
FRDM-K64F, Avnet M14A2A, Grove Shield, to create smart home system. In use with AT&Ts M2x & Flow.
Dependencies: mbed FXOS8700CQ MODSERIAL
hts221_driver.cpp
00001 /* =================================================================== 00002 Copyright © 2016, AVNET Inc. 00003 00004 Licensed under the Apache License, Version 2.0 (the "License"); 00005 you may not use this file except in compliance with the License. 00006 You may obtain a copy of the License at 00007 00008 http://www.apache.org/licenses/LICENSE-2.0 00009 00010 Unless required by applicable law or agreed to in writing, 00011 software distributed under the License is distributed on an 00012 "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, 00013 either express or implied. See the License for the specific 00014 language governing permissions and limitations under the License. 00015 00016 ======================================================================== */ 00017 00018 #include "HTS221.h" 00019 00020 00021 // ------------------------------------------------------------------------------ 00022 //jmf -- define I2C pins and functions to read & write to I2C device 00023 00024 #include <string> 00025 #include "mbed.h" 00026 00027 #include "hardware.h" 00028 //I2C i2c(PTC11, PTC10); //SDA, SCL -- define the I2C pins being used. Defined in a 00029 //common locatioin since sensors also use I2C 00030 00031 // Read a single unsigned char from addressToRead and return it as a unsigned char 00032 unsigned char HTS221::readRegister(unsigned char slaveAddress, unsigned char ToRead) 00033 { 00034 char data = ToRead; 00035 00036 //i2c.write(slaveAddress, &data, 1, 0); 00037 i2c.write(slaveAddress, &data, 1, 1); //by Stefan 00038 i2c.read(slaveAddress, &data, 1, 0); 00039 return data; 00040 } 00041 00042 // Writes a single unsigned char (dataToWrite) into regToWrite 00043 int HTS221::writeRegister(unsigned char slaveAddress, unsigned char regToWrite, unsigned char dataToWrite) 00044 { 00045 const char data[] = {regToWrite, dataToWrite}; 00046 00047 return i2c.write(slaveAddress,data,2,0); 00048 } 00049 00050 00051 //jmf end 00052 // ------------------------------------------------------------------------------ 00053 00054 //static inline int humidityReady(uint8_t data) { 00055 // return (data & 0x02); 00056 //} 00057 //static inline int temperatureReady(uint8_t data) { 00058 // return (data & 0x01); 00059 //} 00060 00061 00062 HTS221::HTS221(void) : _address(HTS221_ADDRESS) 00063 { 00064 _temperature = 0; 00065 _humidity = 0; 00066 } 00067 00068 00069 int HTS221::begin(void) 00070 { 00071 uint8_t data; 00072 00073 data = readRegister(_address, WHO_AM_I); 00074 if (data == WHO_AM_I_RETURN){ 00075 if (activate()){ 00076 storeCalibration(); 00077 return data; 00078 } 00079 } 00080 00081 return 0; 00082 } 00083 00084 int 00085 HTS221::storeCalibration(void) 00086 { 00087 uint8_t data; 00088 uint16_t tmp; 00089 00090 for (int reg=CALIB_START; reg<=CALIB_END; reg++) { 00091 if ((reg!=CALIB_START+8) && (reg!=CALIB_START+9) && (reg!=CALIB_START+4)) { 00092 00093 data = readRegister(HTS221_ADDRESS, reg); 00094 00095 switch (reg) { 00096 case CALIB_START: 00097 _h0_rH = data; 00098 break; 00099 case CALIB_START+1: 00100 _h1_rH = data; 00101 break; 00102 case CALIB_START+2: 00103 _T0_degC = data; 00104 break; 00105 case CALIB_START+3: 00106 _T1_degC = data; 00107 break; 00108 00109 case CALIB_START+5: 00110 tmp = _T0_degC; 00111 _T0_degC = (data&0x3)<<8; 00112 _T0_degC |= tmp; 00113 00114 tmp = _T1_degC; 00115 _T1_degC = ((data&0xC)>>2)<<8; 00116 _T1_degC |= tmp; 00117 break; 00118 case CALIB_START+6: 00119 _H0_T0 = data; 