================== */ 00017 00018 //Used for ULINK output only 00019 // 00020 00021 /* ITM registers */ 00022 #define ITM_PORT0_U8 (*((volatile unsigned int *)0xE0000000)) 00023 #define ITM_PORT0_U32 (*((volatile unsigned long *)0xE0000000)) 00024 #define ITM_TER (*((volatile unsigned long *)0xE0000E00)) 00025 #define ITM_TCR (*((volatile unsigned long *)0xE0000E80)) 00026 00027 #define ITM_TCR_ITMENA_Msk (1UL << 0) 00028 00029 /*!< Value identifying \ref ITM_RxBuffer is ready for next character. */ 00030 #define ITM_RXBUFFER_EMPTY 0x5AA55AA5 00031 00032 /*!< Variable to receive characters. */ 00033 extern 00034 volatile int ITM_RxBuffer; 00035 volatile int ITM_RxBuffer = ITM_RXBUFFER_EMPTY; 00036 00037 /** \brief ITM Send Character 00038 00039 The function transmits a character via the ITM channel 0, and 00040 \li Just returns when no debugger is connected that has booked the output. 00041 \li Is blocking when a debugger is connected, but the previous character 00042 sent has not been transmitted. 00043 00044 \param [in] ch Character to transmit. 00045 00046 \returns Character to transmit. 00047 */ 00048 int ITM_putc (int ch) { 00049 if ((ITM_TCR & ITM_TCR_ITMENA_Msk) && /* ITM enabled */ 00050 (ITM_TER & (1UL << 0) )) { /* ITM Port #0 enabled */ 00051 while (ITM_PORT0_U32 == 0); 00052 ITM_PORT0_U8 = (int)ch; 00053 } 00054 return (ch); 00055 } 00056 00057 /** \brief ITM Receive Character 00058 00059 The function inputs a character via the external variable \ref ITM_RxBuffer. 00060 This variable is monitored and altered by the debugger to provide input. 00061 00062 \return Received character. 00063 \return -1 No character pending. 00064 */ 00065 int ITM_getc (void) { 00066 int ch = -1; /* no character available */ 00067 00068 if (ITM_RxBuffer != ITM_RXBUFFER_EMPTY) { 00069 ch = ITM_RxBuffer; 00070 ITM_RxBuffer = ITM_RXBUFFER_EMPTY; /* ready for next character */ 00071 } 00072 00073 return (ch); 00074 } 00075 00076 /** \brief ITM send string 00077 00078 The function sends a null terminated string via the external variable \ref ITM_RxBuffer. 00079 This variable is monitored and altered by the debugger to provide input. 00080 00081 \return Received character. 00082 \return -1 No character pending. 00083 */ 00084 int ITM_puts (char * str) { 00085 int i=0; 00086 00087 while(str[i]) 00088 ITM_putc(str[i++]); 00089 return i; 00090 } 00091
Generated on Wed Jul 13 2022 15:25:27 by
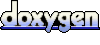