tone function Library
Dependents: PiezoBuzzer_HelloWorld_WIZwiki-W7500 kragl kragl_v2 Internet_Orgel ... more
pwm_tone.cpp
00001 /* Includes ------------------------------------------------------------------*/ 00002 #include "pwm_tone.h" 00003 00004 /* Private typedef -----------------------------------------------------------*/ 00005 /* Private define ------------------------------------------------------------*/ 00006 /* Private variables ---------------------------------------------------------*/ 00007 /* Private function prototypes -----------------------------------------------*/ 00008 /* Private functions ---------------------------------------------------------*/ 00009 00010 /** 00011 * @brief Tune Function 00012 * @param name : Choose the PwmOut 00013 period : this param is tune value. (C_3...B_5) 00014 beat : this param is beat value. (1..16) 1 means 1/16 beat 00015 * @retval None 00016 */ 00017 void Tune(PwmOut name, int period, int beat) 00018 { 00019 int delay; 00020 00021 delay = beat*63; 00022 name.period_us(period); 00023 name.write(0.50f); // 50% duty cycle 00024 wait_ms(delay); // 1 beat 00025 name.period_us(0); // Sound off 00026 } 00027 00028 /** 00029 * @brief Auto tunes Function 00030 * @param name : Choose the PwmOut 00031 period : this param is tune value. (C_3...B_5) 00032 beat : this param is beat value. (1..16) 1 means 1/16 beat 00033 * @retval None 00034 */ 00035 void Auto_tunes(PwmOut name, int period, int beat) 00036 { 00037 int delay; 00038 00039 delay = beat*63; 00040 name.period_us(period); 00041 name.write(0.50f); // 50% duty cycle 00042 wait_ms(delay); 00043 } 00044 00045 /** 00046 * @brief Stop tunes Function 00047 * @param name : Choose the PwmOut 00048 * @retval None 00049 */ 00050 void Stop_tunes(PwmOut name) 00051 { 00052 name.period_us(0); 00053 }
Generated on Tue Jul 12 2022 16:33:01 by
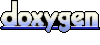