Adafruit 9DOF - LSM303DLHC
Dependents: Galileo_HoverBoardRider accelerometerTest
LSM303DLHC.h
00001 #ifndef __LSM303DLHC_H 00002 #define __LSM303DLHC_H 00003 00004 #include "mbed.h" 00005 00006 // register addresses 00007 00008 #define CTRL_REG1_A 0x20 00009 #define CTRL_REG2_A 0x21 00010 #define CTRL_REG3_A 0x22 00011 #define CTRL_REG4_A 0x23 00012 #define CTRL_REG5_A 0x24 00013 #define CTRL_REG6_A 0x25 // DLHC only 00014 #define REFERENCE_A 0x26 00015 #define STATUS_REG_A 0x27 00016 00017 #define OUT_X_L_A 0x28 00018 #define OUT_X_H_A 0x29 00019 #define OUT_Y_L_A 0x2A 00020 #define OUT_Y_H_A 0x2B 00021 #define OUT_Z_L_A 0x2C 00022 #define OUT_Z_H_A 0x2D 00023 00024 #define INT1_CFG_A 0x30 00025 #define INT1_SRC_A 0x31 00026 #define INT1_THS_A 0x32 00027 #define INT1_DURATION_A 0x33 00028 #define INT2_CFG_A 0x34 00029 #define INT2_SRC_A 0x35 00030 #define INT2_THS_A 0x36 00031 #define INT2_DURATION_A 0x37 00032 00033 #define CRA_REG_M 0x00 00034 #define CRB_REG_M 0x01 00035 #define MR_REG_M 0x02 00036 00037 #define OUT_X_H_M 0x03 00038 #define OUT_X_L_M 0x04 00039 #define OUT_Y_H_M 0x07 00040 #define OUT_Y_L_M 0x08 00041 #define OUT_Z_H_M 0x05 00042 #define OUT_Z_L_M 0x06 00043 00044 #define SR_REG_M 0x09 00045 #define IRA_REG_M 0x0A 00046 #define IRB_REG_M 0x0B 00047 #define IRC_REG_M 0x0C 00048 00049 /** Tilt-compensated compass interface Library for the STMicro LSM303DLm 3-axis magnetometer, 3-axis acceleromter 00050 * @author Michael Shimniok http://www.bot-thoughts.com/ 00051 * 00052 * This is an early revision; I've not yet implemented heading calculation and the interface differs from my 00053 * earlier LSM303DLH; I hope to make this library drop in compatible at some point in the future. 00054 * setScale() and setOffset() have no effect at this time. 00055 * 00056 * @code 00057 * #include "mbed.h" 00058 * #include "LSM303DLM.h" 00059 * 00060 * LSM303DLM compass(p28, p27); 00061 * ... 00062 * int a[3], m[3]; 00063 * ... 00064 * compass.readAcc(a); 00065 * compass.readMag(m); 00066 * 00067 * @endcode 00068 */ 00069 class LSM303DLHC { 00070 00071 public: 00072 /** Create a new interface for an LSM303DLM 00073 * 00074 * @param sda is the pin for the I2C SDA line 00075 * @param scl is the pin for the I2C SCL line 00076 */ 00077 LSM303DLHC(PinName sda, PinName scl); 00078 00079 /** sets the x, y, and z offset corrections for hard iron calibration 00080 * 00081 * Calibration details here: 00082 * http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/ 00083 * 00084 * If you gather raw magnetometer data and find, for example, x is offset 00085 * by hard iron by -20 then pass +20 to this member function to correct 00086 * for hard iron. 00087 * 00088 * @param x is the offset correction for the x axis 00089 * @param y is the offset correction for the y axis 00090 * @param z is the offset correction for the z axis 00091 */ 00092 void init(); 00093 void setOffset(float x, float y, float z); 00094 00095 /** sets the scale factor for the x, y, and z axes 00096 * 00097 * Calibratio details here: 00098 * http://mbed.org/users/shimniok/notebook/quick-and-dirty-3d-compass-calibration/ 00099 * 00100 * Sensitivity of the three axes is never perfectly identical and this 00101 * function can help to correct differences in sensitivity. You're 00102 * supplying a multipler such that x, y and z will be normalized to the 00103 * same max/min values 00104 */ 00105 void setScale(float x, float y, float z); 00106 00107 /** read the calibrated accelerometer and magnetometer values 00108 * 00109 * @param a is the accelerometer 3d vector, written by the function 00110 * @param m is the magnetometer 3d vector, written by the function 00111 */ 00112 void read(int a[3], int m[3]); 00113 00114 /** read the calibrated accelerometer values 00115 * 00116 * @param a is the accelerometer 3d vector, written by the function 00117 */ 00118 void readAcc(int a[3]); 00119 00120 /** read the calibrated magnetometer values 00121 * 00122 * @param m is the magnetometer 3d vector, written by the function 00123 */ 00124 void readMag(int m[3]); 00125 00126 /** sets the I2C bus frequency 00127 * 00128 * @param frequency is the I2C bus/clock frequency, either standard (100000) or fast (400000) 00129 */ 00130 void frequency(int hz); 00131 00132 private: 00133 I2C _device; 00134 char _data[6]; 00135 int offset[3]; 00136 int scale[3]; 00137 }; 00138 00139 #endif
Generated on Fri Jul 15 2022 03:18:26 by
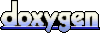