00120 break; 00121 case CALIB_START+7: 00122 _H0_T0 |= data<<8; 00123 break; 00124 case CALIB_START+0xA: 00125 _H1_T0 = data; 00126 break; 00127 case CALIB_START+0xB: 00128 _H1_T0 |= data <<8; 00129 break; 00130 case CALIB_START+0xC: 00131 _T0_OUT = data; 00132 break; 00133 case CALIB_START+0xD: 00134 _T0_OUT |= data << 8; 00135 break; 00136 case CALIB_START+0xE: 00137 _T1_OUT = data; 00138 break; 00139 case CALIB_START+0xF: 00140 _T1_OUT |= data << 8; 00141 break; 00142 00143 00144 case CALIB_START+8: 00145 case CALIB_START+9: 00146 case CALIB_START+4: 00147 //DO NOTHING 00148 break; 00149 00150 // to cover any possible error 00151 default: 00152 return false; 00153 } /* switch */ 00154 } /* if */ 00155 } /* for */ 00156 return true; 00157 } 00158 00159 00160 int 00161 HTS221::activate(void) 00162 { 00163 uint8_t data; 00164 00165 data = readRegister(_address, CTRL_REG1); 00166 data |= POWER_UP; 00167 data |= ODR0_SET; 00168 writeRegister(_address, CTRL_REG1, data); 00169 00170 return true; 00171 } 00172 00173 00174 int HTS221::deactivate(void) 00175 { 00176 uint8_t data; 00177 00178 data = readRegister(_address, CTRL_REG1); 00179 data &= ~POWER_UP; 00180 writeRegister(_address, CTRL_REG1, data); 00181 return true; 00182 } 00183 00184 00185 int 00186 HTS221::bduActivate(void) 00187 { 00188 uint8_t data; 00189 00190 data = readRegister(_address, CTRL_REG1); 00191 data |= BDU_SET; 00192 writeRegister(_address, CTRL_REG1, data); 00193 00194 return true; 00195 } 00196 00197 00198 int 00199 HTS221::bduDeactivate(void) 00200 { 00201 uint8_t data; 00202 00203 data = readRegister(_address, CTRL_REG1); 00204 data &= ~BDU_SET; 00205 writeRegister(_address, CTRL_REG1, data); 00206 return true; 00207 } 00208 00209 00210 int 00211 HTS221::readHumidity(void) 00212 { 00213 uint8_t data = 0; 00214 uint16_t h_out = 0; 00215 double h_temp = 0.0; 00216 double hum = 0.0; 00217 00218 data = readRegister(_address, STATUS_REG); 00219 00220 if (data & HUMIDITY_READY) { 00221 data = readRegister(_address, HUMIDITY_H_REG); 00222 h_out = data << 8; // MSB 00223 data = readRegister(_address, HUMIDITY_L_REG); 00224 h_out |= data; // LSB 00225 00226 // Decode Humidity 00227 hum = ((int16_t)(_h1_rH) - (int16_t)(_h0_rH))/2.0; // remove x2 multiple 00228 00229 // Calculate humidity in decimal of grade centigrades i.e. 15.0 = 150. 00230 h_temp = (((int16_t)h_out - (int16_t)_H0_T0) * hum) / ((int16_t)_H1_T0 - (int16_t)_H0_T0); 00231 hum = ((int16_t)_h0_rH) / 2.0; // remove x2 multiple 00232 _humidity = (int16_t)((hum + h_temp)); // provide signed % measurement unit 00233 } 00234 return _humidity; 00235 } 00236 00237 00238 00239 double 00240 HTS221::readTemperature(void) 00241 { 00242 uint8_t data = 0; 00243 uint16_t t_out = 0; 00244 double t_temp = 0.0; 00245 double deg = 0.0; 00246 00247 data = readRegister(_address, STATUS_REG); 00248 00249 if (data & TEMPERATURE_READY) { 00250 00251 data= readRegister(_address, TEMP_H_REG); 00252 t_out = data << 8; // MSB 00253 data = readRegister(_address, TEMP_L_REG); 00254 t_out |= data; // LSB 00255 00256 // Decode Temperature 00257 deg = ((int16_t)(_T1_degC) - (int16_t)(_T0_degC))/8.0; // remove x8 multiple 00258 00259 // Calculate Temperature in decimal of grade centigrades i.e. 15.0 = 150. 00260 t_temp = (((int16_t)t_out - (int16_t)_T0_OUT) * deg) / ((int16_t)_T1_OUT - (int16_t)_T0_OUT); 00261 deg = ((int16_t)_T0_degC) / 8.0; // remove x8 multiple 00262 _temperature = deg + t_temp; // provide signed celsius measurement unit 00263 } 00264 00265 return _temperature; 00266 }
Generated on Wed Jul 13 2022 15:25:27 by
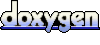