
lkvzxklzvxckl
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 DigitalOut internalLED(LED1); 00003 DigitalOut myled(p18); 00004 DigitalIn pb(p26); 00005 00006 // LEDs used to indicate code activity and reset source 00007 DigitalOut myled1(LED1); //in main loop part 1 00008 DigitalOut myled2(LED2); //in main loop part 2 (where fault occurs) 00009 DigitalOut myled3(LED3); //The pushbutton or power on caused a reset 00010 DigitalOut myled4(LED4); //The watchdog timer caused a reset 00011 // SPST Pushbutton demo using internal PullUp function 00012 // no external PullUp resistor needed 00013 // Pushbutton from P8 to GND. 00014 00015 class Watchdog { 00016 public: 00017 // Load timeout value in watchdog timer and enable 00018 void kick(float s) { 00019 LPC_WDT->WDCLKSEL = 0x1; // Set CLK src to PCLK 00020 uint32_t clk = SystemCoreClock / 16; // WD has a fixed /4 prescaler, PCLK default is /4 00021 LPC_WDT->WDTC = s * (float)clk; 00022 LPC_WDT->WDMOD = 0x3; // Enabled and Reset 00023 kick(); 00024 } 00025 // "kick" or "feed" the dog - reset the watchdog timer 00026 // by writing this required bit pattern 00027 void kick() { 00028 LPC_WDT->WDFEED = 0xAA; 00029 LPC_WDT->WDFEED = 0x55; 00030 } 00031 }; 00032 00033 // Setup the watchdog timer 00034 Watchdog wdt; 00035 00036 int main() { 00037 pb.mode(PullUp); 00038 int count = 0; 00039 // On reset, indicate a watchdog reset or a pushbutton reset on LED 4 or 3 00040 if ((LPC_WDT->WDMOD >> 2) & 1) 00041 myled4 = 1; else myled3 = 1; 00042 00043 // setup a 10 second timeout on watchdog timer hardware 00044 // needs to be longer than worst case main loop exection time 00045 wdt.kick(10.0); 00046 00047 // Main program loop - resets watchdog once each loop iteration 00048 // Would typically have a lot of code in loop with many calls 00049 while (1) { 00050 myled1 = 1; //Flash LEDs 1 & 2 to indicate normal loop activity 00051 wait(.05); 00052 myled1 = 0; 00053 myled2 = 1; 00054 wait(.05); 00055 // Simulate a fault lock up with an infinite while loop, but only after 25 loop iterations 00056 if (count == 25) while (1) {}; 00057 // LED 2 will stay on during the fault 00058 myled2 = 0; 00059 count ++; 00060 // End of main loop so "kick" to reset watchdog timer and avoid a reset 00061 wdt.kick(); 00062 00063 myled = !pb; 00064 internalLED = pb; 00065 } 00066 } 00067
Generated on Sun Dec 4 2022 23:59:05 by
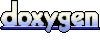